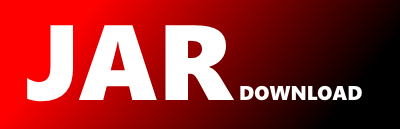
commonMain.aws.sdk.kotlin.services.transcribestreaming.model.UtteranceEvent.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of transcribestreaming-jvm Show documentation
Show all versions of transcribestreaming-jvm Show documentation
The AWS SDK for Kotlin client for Transcribe Streaming
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.transcribestreaming.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Contains set of transcription results from one or more audio segments, along with additional information about the parameters included in your request. For example, channel definitions, partial result stabilization, sentiment, and issue detection.
*/
public class UtteranceEvent private constructor(builder: Builder) {
/**
* The time, in milliseconds, from the beginning of the audio stream to the start of the `UtteranceEvent`.
*/
public val beginOffsetMillis: kotlin.Long? = builder.beginOffsetMillis
/**
* The time, in milliseconds, from the beginning of the audio stream to the start of the `UtteranceEvent`.
*/
public val endOffsetMillis: kotlin.Long? = builder.endOffsetMillis
/**
* Contains entities identified as personally identifiable information (PII) in your transcription output.
*/
public val entities: List? = builder.entities
/**
* Indicates whether the segment in the `UtteranceEvent` is complete (`FALSE`) or partial (`TRUE`).
*/
public val isPartial: kotlin.Boolean = builder.isPartial
/**
* Provides the issue that was detected in the specified segment.
*/
public val issuesDetected: List? = builder.issuesDetected
/**
* Contains words, phrases, or punctuation marks that are associated with the specified `UtteranceEvent`.
*/
public val items: List? = builder.items
/**
* Provides the role of the speaker for each audio channel, either `CUSTOMER` or `AGENT`.
*/
public val participantRole: aws.sdk.kotlin.services.transcribestreaming.model.ParticipantRole? = builder.participantRole
/**
* Provides the sentiment that was detected in the specified segment.
*/
public val sentiment: aws.sdk.kotlin.services.transcribestreaming.model.Sentiment? = builder.sentiment
/**
* Contains transcribed text.
*/
public val transcript: kotlin.String? = builder.transcript
/**
* The unique identifier that is associated with the specified `UtteranceEvent`.
*/
public val utteranceId: kotlin.String? = builder.utteranceId
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.transcribestreaming.model.UtteranceEvent = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("UtteranceEvent(")
append("beginOffsetMillis=$beginOffsetMillis,")
append("endOffsetMillis=$endOffsetMillis,")
append("entities=$entities,")
append("isPartial=$isPartial,")
append("issuesDetected=$issuesDetected,")
append("items=$items,")
append("participantRole=$participantRole,")
append("sentiment=$sentiment,")
append("transcript=$transcript,")
append("utteranceId=$utteranceId")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = beginOffsetMillis?.hashCode() ?: 0
result = 31 * result + (endOffsetMillis?.hashCode() ?: 0)
result = 31 * result + (entities?.hashCode() ?: 0)
result = 31 * result + (isPartial.hashCode())
result = 31 * result + (issuesDetected?.hashCode() ?: 0)
result = 31 * result + (items?.hashCode() ?: 0)
result = 31 * result + (participantRole?.hashCode() ?: 0)
result = 31 * result + (sentiment?.hashCode() ?: 0)
result = 31 * result + (transcript?.hashCode() ?: 0)
result = 31 * result + (utteranceId?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as UtteranceEvent
if (beginOffsetMillis != other.beginOffsetMillis) return false
if (endOffsetMillis != other.endOffsetMillis) return false
if (entities != other.entities) return false
if (isPartial != other.isPartial) return false
if (issuesDetected != other.issuesDetected) return false
if (items != other.items) return false
if (participantRole != other.participantRole) return false
if (sentiment != other.sentiment) return false
if (transcript != other.transcript) return false
if (utteranceId != other.utteranceId) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.transcribestreaming.model.UtteranceEvent = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The time, in milliseconds, from the beginning of the audio stream to the start of the `UtteranceEvent`.
*/
public var beginOffsetMillis: kotlin.Long? = null
/**
* The time, in milliseconds, from the beginning of the audio stream to the start of the `UtteranceEvent`.
*/
public var endOffsetMillis: kotlin.Long? = null
/**
* Contains entities identified as personally identifiable information (PII) in your transcription output.
*/
public var entities: List? = null
/**
* Indicates whether the segment in the `UtteranceEvent` is complete (`FALSE`) or partial (`TRUE`).
*/
public var isPartial: kotlin.Boolean = false
/**
* Provides the issue that was detected in the specified segment.
*/
public var issuesDetected: List? = null
/**
* Contains words, phrases, or punctuation marks that are associated with the specified `UtteranceEvent`.
*/
public var items: List? = null
/**
* Provides the role of the speaker for each audio channel, either `CUSTOMER` or `AGENT`.
*/
public var participantRole: aws.sdk.kotlin.services.transcribestreaming.model.ParticipantRole? = null
/**
* Provides the sentiment that was detected in the specified segment.
*/
public var sentiment: aws.sdk.kotlin.services.transcribestreaming.model.Sentiment? = null
/**
* Contains transcribed text.
*/
public var transcript: kotlin.String? = null
/**
* The unique identifier that is associated with the specified `UtteranceEvent`.
*/
public var utteranceId: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.transcribestreaming.model.UtteranceEvent) : this() {
this.beginOffsetMillis = x.beginOffsetMillis
this.endOffsetMillis = x.endOffsetMillis
this.entities = x.entities
this.isPartial = x.isPartial
this.issuesDetected = x.issuesDetected
this.items = x.items
this.participantRole = x.participantRole
this.sentiment = x.sentiment
this.transcript = x.transcript
this.utteranceId = x.utteranceId
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.transcribestreaming.model.UtteranceEvent = UtteranceEvent(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy