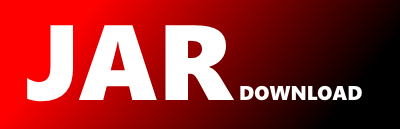
commonMain.aws.sdk.kotlin.services.wafv2.model.AwsManagedRulesAtpRuleSet.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of wafv2-jvm Show documentation
Show all versions of wafv2-jvm Show documentation
The AWS Kotlin client for WAFV2
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.wafv2.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Details for your use of the account takeover prevention managed rule group, `AWSManagedRulesATPRuleSet`. This configuration is used in `ManagedRuleGroupConfig`.
*/
public class AwsManagedRulesAtpRuleSet private constructor(builder: Builder) {
/**
* Allow the use of regular expressions in the login page path.
*/
public val enableRegexInPath: kotlin.Boolean = builder.enableRegexInPath
/**
* The path of the login endpoint for your application. For example, for the URL `https://example.com/web/login`, you would provide the path `/web/login`. Login paths that start with the path that you provide are considered a match. For example `/web/login` matches the login paths `/web/login`, `/web/login/`, `/web/loginPage`, and `/web/login/thisPage`, but doesn't match the login path `/home/web/login` or `/website/login`.
*
* The rule group inspects only HTTP `POST` requests to your specified login endpoint.
*/
public val loginPath: kotlin.String = requireNotNull(builder.loginPath) { "A non-null value must be provided for loginPath" }
/**
* The criteria for inspecting login requests, used by the ATP rule group to validate credentials usage.
*/
public val requestInspection: aws.sdk.kotlin.services.wafv2.model.RequestInspection? = builder.requestInspection
/**
* The criteria for inspecting responses to login requests, used by the ATP rule group to track login failure rates.
*
* Response inspection is available only in web ACLs that protect Amazon CloudFront distributions.
*
* The ATP rule group evaluates the responses that your protected resources send back to client login attempts, keeping count of successful and failed attempts for each IP address and client session. Using this information, the rule group labels and mitigates requests from client sessions and IP addresses that have had too many failed login attempts in a short amount of time.
*/
public val responseInspection: aws.sdk.kotlin.services.wafv2.model.ResponseInspection? = builder.responseInspection
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.wafv2.model.AwsManagedRulesAtpRuleSet = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("AwsManagedRulesAtpRuleSet(")
append("enableRegexInPath=$enableRegexInPath,")
append("loginPath=$loginPath,")
append("requestInspection=$requestInspection,")
append("responseInspection=$responseInspection")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = enableRegexInPath.hashCode()
result = 31 * result + (loginPath.hashCode())
result = 31 * result + (requestInspection?.hashCode() ?: 0)
result = 31 * result + (responseInspection?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as AwsManagedRulesAtpRuleSet
if (enableRegexInPath != other.enableRegexInPath) return false
if (loginPath != other.loginPath) return false
if (requestInspection != other.requestInspection) return false
if (responseInspection != other.responseInspection) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.wafv2.model.AwsManagedRulesAtpRuleSet = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* Allow the use of regular expressions in the login page path.
*/
public var enableRegexInPath: kotlin.Boolean = false
/**
* The path of the login endpoint for your application. For example, for the URL `https://example.com/web/login`, you would provide the path `/web/login`. Login paths that start with the path that you provide are considered a match. For example `/web/login` matches the login paths `/web/login`, `/web/login/`, `/web/loginPage`, and `/web/login/thisPage`, but doesn't match the login path `/home/web/login` or `/website/login`.
*
* The rule group inspects only HTTP `POST` requests to your specified login endpoint.
*/
public var loginPath: kotlin.String? = null
/**
* The criteria for inspecting login requests, used by the ATP rule group to validate credentials usage.
*/
public var requestInspection: aws.sdk.kotlin.services.wafv2.model.RequestInspection? = null
/**
* The criteria for inspecting responses to login requests, used by the ATP rule group to track login failure rates.
*
* Response inspection is available only in web ACLs that protect Amazon CloudFront distributions.
*
* The ATP rule group evaluates the responses that your protected resources send back to client login attempts, keeping count of successful and failed attempts for each IP address and client session. Using this information, the rule group labels and mitigates requests from client sessions and IP addresses that have had too many failed login attempts in a short amount of time.
*/
public var responseInspection: aws.sdk.kotlin.services.wafv2.model.ResponseInspection? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.wafv2.model.AwsManagedRulesAtpRuleSet) : this() {
this.enableRegexInPath = x.enableRegexInPath
this.loginPath = x.loginPath
this.requestInspection = x.requestInspection
this.responseInspection = x.responseInspection
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.wafv2.model.AwsManagedRulesAtpRuleSet = AwsManagedRulesAtpRuleSet(this)
/**
* construct an [aws.sdk.kotlin.services.wafv2.model.RequestInspection] inside the given [block]
*/
public fun requestInspection(block: aws.sdk.kotlin.services.wafv2.model.RequestInspection.Builder.() -> kotlin.Unit) {
this.requestInspection = aws.sdk.kotlin.services.wafv2.model.RequestInspection.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.wafv2.model.ResponseInspection] inside the given [block]
*/
public fun responseInspection(block: aws.sdk.kotlin.services.wafv2.model.ResponseInspection.Builder.() -> kotlin.Unit) {
this.responseInspection = aws.sdk.kotlin.services.wafv2.model.ResponseInspection.invoke(block)
}
internal fun correctErrors(): Builder {
if (loginPath == null) loginPath = ""
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy