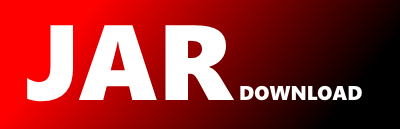
commonMain.aws.sdk.kotlin.services.wafv2.model.OverrideAction.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of wafv2-jvm Show documentation
Show all versions of wafv2-jvm Show documentation
The AWS Kotlin client for WAFV2
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.wafv2.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* The action to use in the place of the action that results from the rule group evaluation. Set the override action to none to leave the result of the rule group alone. Set it to count to override the result to count only.
*
* You can only use this for rule statements that reference a rule group, like `RuleGroupReferenceStatement` and `ManagedRuleGroupStatement`.
*
* This option is usually set to none. It does not affect how the rules in the rule group are evaluated. If you want the rules in the rule group to only count matches, do not use this and instead use the rule action override option, with `Count` action, in your rule group reference statement settings.
*/
public class OverrideAction private constructor(builder: Builder) {
/**
* Override the rule group evaluation result to count only.
*
* This option is usually set to none. It does not affect how the rules in the rule group are evaluated. If you want the rules in the rule group to only count matches, do not use this and instead use the rule action override option, with `Count` action, in your rule group reference statement settings.
*/
public val count: aws.sdk.kotlin.services.wafv2.model.CountAction? = builder.count
/**
* Don't override the rule group evaluation result. This is the most common setting.
*/
public val none: aws.sdk.kotlin.services.wafv2.model.NoneAction? = builder.none
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.wafv2.model.OverrideAction = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("OverrideAction(")
append("count=$count,")
append("none=$none")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = count?.hashCode() ?: 0
result = 31 * result + (none?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as OverrideAction
if (count != other.count) return false
if (none != other.none) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.wafv2.model.OverrideAction = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* Override the rule group evaluation result to count only.
*
* This option is usually set to none. It does not affect how the rules in the rule group are evaluated. If you want the rules in the rule group to only count matches, do not use this and instead use the rule action override option, with `Count` action, in your rule group reference statement settings.
*/
public var count: aws.sdk.kotlin.services.wafv2.model.CountAction? = null
/**
* Don't override the rule group evaluation result. This is the most common setting.
*/
public var none: aws.sdk.kotlin.services.wafv2.model.NoneAction? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.wafv2.model.OverrideAction) : this() {
this.count = x.count
this.none = x.none
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.wafv2.model.OverrideAction = OverrideAction(this)
/**
* construct an [aws.sdk.kotlin.services.wafv2.model.CountAction] inside the given [block]
*/
public fun count(block: aws.sdk.kotlin.services.wafv2.model.CountAction.Builder.() -> kotlin.Unit) {
this.count = aws.sdk.kotlin.services.wafv2.model.CountAction.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.wafv2.model.NoneAction] inside the given [block]
*/
public fun none(block: aws.sdk.kotlin.services.wafv2.model.NoneAction.Builder.() -> kotlin.Unit) {
this.none = aws.sdk.kotlin.services.wafv2.model.NoneAction.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy