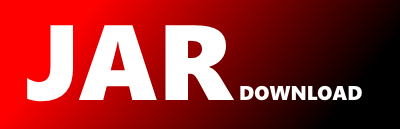
commonMain.aws.sdk.kotlin.services.wafv2.model.ManagedRuleSetVersion.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of wafv2-jvm Show documentation
Show all versions of wafv2-jvm Show documentation
The AWS Kotlin client for WAFV2
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.wafv2.model
import aws.smithy.kotlin.runtime.SdkDsl
import aws.smithy.kotlin.runtime.time.Instant
/**
* Information for a single version of a managed rule set.
*
* This is intended for use only by vendors of managed rule sets. Vendors are Amazon Web Services and Amazon Web Services Marketplace sellers.
*
* Vendors, you can use the managed rule set APIs to provide controlled rollout of your versioned managed rule group offerings for your customers. The APIs are `ListManagedRuleSets`, `GetManagedRuleSet`, `PutManagedRuleSetVersions`, and `UpdateManagedRuleSetVersionExpiryDate`.
*/
public class ManagedRuleSetVersion private constructor(builder: Builder) {
/**
* The Amazon Resource Name (ARN) of the vendor rule group that's used to define the published version of your managed rule group.
*/
public val associatedRuleGroupArn: kotlin.String? = builder.associatedRuleGroupArn
/**
* The web ACL capacity units (WCUs) required for this rule group.
*
* WAF uses WCUs to calculate and control the operating resources that are used to run your rules, rule groups, and web ACLs. WAF calculates capacity differently for each rule type, to reflect the relative cost of each rule. Simple rules that cost little to run use fewer WCUs than more complex rules that use more processing power. Rule group capacity is fixed at creation, which helps users plan their web ACL WCU usage when they use a rule group. For more information, see [WAF web ACL capacity units (WCU)](https://docs.aws.amazon.com/waf/latest/developerguide/aws-waf-capacity-units.html) in the *WAF Developer Guide*.
*/
public val capacity: kotlin.Long? = builder.capacity
/**
* The time that this version is set to expire.
*
* Times are in Coordinated Universal Time (UTC) format. UTC format includes the special designator, Z. For example, "2016-09-27T14:50Z".
*/
public val expiryTimestamp: aws.smithy.kotlin.runtime.time.Instant? = builder.expiryTimestamp
/**
* The amount of time you expect this version of your managed rule group to last, in days.
*/
public val forecastedLifetime: kotlin.Int? = builder.forecastedLifetime
/**
* The last time that you updated this version.
*
* Times are in Coordinated Universal Time (UTC) format. UTC format includes the special designator, Z. For example, "2016-09-27T14:50Z".
*/
public val lastUpdateTimestamp: aws.smithy.kotlin.runtime.time.Instant? = builder.lastUpdateTimestamp
/**
* The time that you first published this version.
*
* Times are in Coordinated Universal Time (UTC) format. UTC format includes the special designator, Z. For example, "2016-09-27T14:50Z".
*/
public val publishTimestamp: aws.smithy.kotlin.runtime.time.Instant? = builder.publishTimestamp
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.wafv2.model.ManagedRuleSetVersion = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("ManagedRuleSetVersion(")
append("associatedRuleGroupArn=$associatedRuleGroupArn,")
append("capacity=$capacity,")
append("expiryTimestamp=$expiryTimestamp,")
append("forecastedLifetime=$forecastedLifetime,")
append("lastUpdateTimestamp=$lastUpdateTimestamp,")
append("publishTimestamp=$publishTimestamp")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = associatedRuleGroupArn?.hashCode() ?: 0
result = 31 * result + (capacity?.hashCode() ?: 0)
result = 31 * result + (expiryTimestamp?.hashCode() ?: 0)
result = 31 * result + (forecastedLifetime ?: 0)
result = 31 * result + (lastUpdateTimestamp?.hashCode() ?: 0)
result = 31 * result + (publishTimestamp?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as ManagedRuleSetVersion
if (associatedRuleGroupArn != other.associatedRuleGroupArn) return false
if (capacity != other.capacity) return false
if (expiryTimestamp != other.expiryTimestamp) return false
if (forecastedLifetime != other.forecastedLifetime) return false
if (lastUpdateTimestamp != other.lastUpdateTimestamp) return false
if (publishTimestamp != other.publishTimestamp) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.wafv2.model.ManagedRuleSetVersion = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The Amazon Resource Name (ARN) of the vendor rule group that's used to define the published version of your managed rule group.
*/
public var associatedRuleGroupArn: kotlin.String? = null
/**
* The web ACL capacity units (WCUs) required for this rule group.
*
* WAF uses WCUs to calculate and control the operating resources that are used to run your rules, rule groups, and web ACLs. WAF calculates capacity differently for each rule type, to reflect the relative cost of each rule. Simple rules that cost little to run use fewer WCUs than more complex rules that use more processing power. Rule group capacity is fixed at creation, which helps users plan their web ACL WCU usage when they use a rule group. For more information, see [WAF web ACL capacity units (WCU)](https://docs.aws.amazon.com/waf/latest/developerguide/aws-waf-capacity-units.html) in the *WAF Developer Guide*.
*/
public var capacity: kotlin.Long? = null
/**
* The time that this version is set to expire.
*
* Times are in Coordinated Universal Time (UTC) format. UTC format includes the special designator, Z. For example, "2016-09-27T14:50Z".
*/
public var expiryTimestamp: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The amount of time you expect this version of your managed rule group to last, in days.
*/
public var forecastedLifetime: kotlin.Int? = null
/**
* The last time that you updated this version.
*
* Times are in Coordinated Universal Time (UTC) format. UTC format includes the special designator, Z. For example, "2016-09-27T14:50Z".
*/
public var lastUpdateTimestamp: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The time that you first published this version.
*
* Times are in Coordinated Universal Time (UTC) format. UTC format includes the special designator, Z. For example, "2016-09-27T14:50Z".
*/
public var publishTimestamp: aws.smithy.kotlin.runtime.time.Instant? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.wafv2.model.ManagedRuleSetVersion) : this() {
this.associatedRuleGroupArn = x.associatedRuleGroupArn
this.capacity = x.capacity
this.expiryTimestamp = x.expiryTimestamp
this.forecastedLifetime = x.forecastedLifetime
this.lastUpdateTimestamp = x.lastUpdateTimestamp
this.publishTimestamp = x.publishTimestamp
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.wafv2.model.ManagedRuleSetVersion = ManagedRuleSetVersion(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy