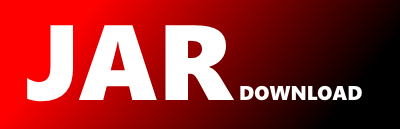
commonMain.aws.sdk.kotlin.services.wafv2.model.ResponseInspectionJson.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of wafv2-jvm Show documentation
Show all versions of wafv2-jvm Show documentation
The AWS Kotlin client for WAFV2
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.wafv2.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Configures inspection of the response JSON. WAF can inspect the first 65,536 bytes (64 KB) of the response JSON. This is part of the `ResponseInspection` configuration for `AWSManagedRulesATPRuleSet` and `AWSManagedRulesACFPRuleSet`.
*
* Response inspection is available only in web ACLs that protect Amazon CloudFront distributions.
*/
public class ResponseInspectionJson private constructor(builder: Builder) {
/**
* Values for the specified identifier in the response JSON that indicate a failed login or account creation attempt. To be counted as a failure, the value must be an exact match, including case. Each value must be unique among the success and failure values.
*
* JSON example: `"FailureValues": [ "False", "Failed" ]`
*/
public val failureValues: List = requireNotNull(builder.failureValues) { "A non-null value must be provided for failureValues" }
/**
* The identifier for the value to match against in the JSON. The identifier must be an exact match, including case.
*
* JSON examples: `"Identifier": [ "/login/success" ]` and `"Identifier": [ "/sign-up/success" ]`
*/
public val identifier: kotlin.String = requireNotNull(builder.identifier) { "A non-null value must be provided for identifier" }
/**
* Values for the specified identifier in the response JSON that indicate a successful login or account creation attempt. To be counted as a success, the value must be an exact match, including case. Each value must be unique among the success and failure values.
*
* JSON example: `"SuccessValues": [ "True", "Succeeded" ]`
*/
public val successValues: List = requireNotNull(builder.successValues) { "A non-null value must be provided for successValues" }
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.wafv2.model.ResponseInspectionJson = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("ResponseInspectionJson(")
append("failureValues=$failureValues,")
append("identifier=$identifier,")
append("successValues=$successValues")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = failureValues.hashCode()
result = 31 * result + (identifier.hashCode())
result = 31 * result + (successValues.hashCode())
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as ResponseInspectionJson
if (failureValues != other.failureValues) return false
if (identifier != other.identifier) return false
if (successValues != other.successValues) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.wafv2.model.ResponseInspectionJson = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* Values for the specified identifier in the response JSON that indicate a failed login or account creation attempt. To be counted as a failure, the value must be an exact match, including case. Each value must be unique among the success and failure values.
*
* JSON example: `"FailureValues": [ "False", "Failed" ]`
*/
public var failureValues: List? = null
/**
* The identifier for the value to match against in the JSON. The identifier must be an exact match, including case.
*
* JSON examples: `"Identifier": [ "/login/success" ]` and `"Identifier": [ "/sign-up/success" ]`
*/
public var identifier: kotlin.String? = null
/**
* Values for the specified identifier in the response JSON that indicate a successful login or account creation attempt. To be counted as a success, the value must be an exact match, including case. Each value must be unique among the success and failure values.
*
* JSON example: `"SuccessValues": [ "True", "Succeeded" ]`
*/
public var successValues: List? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.wafv2.model.ResponseInspectionJson) : this() {
this.failureValues = x.failureValues
this.identifier = x.identifier
this.successValues = x.successValues
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.wafv2.model.ResponseInspectionJson = ResponseInspectionJson(this)
internal fun correctErrors(): Builder {
if (failureValues == null) failureValues = emptyList()
if (identifier == null) identifier = ""
if (successValues == null) successValues = emptyList()
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy