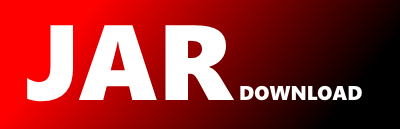
commonMain.aws.sdk.kotlin.services.wafv2.model.MobileSdkRelease.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of wafv2-jvm Show documentation
Show all versions of wafv2-jvm Show documentation
The AWS Kotlin client for WAFV2
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.wafv2.model
import aws.smithy.kotlin.runtime.SdkDsl
import aws.smithy.kotlin.runtime.time.Instant
/**
* Information for a release of the mobile SDK, including release notes and tags.
*
* The mobile SDK is not generally available. Customers who have access to the mobile SDK can use it to establish and manage WAF tokens for use in HTTP(S) requests from a mobile device to WAF. For more information, see [WAF client application integration](https://docs.aws.amazon.com/waf/latest/developerguide/waf-application-integration.html) in the *WAF Developer Guide*.
*/
public class MobileSdkRelease private constructor(builder: Builder) {
/**
* Notes describing the release.
*/
public val releaseNotes: kotlin.String? = builder.releaseNotes
/**
* The release version.
*/
public val releaseVersion: kotlin.String? = builder.releaseVersion
/**
* Tags that are associated with the release.
*/
public val tags: List? = builder.tags
/**
* The timestamp of the release.
*/
public val timestamp: aws.smithy.kotlin.runtime.time.Instant? = builder.timestamp
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.wafv2.model.MobileSdkRelease = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("MobileSdkRelease(")
append("releaseNotes=$releaseNotes,")
append("releaseVersion=$releaseVersion,")
append("tags=$tags,")
append("timestamp=$timestamp")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = releaseNotes?.hashCode() ?: 0
result = 31 * result + (releaseVersion?.hashCode() ?: 0)
result = 31 * result + (tags?.hashCode() ?: 0)
result = 31 * result + (timestamp?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as MobileSdkRelease
if (releaseNotes != other.releaseNotes) return false
if (releaseVersion != other.releaseVersion) return false
if (tags != other.tags) return false
if (timestamp != other.timestamp) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.wafv2.model.MobileSdkRelease = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* Notes describing the release.
*/
public var releaseNotes: kotlin.String? = null
/**
* The release version.
*/
public var releaseVersion: kotlin.String? = null
/**
* Tags that are associated with the release.
*/
public var tags: List? = null
/**
* The timestamp of the release.
*/
public var timestamp: aws.smithy.kotlin.runtime.time.Instant? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.wafv2.model.MobileSdkRelease) : this() {
this.releaseNotes = x.releaseNotes
this.releaseVersion = x.releaseVersion
this.tags = x.tags
this.timestamp = x.timestamp
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.wafv2.model.MobileSdkRelease = MobileSdkRelease(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy