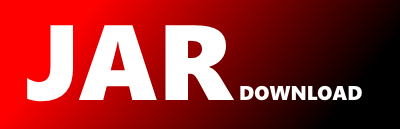
commonMain.aws.sdk.kotlin.services.wafv2.model.CaptchaAction.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of wafv2-jvm Show documentation
Show all versions of wafv2-jvm Show documentation
The AWS Kotlin client for WAFV2
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.wafv2.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Specifies that WAF should run a `CAPTCHA` check against the request:
* + If the request includes a valid, unexpired `CAPTCHA` token, WAF applies any custom request handling and labels that you've configured and then allows the web request inspection to proceed to the next rule, similar to a `CountAction`.
* + If the request doesn't include a valid, unexpired token, WAF discontinues the web ACL evaluation of the request and blocks it from going to its intended destination.WAF generates a response that it sends back to the client, which includes the following:
* + The header `x-amzn-waf-action` with a value of `captcha`.
* + The HTTP status code `405 Method Not Allowed`.
* + If the request contains an `Accept` header with a value of `text/html`, the response includes a `CAPTCHA` JavaScript page interstitial.
*
* You can configure the expiration time in the `CaptchaConfig``ImmunityTimeProperty` setting at the rule and web ACL level. The rule setting overrides the web ACL setting.
*
* This action option is available for rules. It isn't available for web ACL default actions.
*/
public class CaptchaAction private constructor(builder: Builder) {
/**
* Defines custom handling for the web request, used when the `CAPTCHA` inspection determines that the request's token is valid and unexpired.
*
* For information about customizing web requests and responses, see [Customizing web requests and responses in WAF](https://docs.aws.amazon.com/waf/latest/developerguide/waf-custom-request-response.html) in the *WAF Developer Guide*.
*/
public val customRequestHandling: aws.sdk.kotlin.services.wafv2.model.CustomRequestHandling? = builder.customRequestHandling
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.wafv2.model.CaptchaAction = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("CaptchaAction(")
append("customRequestHandling=$customRequestHandling")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = customRequestHandling?.hashCode() ?: 0
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as CaptchaAction
if (customRequestHandling != other.customRequestHandling) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.wafv2.model.CaptchaAction = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* Defines custom handling for the web request, used when the `CAPTCHA` inspection determines that the request's token is valid and unexpired.
*
* For information about customizing web requests and responses, see [Customizing web requests and responses in WAF](https://docs.aws.amazon.com/waf/latest/developerguide/waf-custom-request-response.html) in the *WAF Developer Guide*.
*/
public var customRequestHandling: aws.sdk.kotlin.services.wafv2.model.CustomRequestHandling? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.wafv2.model.CaptchaAction) : this() {
this.customRequestHandling = x.customRequestHandling
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.wafv2.model.CaptchaAction = CaptchaAction(this)
/**
* construct an [aws.sdk.kotlin.services.wafv2.model.CustomRequestHandling] inside the given [block]
*/
public fun customRequestHandling(block: aws.sdk.kotlin.services.wafv2.model.CustomRequestHandling.Builder.() -> kotlin.Unit) {
this.customRequestHandling = aws.sdk.kotlin.services.wafv2.model.CustomRequestHandling.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy