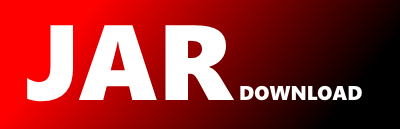
aws.sdk.kotlin.services.transcribe.model.StartTranscriptionJobRequest.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of transcribe Show documentation
Show all versions of transcribe Show documentation
Amazon Transcribe Service
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.transcribe.model
class StartTranscriptionJobRequest private constructor(builder: Builder) {
/**
* An object that contains the request parameters for content redaction.
*/
val contentRedaction: aws.sdk.kotlin.services.transcribe.model.ContentRedaction? = builder.contentRedaction
/**
* Set this field to true to enable automatic language identification. Automatic language identification
* is disabled by default. You receive a BadRequestException error if you enter a value for a
* LanguageCode.
*/
val identifyLanguage: kotlin.Boolean? = builder.identifyLanguage
/**
* Provides information about how a transcription job is executed. Use this field to indicate that the job can be
* queued for deferred execution if the concurrency limit is reached and there are no slots available to immediately run
* the job.
*/
val jobExecutionSettings: aws.sdk.kotlin.services.transcribe.model.JobExecutionSettings? = builder.jobExecutionSettings
/**
* A map of plain text, non-secret key:value pairs, known as encryption context pairs, that provide an added
* layer of security for your data.
*/
val kmsEncryptionContext: Map? = builder.kmsEncryptionContext
/**
* The language code for the language used in the input media file.
* To transcribe speech in Modern Standard Arabic (ar-SA), your audio or video file must be encoded at a sample
* rate of 16,000 Hz or higher.
*/
val languageCode: aws.sdk.kotlin.services.transcribe.model.LanguageCode? = builder.languageCode
/**
* The language identification settings associated with your transcription job. These settings include
* VocabularyName, VocabularyFilterName, and
* LanguageModelName.
*/
val languageIdSettings: Map? = builder.languageIdSettings
/**
* An object containing a list of languages that might be present in your collection of audio files. Automatic language
* identification chooses a language that best matches the source audio from that list.
* To transcribe speech in Modern Standard Arabic (ar-SA), your audio or video file must be encoded at a sample
* rate of 16,000 Hz or higher.
*/
val languageOptions: List? = builder.languageOptions
/**
* An object that describes the input media for a transcription job.
*/
val media: aws.sdk.kotlin.services.transcribe.model.Media? = builder.media
/**
* The format of the input media file.
*/
val mediaFormat: aws.sdk.kotlin.services.transcribe.model.MediaFormat? = builder.mediaFormat
/**
* The sample rate, in Hertz, of the audio track in the input media file.
* If you do not specify the media sample rate, Amazon Transcribe determines the sample rate. If you specify the sample rate, it
* must match the sample rate detected by Amazon Transcribe. In most cases, you should leave the
* MediaSampleRateHertz field blank and let Amazon Transcribe determine the sample rate.
*/
val mediaSampleRateHertz: kotlin.Int? = builder.mediaSampleRateHertz
/**
* Choose the custom language model you use for your transcription job in this parameter.
*/
val modelSettings: aws.sdk.kotlin.services.transcribe.model.ModelSettings? = builder.modelSettings
/**
* The location where the transcription is stored.
* If you set the OutputBucketName, Amazon Transcribe puts the transcript in the specified S3 bucket. When
* you call the GetTranscriptionJob operation, the operation returns this location in the
* TranscriptFileUri field. If you enable content redaction, the redacted transcript appears in
* RedactedTranscriptFileUri. If you enable content redaction and choose to output an unredacted
* transcript, that transcript's location still appears in the TranscriptFileUri. The S3 bucket must have
* permissions that allow Amazon Transcribe to put files in the bucket. For more information, see Permissions Required for
* IAM User Roles.
* You can specify an Amazon Web Services Key Management Service (KMS) key to encrypt the output of your
* transcription using the OutputEncryptionKMSKeyId parameter. If you don't specify a KMS key, Amazon Transcribe
* uses the default Amazon S3 key for server-side encryption of transcripts that are placed in your S3 bucket.
* If you don't set the OutputBucketName, Amazon Transcribe generates a pre-signed URL, a shareable URL that
* provides secure access to your transcription, and returns it in the TranscriptFileUri field. Use this URL
* to download the transcription.
*/
val outputBucketName: kotlin.String? = builder.outputBucketName
/**
* The Amazon Resource Name (ARN) of the Amazon Web Services Key Management Service (KMS) key used to
* encrypt the output of the transcription job. The user calling the StartTranscriptionJob
* operation must have permission to use the specified KMS key.
* You can use either of the following to identify a KMS key in the current account:
* KMS Key ID: "1234abcd-12ab-34cd-56ef-1234567890ab"
* KMS Key Alias: "alias/ExampleAlias"
* You can use either of the following to identify a KMS key in the current account or another account:
* Amazon Resource Name (ARN) of a KMS Key: "arn:aws:kms:region:account
* ID:key/1234abcd-12ab-34cd-56ef-1234567890ab"
* ARN of a KMS Key Alias: "arn:aws:kms:region:account-ID:alias/ExampleAlias"
* If you don't specify an encryption key, the output of the transcription job is encrypted with the default
* Amazon S3 key (SSE-S3).
* If you specify a KMS key to encrypt your output, you must also specify an output location in the
* OutputBucketName parameter.
*/
val outputEncryptionKmsKeyId: kotlin.String? = builder.outputEncryptionKmsKeyId
/**
* You can specify a location in an Amazon S3 bucket to store the output of your transcription job.
* If you don't specify an output key, Amazon Transcribe stores the output of your transcription job in the Amazon S3 bucket you
* specified. By default, the object key is "your-transcription-job-name.json".
* You can use output keys to specify the Amazon S3 prefix and file name of the transcription output. For example,
* specifying the Amazon S3 prefix, "folder1/folder2/", as an output key would lead to the output being stored as
* "folder1/folder2/your-transcription-job-name.json". If you specify "my-other-job-name.json" as the output key, the
* object key is changed to "my-other-job-name.json". You can use an output key to change both the prefix and the file
* name, for example "folder/my-other-job-name.json".
* If you specify an output key, you must also specify an S3 bucket in the OutputBucketName
* parameter.
*/
val outputKey: kotlin.String? = builder.outputKey
/**
* A Settings object that provides optional settings for a transcription job.
*/
val settings: aws.sdk.kotlin.services.transcribe.model.Settings? = builder.settings
/**
* Add subtitles to your batch transcription job.
*/
val subtitles: aws.sdk.kotlin.services.transcribe.model.Subtitles? = builder.subtitles
/**
* Add tags to an Amazon Transcribe transcription job.
*/
val tags: List? = builder.tags
/**
* The name of the job. You can't use the strings "." or ".." by themselves as the
* job name. The name must also be unique within an Amazon Web Services account. If you try to create a transcription
* job with the same name as a previous transcription job, you get a ConflictException error.
*/
val transcriptionJobName: kotlin.String? = builder.transcriptionJobName
companion object {
operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.transcribe.model.StartTranscriptionJobRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("StartTranscriptionJobRequest(")
append("contentRedaction=$contentRedaction,")
append("identifyLanguage=$identifyLanguage,")
append("jobExecutionSettings=$jobExecutionSettings,")
append("kmsEncryptionContext=$kmsEncryptionContext,")
append("languageCode=$languageCode,")
append("languageIdSettings=$languageIdSettings,")
append("languageOptions=$languageOptions,")
append("media=$media,")
append("mediaFormat=$mediaFormat,")
append("mediaSampleRateHertz=$mediaSampleRateHertz,")
append("modelSettings=$modelSettings,")
append("outputBucketName=$outputBucketName,")
append("outputEncryptionKmsKeyId=$outputEncryptionKmsKeyId,")
append("outputKey=$outputKey,")
append("settings=$settings,")
append("subtitles=$subtitles,")
append("tags=$tags,")
append("transcriptionJobName=$transcriptionJobName)")
}
override fun hashCode(): kotlin.Int {
var result = contentRedaction?.hashCode() ?: 0
result = 31 * result + (identifyLanguage?.hashCode() ?: 0)
result = 31 * result + (jobExecutionSettings?.hashCode() ?: 0)
result = 31 * result + (kmsEncryptionContext?.hashCode() ?: 0)
result = 31 * result + (languageCode?.hashCode() ?: 0)
result = 31 * result + (languageIdSettings?.hashCode() ?: 0)
result = 31 * result + (languageOptions?.hashCode() ?: 0)
result = 31 * result + (media?.hashCode() ?: 0)
result = 31 * result + (mediaFormat?.hashCode() ?: 0)
result = 31 * result + (mediaSampleRateHertz ?: 0)
result = 31 * result + (modelSettings?.hashCode() ?: 0)
result = 31 * result + (outputBucketName?.hashCode() ?: 0)
result = 31 * result + (outputEncryptionKmsKeyId?.hashCode() ?: 0)
result = 31 * result + (outputKey?.hashCode() ?: 0)
result = 31 * result + (settings?.hashCode() ?: 0)
result = 31 * result + (subtitles?.hashCode() ?: 0)
result = 31 * result + (tags?.hashCode() ?: 0)
result = 31 * result + (transcriptionJobName?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as StartTranscriptionJobRequest
if (contentRedaction != other.contentRedaction) return false
if (identifyLanguage != other.identifyLanguage) return false
if (jobExecutionSettings != other.jobExecutionSettings) return false
if (kmsEncryptionContext != other.kmsEncryptionContext) return false
if (languageCode != other.languageCode) return false
if (languageIdSettings != other.languageIdSettings) return false
if (languageOptions != other.languageOptions) return false
if (media != other.media) return false
if (mediaFormat != other.mediaFormat) return false
if (mediaSampleRateHertz != other.mediaSampleRateHertz) return false
if (modelSettings != other.modelSettings) return false
if (outputBucketName != other.outputBucketName) return false
if (outputEncryptionKmsKeyId != other.outputEncryptionKmsKeyId) return false
if (outputKey != other.outputKey) return false
if (settings != other.settings) return false
if (subtitles != other.subtitles) return false
if (tags != other.tags) return false
if (transcriptionJobName != other.transcriptionJobName) return false
return true
}
inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.transcribe.model.StartTranscriptionJobRequest = Builder(this).apply(block).build()
class Builder {
/**
* An object that contains the request parameters for content redaction.
*/
var contentRedaction: aws.sdk.kotlin.services.transcribe.model.ContentRedaction? = null
/**
* Set this field to true to enable automatic language identification. Automatic language identification
* is disabled by default. You receive a BadRequestException error if you enter a value for a
* LanguageCode.
*/
var identifyLanguage: kotlin.Boolean? = null
/**
* Provides information about how a transcription job is executed. Use this field to indicate that the job can be
* queued for deferred execution if the concurrency limit is reached and there are no slots available to immediately run
* the job.
*/
var jobExecutionSettings: aws.sdk.kotlin.services.transcribe.model.JobExecutionSettings? = null
/**
* A map of plain text, non-secret key:value pairs, known as encryption context pairs, that provide an added
* layer of security for your data.
*/
var kmsEncryptionContext: Map? = null
/**
* The language code for the language used in the input media file.
* To transcribe speech in Modern Standard Arabic (ar-SA), your audio or video file must be encoded at a sample
* rate of 16,000 Hz or higher.
*/
var languageCode: aws.sdk.kotlin.services.transcribe.model.LanguageCode? = null
/**
* The language identification settings associated with your transcription job. These settings include
* VocabularyName, VocabularyFilterName, and
* LanguageModelName.
*/
var languageIdSettings: Map? = null
/**
* An object containing a list of languages that might be present in your collection of audio files. Automatic language
* identification chooses a language that best matches the source audio from that list.
* To transcribe speech in Modern Standard Arabic (ar-SA), your audio or video file must be encoded at a sample
* rate of 16,000 Hz or higher.
*/
var languageOptions: List? = null
/**
* An object that describes the input media for a transcription job.
*/
var media: aws.sdk.kotlin.services.transcribe.model.Media? = null
/**
* The format of the input media file.
*/
var mediaFormat: aws.sdk.kotlin.services.transcribe.model.MediaFormat? = null
/**
* The sample rate, in Hertz, of the audio track in the input media file.
* If you do not specify the media sample rate, Amazon Transcribe determines the sample rate. If you specify the sample rate, it
* must match the sample rate detected by Amazon Transcribe. In most cases, you should leave the
* MediaSampleRateHertz field blank and let Amazon Transcribe determine the sample rate.
*/
var mediaSampleRateHertz: kotlin.Int? = null
/**
* Choose the custom language model you use for your transcription job in this parameter.
*/
var modelSettings: aws.sdk.kotlin.services.transcribe.model.ModelSettings? = null
/**
* The location where the transcription is stored.
* If you set the OutputBucketName, Amazon Transcribe puts the transcript in the specified S3 bucket. When
* you call the GetTranscriptionJob operation, the operation returns this location in the
* TranscriptFileUri field. If you enable content redaction, the redacted transcript appears in
* RedactedTranscriptFileUri. If you enable content redaction and choose to output an unredacted
* transcript, that transcript's location still appears in the TranscriptFileUri. The S3 bucket must have
* permissions that allow Amazon Transcribe to put files in the bucket. For more information, see Permissions Required for
* IAM User Roles.
* You can specify an Amazon Web Services Key Management Service (KMS) key to encrypt the output of your
* transcription using the OutputEncryptionKMSKeyId parameter. If you don't specify a KMS key, Amazon Transcribe
* uses the default Amazon S3 key for server-side encryption of transcripts that are placed in your S3 bucket.
* If you don't set the OutputBucketName, Amazon Transcribe generates a pre-signed URL, a shareable URL that
* provides secure access to your transcription, and returns it in the TranscriptFileUri field. Use this URL
* to download the transcription.
*/
var outputBucketName: kotlin.String? = null
/**
* The Amazon Resource Name (ARN) of the Amazon Web Services Key Management Service (KMS) key used to
* encrypt the output of the transcription job. The user calling the StartTranscriptionJob
* operation must have permission to use the specified KMS key.
* You can use either of the following to identify a KMS key in the current account:
* KMS Key ID: "1234abcd-12ab-34cd-56ef-1234567890ab"
* KMS Key Alias: "alias/ExampleAlias"
* You can use either of the following to identify a KMS key in the current account or another account:
* Amazon Resource Name (ARN) of a KMS Key: "arn:aws:kms:region:account
* ID:key/1234abcd-12ab-34cd-56ef-1234567890ab"
* ARN of a KMS Key Alias: "arn:aws:kms:region:account-ID:alias/ExampleAlias"
* If you don't specify an encryption key, the output of the transcription job is encrypted with the default
* Amazon S3 key (SSE-S3).
* If you specify a KMS key to encrypt your output, you must also specify an output location in the
* OutputBucketName parameter.
*/
var outputEncryptionKmsKeyId: kotlin.String? = null
/**
* You can specify a location in an Amazon S3 bucket to store the output of your transcription job.
* If you don't specify an output key, Amazon Transcribe stores the output of your transcription job in the Amazon S3 bucket you
* specified. By default, the object key is "your-transcription-job-name.json".
* You can use output keys to specify the Amazon S3 prefix and file name of the transcription output. For example,
* specifying the Amazon S3 prefix, "folder1/folder2/", as an output key would lead to the output being stored as
* "folder1/folder2/your-transcription-job-name.json". If you specify "my-other-job-name.json" as the output key, the
* object key is changed to "my-other-job-name.json". You can use an output key to change both the prefix and the file
* name, for example "folder/my-other-job-name.json".
* If you specify an output key, you must also specify an S3 bucket in the OutputBucketName
* parameter.
*/
var outputKey: kotlin.String? = null
/**
* A Settings object that provides optional settings for a transcription job.
*/
var settings: aws.sdk.kotlin.services.transcribe.model.Settings? = null
/**
* Add subtitles to your batch transcription job.
*/
var subtitles: aws.sdk.kotlin.services.transcribe.model.Subtitles? = null
/**
* Add tags to an Amazon Transcribe transcription job.
*/
var tags: List? = null
/**
* The name of the job. You can't use the strings "." or ".." by themselves as the
* job name. The name must also be unique within an Amazon Web Services account. If you try to create a transcription
* job with the same name as a previous transcription job, you get a ConflictException error.
*/
var transcriptionJobName: kotlin.String? = null
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.transcribe.model.StartTranscriptionJobRequest) : this() {
this.contentRedaction = x.contentRedaction
this.identifyLanguage = x.identifyLanguage
this.jobExecutionSettings = x.jobExecutionSettings
this.kmsEncryptionContext = x.kmsEncryptionContext
this.languageCode = x.languageCode
this.languageIdSettings = x.languageIdSettings
this.languageOptions = x.languageOptions
this.media = x.media
this.mediaFormat = x.mediaFormat
this.mediaSampleRateHertz = x.mediaSampleRateHertz
this.modelSettings = x.modelSettings
this.outputBucketName = x.outputBucketName
this.outputEncryptionKmsKeyId = x.outputEncryptionKmsKeyId
this.outputKey = x.outputKey
this.settings = x.settings
this.subtitles = x.subtitles
this.tags = x.tags
this.transcriptionJobName = x.transcriptionJobName
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.transcribe.model.StartTranscriptionJobRequest = StartTranscriptionJobRequest(this)
/**
* construct an [aws.sdk.kotlin.services.transcribe.model.ContentRedaction] inside the given [block]
*/
fun contentRedaction(block: aws.sdk.kotlin.services.transcribe.model.ContentRedaction.Builder.() -> kotlin.Unit) {
this.contentRedaction = aws.sdk.kotlin.services.transcribe.model.ContentRedaction.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.transcribe.model.JobExecutionSettings] inside the given [block]
*/
fun jobExecutionSettings(block: aws.sdk.kotlin.services.transcribe.model.JobExecutionSettings.Builder.() -> kotlin.Unit) {
this.jobExecutionSettings = aws.sdk.kotlin.services.transcribe.model.JobExecutionSettings.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.transcribe.model.Media] inside the given [block]
*/
fun media(block: aws.sdk.kotlin.services.transcribe.model.Media.Builder.() -> kotlin.Unit) {
this.media = aws.sdk.kotlin.services.transcribe.model.Media.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.transcribe.model.ModelSettings] inside the given [block]
*/
fun modelSettings(block: aws.sdk.kotlin.services.transcribe.model.ModelSettings.Builder.() -> kotlin.Unit) {
this.modelSettings = aws.sdk.kotlin.services.transcribe.model.ModelSettings.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.transcribe.model.Settings] inside the given [block]
*/
fun settings(block: aws.sdk.kotlin.services.transcribe.model.Settings.Builder.() -> kotlin.Unit) {
this.settings = aws.sdk.kotlin.services.transcribe.model.Settings.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.transcribe.model.Subtitles] inside the given [block]
*/
fun subtitles(block: aws.sdk.kotlin.services.transcribe.model.Subtitles.Builder.() -> kotlin.Unit) {
this.subtitles = aws.sdk.kotlin.services.transcribe.model.Subtitles.invoke(block)
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy