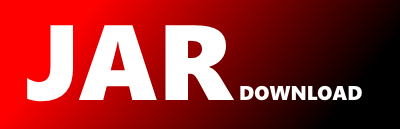
commonMain.aws.sdk.kotlin.services.wafv2.model.ManagedRuleGroupSummary.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of wafv2-jvm Show documentation
Show all versions of wafv2-jvm Show documentation
The AWS Kotlin client for WAFV2
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.wafv2.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* High-level information about a managed rule group, returned by ListAvailableManagedRuleGroups. This provides information like the name and vendor name, that you provide when you add a ManagedRuleGroupStatement to a web ACL. Managed rule groups include Amazon Web Services Managed Rules rule groups and Amazon Web Services Marketplace managed rule groups. To use any Amazon Web Services Marketplace managed rule group, first subscribe to the rule group through Amazon Web Services Marketplace.
*/
public class ManagedRuleGroupSummary private constructor(builder: Builder) {
/**
* The description of the managed rule group, provided by Amazon Web Services Managed Rules or the Amazon Web Services Marketplace seller who manages it.
*/
public val description: kotlin.String? = builder.description
/**
* The name of the managed rule group. You use this, along with the vendor name, to identify the rule group.
*/
public val name: kotlin.String? = builder.name
/**
* The name of the managed rule group vendor. You use this, along with the rule group name, to identify a rule group.
*/
public val vendorName: kotlin.String? = builder.vendorName
/**
* Indicates whether the managed rule group is versioned. If it is, you can retrieve the versions list by calling ListAvailableManagedRuleGroupVersions.
*/
public val versioningSupported: kotlin.Boolean = builder.versioningSupported
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.wafv2.model.ManagedRuleGroupSummary = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("ManagedRuleGroupSummary(")
append("description=$description,")
append("name=$name,")
append("vendorName=$vendorName,")
append("versioningSupported=$versioningSupported")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = description?.hashCode() ?: 0
result = 31 * result + (name?.hashCode() ?: 0)
result = 31 * result + (vendorName?.hashCode() ?: 0)
result = 31 * result + (versioningSupported.hashCode())
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as ManagedRuleGroupSummary
if (description != other.description) return false
if (name != other.name) return false
if (vendorName != other.vendorName) return false
if (versioningSupported != other.versioningSupported) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.wafv2.model.ManagedRuleGroupSummary = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The description of the managed rule group, provided by Amazon Web Services Managed Rules or the Amazon Web Services Marketplace seller who manages it.
*/
public var description: kotlin.String? = null
/**
* The name of the managed rule group. You use this, along with the vendor name, to identify the rule group.
*/
public var name: kotlin.String? = null
/**
* The name of the managed rule group vendor. You use this, along with the rule group name, to identify a rule group.
*/
public var vendorName: kotlin.String? = null
/**
* Indicates whether the managed rule group is versioned. If it is, you can retrieve the versions list by calling ListAvailableManagedRuleGroupVersions.
*/
public var versioningSupported: kotlin.Boolean = false
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.wafv2.model.ManagedRuleGroupSummary) : this() {
this.description = x.description
this.name = x.name
this.vendorName = x.vendorName
this.versioningSupported = x.versioningSupported
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.wafv2.model.ManagedRuleGroupSummary = ManagedRuleGroupSummary(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy