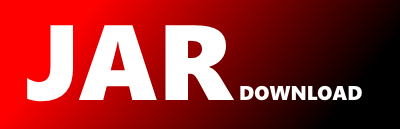
aws.sdk.kotlin.services.translate.model.InputDataConfig.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of translate Show documentation
Show all versions of translate Show documentation
The AWS Kotlin client for Translate
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.translate.model
/**
* The input configuration properties for requesting a batch translation job.
*/
class InputDataConfig private constructor(builder: BuilderImpl) {
/**
* Describes the format of the data that you submit to Amazon Translate as input. You can
* specify one of the following multipurpose internet mail extension (MIME) types:
* text/html: The input data consists of one or more HTML files. Amazon
* Translate translates only the text that resides in the html element in each
* file.
* text/plain: The input data consists of one or more unformatted text
* files. Amazon Translate translates every character in this type of input.
* application/vnd.openxmlformats-officedocument.wordprocessingml.document:
* The input data consists of one or more Word documents (.docx).
* application/vnd.openxmlformats-officedocument.presentationml.presentation:
* The input data consists of one or more PowerPoint Presentation files (.pptx).
* application/vnd.openxmlformats-officedocument.spreadsheetml.sheet: The
* input data consists of one or more Excel Workbook files (.xlsx).
* If you structure your input data as HTML, ensure that you set this parameter to
* text/html. By doing so, you cut costs by limiting the translation to the
* contents of the html element in each file. Otherwise, if you set this parameter
* to text/plain, your costs will cover the translation of every character.
*/
val contentType: String? = builder.contentType
/**
* The URI of the AWS S3 folder that contains the input file. The folder must be in the
* same Region as the API endpoint you are calling.
*/
val s3Uri: String? = builder.s3Uri
companion object {
@JvmStatic
fun fluentBuilder(): FluentBuilder = BuilderImpl()
internal fun builder(): DslBuilder = BuilderImpl()
operator fun invoke(block: DslBuilder.() -> kotlin.Unit): InputDataConfig = BuilderImpl().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("InputDataConfig(")
append("contentType=$contentType,")
append("s3Uri=$s3Uri)")
}
override fun hashCode(): kotlin.Int {
var result = contentType?.hashCode() ?: 0
result = 31 * result + (s3Uri?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (javaClass != other?.javaClass) return false
other as InputDataConfig
if (contentType != other.contentType) return false
if (s3Uri != other.s3Uri) return false
return true
}
fun copy(block: DslBuilder.() -> kotlin.Unit = {}): InputDataConfig = BuilderImpl(this).apply(block).build()
interface FluentBuilder {
fun build(): InputDataConfig
/**
* Describes the format of the data that you submit to Amazon Translate as input. You can
* specify one of the following multipurpose internet mail extension (MIME) types:
* text/html: The input data consists of one or more HTML files. Amazon
* Translate translates only the text that resides in the html element in each
* file.
* text/plain: The input data consists of one or more unformatted text
* files. Amazon Translate translates every character in this type of input.
* application/vnd.openxmlformats-officedocument.wordprocessingml.document:
* The input data consists of one or more Word documents (.docx).
* application/vnd.openxmlformats-officedocument.presentationml.presentation:
* The input data consists of one or more PowerPoint Presentation files (.pptx).
* application/vnd.openxmlformats-officedocument.spreadsheetml.sheet: The
* input data consists of one or more Excel Workbook files (.xlsx).
* If you structure your input data as HTML, ensure that you set this parameter to
* text/html. By doing so, you cut costs by limiting the translation to the
* contents of the html element in each file. Otherwise, if you set this parameter
* to text/plain, your costs will cover the translation of every character.
*/
fun contentType(contentType: String): FluentBuilder
/**
* The URI of the AWS S3 folder that contains the input file. The folder must be in the
* same Region as the API endpoint you are calling.
*/
fun s3Uri(s3Uri: String): FluentBuilder
}
interface DslBuilder {
/**
* Describes the format of the data that you submit to Amazon Translate as input. You can
* specify one of the following multipurpose internet mail extension (MIME) types:
* text/html: The input data consists of one or more HTML files. Amazon
* Translate translates only the text that resides in the html element in each
* file.
* text/plain: The input data consists of one or more unformatted text
* files. Amazon Translate translates every character in this type of input.
* application/vnd.openxmlformats-officedocument.wordprocessingml.document:
* The input data consists of one or more Word documents (.docx).
* application/vnd.openxmlformats-officedocument.presentationml.presentation:
* The input data consists of one or more PowerPoint Presentation files (.pptx).
* application/vnd.openxmlformats-officedocument.spreadsheetml.sheet: The
* input data consists of one or more Excel Workbook files (.xlsx).
* If you structure your input data as HTML, ensure that you set this parameter to
* text/html. By doing so, you cut costs by limiting the translation to the
* contents of the html element in each file. Otherwise, if you set this parameter
* to text/plain, your costs will cover the translation of every character.
*/
var contentType: String?
/**
* The URI of the AWS S3 folder that contains the input file. The folder must be in the
* same Region as the API endpoint you are calling.
*/
var s3Uri: String?
fun build(): InputDataConfig
}
private class BuilderImpl() : FluentBuilder, DslBuilder {
override var contentType: String? = null
override var s3Uri: String? = null
constructor(x: InputDataConfig) : this() {
this.contentType = x.contentType
this.s3Uri = x.s3Uri
}
override fun build(): InputDataConfig = InputDataConfig(this)
override fun contentType(contentType: String): FluentBuilder = apply { this.contentType = contentType }
override fun s3Uri(s3Uri: String): FluentBuilder = apply { this.s3Uri = s3Uri }
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy