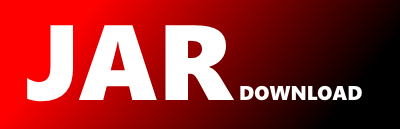
commonMain.aws.sdk.kotlin.services.transcribestreaming.model.Result.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of transcribestreaming-jvm Show documentation
Show all versions of transcribestreaming-jvm Show documentation
The AWS SDK for Kotlin client for Transcribe Streaming
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.transcribestreaming.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* The `Result` associated with a ``.
*
* Contains a set of transcription results from one or more audio segments, along with additional information per your request parameters. This can include information relating to alternative transcriptions, channel identification, partial result stabilization, language identification, and other transcription-related data.
*/
public class Result private constructor(builder: Builder) {
/**
* A list of possible alternative transcriptions for the input audio. Each alternative may contain one or more of `Items`, `Entities`, or `Transcript`.
*/
public val alternatives: List? = builder.alternatives
/**
* Indicates which audio channel is associated with the `Result`.
*/
public val channelId: kotlin.String? = builder.channelId
/**
* The end time, in milliseconds, of the `Result`.
*/
public val endTime: kotlin.Double = builder.endTime
/**
* Indicates if the segment is complete.
*
* If `IsPartial` is `true`, the segment is not complete. If `IsPartial` is `false`, the segment is complete.
*/
public val isPartial: kotlin.Boolean = builder.isPartial
/**
* The language code that represents the language spoken in your audio stream.
*/
public val languageCode: aws.sdk.kotlin.services.transcribestreaming.model.LanguageCode? = builder.languageCode
/**
* The language code of the dominant language identified in your stream.
*
* If you enabled channel identification and each channel of your audio contains a different language, you may have more than one result.
*/
public val languageIdentification: List? = builder.languageIdentification
/**
* Provides a unique identifier for the `Result`.
*/
public val resultId: kotlin.String? = builder.resultId
/**
* The start time, in milliseconds, of the `Result`.
*/
public val startTime: kotlin.Double = builder.startTime
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.transcribestreaming.model.Result = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("Result(")
append("alternatives=$alternatives,")
append("channelId=$channelId,")
append("endTime=$endTime,")
append("isPartial=$isPartial,")
append("languageCode=$languageCode,")
append("languageIdentification=$languageIdentification,")
append("resultId=$resultId,")
append("startTime=$startTime")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = alternatives?.hashCode() ?: 0
result = 31 * result + (channelId?.hashCode() ?: 0)
result = 31 * result + (endTime.hashCode())
result = 31 * result + (isPartial.hashCode())
result = 31 * result + (languageCode?.hashCode() ?: 0)
result = 31 * result + (languageIdentification?.hashCode() ?: 0)
result = 31 * result + (resultId?.hashCode() ?: 0)
result = 31 * result + (startTime.hashCode())
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as Result
if (alternatives != other.alternatives) return false
if (channelId != other.channelId) return false
if (!(endTime?.equals(other.endTime) ?: (other.endTime == null))) return false
if (isPartial != other.isPartial) return false
if (languageCode != other.languageCode) return false
if (languageIdentification != other.languageIdentification) return false
if (resultId != other.resultId) return false
if (!(startTime?.equals(other.startTime) ?: (other.startTime == null))) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.transcribestreaming.model.Result = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* A list of possible alternative transcriptions for the input audio. Each alternative may contain one or more of `Items`, `Entities`, or `Transcript`.
*/
public var alternatives: List? = null
/**
* Indicates which audio channel is associated with the `Result`.
*/
public var channelId: kotlin.String? = null
/**
* The end time, in milliseconds, of the `Result`.
*/
public var endTime: kotlin.Double = 0.0
/**
* Indicates if the segment is complete.
*
* If `IsPartial` is `true`, the segment is not complete. If `IsPartial` is `false`, the segment is complete.
*/
public var isPartial: kotlin.Boolean = false
/**
* The language code that represents the language spoken in your audio stream.
*/
public var languageCode: aws.sdk.kotlin.services.transcribestreaming.model.LanguageCode? = null
/**
* The language code of the dominant language identified in your stream.
*
* If you enabled channel identification and each channel of your audio contains a different language, you may have more than one result.
*/
public var languageIdentification: List? = null
/**
* Provides a unique identifier for the `Result`.
*/
public var resultId: kotlin.String? = null
/**
* The start time, in milliseconds, of the `Result`.
*/
public var startTime: kotlin.Double = 0.0
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.transcribestreaming.model.Result) : this() {
this.alternatives = x.alternatives
this.channelId = x.channelId
this.endTime = x.endTime
this.isPartial = x.isPartial
this.languageCode = x.languageCode
this.languageIdentification = x.languageIdentification
this.resultId = x.resultId
this.startTime = x.startTime
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.transcribestreaming.model.Result = Result(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy