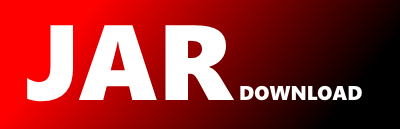
commonMain.aws.sdk.kotlin.services.wafv2.model.IpSet.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of wafv2-jvm Show documentation
Show all versions of wafv2-jvm Show documentation
The AWS Kotlin client for WAFV2
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.wafv2.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Contains zero or more IP addresses or blocks of IP addresses specified in Classless Inter-Domain Routing (CIDR) notation. WAF supports all IPv4 and IPv6 CIDR ranges except for /0. For information about CIDR notation, see the Wikipedia entry [Classless Inter-Domain Routing](https://en.wikipedia.org/wiki/Classless_Inter-Domain_Routing).
*
* WAF assigns an ARN to each `IPSet` that you create. To use an IP set in a rule, you provide the ARN to the Rule statement IPSetReferenceStatement.
*/
public class IpSet private constructor(builder: Builder) {
/**
* Contains an array of strings that specifies zero or more IP addresses or blocks of IP addresses that you want WAF to inspect for in incoming requests. All addresses must be specified using Classless Inter-Domain Routing (CIDR) notation. WAF supports all IPv4 and IPv6 CIDR ranges except for `/0`.
*
* Example address strings:
* + For requests that originated from the IP address 192.0.2.44, specify `192.0.2.44/32`.
* + For requests that originated from IP addresses from 192.0.2.0 to 192.0.2.255, specify `192.0.2.0/24`.
* + For requests that originated from the IP address 1111:0000:0000:0000:0000:0000:0000:0111, specify `1111:0000:0000:0000:0000:0000:0000:0111/128`.
* + For requests that originated from IP addresses 1111:0000:0000:0000:0000:0000:0000:0000 to 1111:0000:0000:0000:ffff:ffff:ffff:ffff, specify `1111:0000:0000:0000:0000:0000:0000:0000/64`.
*
* For more information about CIDR notation, see the Wikipedia entry [Classless Inter-Domain Routing](https://en.wikipedia.org/wiki/Classless_Inter-Domain_Routing).
*
* Example JSON `Addresses` specifications:
* + Empty array: `"Addresses": []`
* + Array with one address: `"Addresses": ["192.0.2.44/32"]`
* + Array with three addresses: `"Addresses": ["192.0.2.44/32", "192.0.2.0/24", "192.0.0.0/16"]`
* + INVALID specification: `"Addresses": [""]` INVALID
*/
public val addresses: List = requireNotNull(builder.addresses) { "A non-null value must be provided for addresses" }
/**
* The Amazon Resource Name (ARN) of the entity.
*/
public val arn: kotlin.String = requireNotNull(builder.arn) { "A non-null value must be provided for arn" }
/**
* A description of the IP set that helps with identification.
*/
public val description: kotlin.String? = builder.description
/**
* A unique identifier for the set. This ID is returned in the responses to create and list commands. You provide it to operations like update and delete.
*/
public val id: kotlin.String = requireNotNull(builder.id) { "A non-null value must be provided for id" }
/**
* The version of the IP addresses, either `IPV4` or `IPV6`.
*/
public val ipAddressVersion: aws.sdk.kotlin.services.wafv2.model.IpAddressVersion = requireNotNull(builder.ipAddressVersion) { "A non-null value must be provided for ipAddressVersion" }
/**
* The name of the IP set. You cannot change the name of an `IPSet` after you create it.
*/
public val name: kotlin.String = requireNotNull(builder.name) { "A non-null value must be provided for name" }
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.wafv2.model.IpSet = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("IpSet(")
append("addresses=$addresses,")
append("arn=$arn,")
append("description=$description,")
append("id=$id,")
append("ipAddressVersion=$ipAddressVersion,")
append("name=$name")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = addresses.hashCode()
result = 31 * result + (arn.hashCode())
result = 31 * result + (description?.hashCode() ?: 0)
result = 31 * result + (id.hashCode())
result = 31 * result + (ipAddressVersion.hashCode())
result = 31 * result + (name.hashCode())
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as IpSet
if (addresses != other.addresses) return false
if (arn != other.arn) return false
if (description != other.description) return false
if (id != other.id) return false
if (ipAddressVersion != other.ipAddressVersion) return false
if (name != other.name) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.wafv2.model.IpSet = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* Contains an array of strings that specifies zero or more IP addresses or blocks of IP addresses that you want WAF to inspect for in incoming requests. All addresses must be specified using Classless Inter-Domain Routing (CIDR) notation. WAF supports all IPv4 and IPv6 CIDR ranges except for `/0`.
*
* Example address strings:
* + For requests that originated from the IP address 192.0.2.44, specify `192.0.2.44/32`.
* + For requests that originated from IP addresses from 192.0.2.0 to 192.0.2.255, specify `192.0.2.0/24`.
* + For requests that originated from the IP address 1111:0000:0000:0000:0000:0000:0000:0111, specify `1111:0000:0000:0000:0000:0000:0000:0111/128`.
* + For requests that originated from IP addresses 1111:0000:0000:0000:0000:0000:0000:0000 to 1111:0000:0000:0000:ffff:ffff:ffff:ffff, specify `1111:0000:0000:0000:0000:0000:0000:0000/64`.
*
* For more information about CIDR notation, see the Wikipedia entry [Classless Inter-Domain Routing](https://en.wikipedia.org/wiki/Classless_Inter-Domain_Routing).
*
* Example JSON `Addresses` specifications:
* + Empty array: `"Addresses": []`
* + Array with one address: `"Addresses": ["192.0.2.44/32"]`
* + Array with three addresses: `"Addresses": ["192.0.2.44/32", "192.0.2.0/24", "192.0.0.0/16"]`
* + INVALID specification: `"Addresses": [""]` INVALID
*/
public var addresses: List? = null
/**
* The Amazon Resource Name (ARN) of the entity.
*/
public var arn: kotlin.String? = null
/**
* A description of the IP set that helps with identification.
*/
public var description: kotlin.String? = null
/**
* A unique identifier for the set. This ID is returned in the responses to create and list commands. You provide it to operations like update and delete.
*/
public var id: kotlin.String? = null
/**
* The version of the IP addresses, either `IPV4` or `IPV6`.
*/
public var ipAddressVersion: aws.sdk.kotlin.services.wafv2.model.IpAddressVersion? = null
/**
* The name of the IP set. You cannot change the name of an `IPSet` after you create it.
*/
public var name: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.wafv2.model.IpSet) : this() {
this.addresses = x.addresses
this.arn = x.arn
this.description = x.description
this.id = x.id
this.ipAddressVersion = x.ipAddressVersion
this.name = x.name
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.wafv2.model.IpSet = IpSet(this)
internal fun correctErrors(): Builder {
if (addresses == null) addresses = emptyList()
if (arn == null) arn = ""
if (id == null) id = ""
if (ipAddressVersion == null) ipAddressVersion = IpAddressVersion.SdkUnknown("no value provided")
if (name == null) name = ""
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy