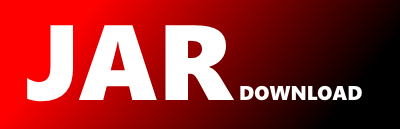
commonMain.aws.sdk.kotlin.services.wafv2.model.IpSetReferenceStatement.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of wafv2-jvm Show documentation
Show all versions of wafv2-jvm Show documentation
The AWS Kotlin client for WAFV2
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.wafv2.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* A rule statement used to detect web requests coming from particular IP addresses or address ranges. To use this, create an IPSet that specifies the addresses you want to detect, then use the ARN of that set in this statement. To create an IP set, see CreateIPSet.
*
* Each IP set rule statement references an IP set. You create and maintain the set independent of your rules. This allows you to use the single set in multiple rules. When you update the referenced set, WAF automatically updates all rules that reference it.
*/
public class IpSetReferenceStatement private constructor(builder: Builder) {
/**
* The Amazon Resource Name (ARN) of the IPSet that this statement references.
*/
public val arn: kotlin.String = requireNotNull(builder.arn) { "A non-null value must be provided for arn" }
/**
* The configuration for inspecting IP addresses in an HTTP header that you specify, instead of using the IP address that's reported by the web request origin. Commonly, this is the X-Forwarded-For (XFF) header, but you can specify any header name.
*
* If the specified header isn't present in the request, WAF doesn't apply the rule to the web request at all.
*/
public val ipSetForwardedIpConfig: aws.sdk.kotlin.services.wafv2.model.IpSetForwardedIpConfig? = builder.ipSetForwardedIpConfig
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.wafv2.model.IpSetReferenceStatement = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("IpSetReferenceStatement(")
append("arn=$arn,")
append("ipSetForwardedIpConfig=$ipSetForwardedIpConfig")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = arn.hashCode()
result = 31 * result + (ipSetForwardedIpConfig?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as IpSetReferenceStatement
if (arn != other.arn) return false
if (ipSetForwardedIpConfig != other.ipSetForwardedIpConfig) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.wafv2.model.IpSetReferenceStatement = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The Amazon Resource Name (ARN) of the IPSet that this statement references.
*/
public var arn: kotlin.String? = null
/**
* The configuration for inspecting IP addresses in an HTTP header that you specify, instead of using the IP address that's reported by the web request origin. Commonly, this is the X-Forwarded-For (XFF) header, but you can specify any header name.
*
* If the specified header isn't present in the request, WAF doesn't apply the rule to the web request at all.
*/
public var ipSetForwardedIpConfig: aws.sdk.kotlin.services.wafv2.model.IpSetForwardedIpConfig? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.wafv2.model.IpSetReferenceStatement) : this() {
this.arn = x.arn
this.ipSetForwardedIpConfig = x.ipSetForwardedIpConfig
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.wafv2.model.IpSetReferenceStatement = IpSetReferenceStatement(this)
/**
* construct an [aws.sdk.kotlin.services.wafv2.model.IpSetForwardedIpConfig] inside the given [block]
*/
public fun ipSetForwardedIpConfig(block: aws.sdk.kotlin.services.wafv2.model.IpSetForwardedIpConfig.Builder.() -> kotlin.Unit) {
this.ipSetForwardedIpConfig = aws.sdk.kotlin.services.wafv2.model.IpSetForwardedIpConfig.invoke(block)
}
internal fun correctErrors(): Builder {
if (arn == null) arn = ""
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy