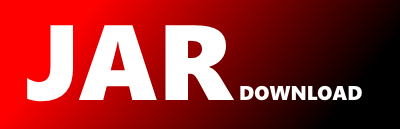
commonMain.aws.sdk.kotlin.services.wafv2.model.ResponseInspection.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of wafv2-jvm Show documentation
Show all versions of wafv2-jvm Show documentation
The AWS Kotlin client for WAFV2
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.wafv2.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* The criteria for inspecting responses to login requests and account creation requests, used by the ATP and ACFP rule groups to track login and account creation success and failure rates.
*
* Response inspection is available only in web ACLs that protect Amazon CloudFront distributions.
*
* The rule groups evaluates the responses that your protected resources send back to client login and account creation attempts, keeping count of successful and failed attempts from each IP address and client session. Using this information, the rule group labels and mitigates requests from client sessions and IP addresses with too much suspicious activity in a short amount of time.
*
* This is part of the `AWSManagedRulesATPRuleSet` and `AWSManagedRulesACFPRuleSet` configurations in `ManagedRuleGroupConfig`.
*
* Enable response inspection by configuring exactly one component of the response to inspect, for example, `Header` or `StatusCode`. You can't configure more than one component for inspection. If you don't configure any of the response inspection options, response inspection is disabled.
*/
public class ResponseInspection private constructor(builder: Builder) {
/**
* Configures inspection of the response body for success and failure indicators. WAF can inspect the first 65,536 bytes (64 KB) of the response body.
*/
public val bodyContains: aws.sdk.kotlin.services.wafv2.model.ResponseInspectionBodyContains? = builder.bodyContains
/**
* Configures inspection of the response header for success and failure indicators.
*/
public val header: aws.sdk.kotlin.services.wafv2.model.ResponseInspectionHeader? = builder.header
/**
* Configures inspection of the response JSON for success and failure indicators. WAF can inspect the first 65,536 bytes (64 KB) of the response JSON.
*/
public val json: aws.sdk.kotlin.services.wafv2.model.ResponseInspectionJson? = builder.json
/**
* Configures inspection of the response status code for success and failure indicators.
*/
public val statusCode: aws.sdk.kotlin.services.wafv2.model.ResponseInspectionStatusCode? = builder.statusCode
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.wafv2.model.ResponseInspection = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("ResponseInspection(")
append("bodyContains=$bodyContains,")
append("header=$header,")
append("json=$json,")
append("statusCode=$statusCode")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = bodyContains?.hashCode() ?: 0
result = 31 * result + (header?.hashCode() ?: 0)
result = 31 * result + (json?.hashCode() ?: 0)
result = 31 * result + (statusCode?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as ResponseInspection
if (bodyContains != other.bodyContains) return false
if (header != other.header) return false
if (json != other.json) return false
if (statusCode != other.statusCode) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.wafv2.model.ResponseInspection = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* Configures inspection of the response body for success and failure indicators. WAF can inspect the first 65,536 bytes (64 KB) of the response body.
*/
public var bodyContains: aws.sdk.kotlin.services.wafv2.model.ResponseInspectionBodyContains? = null
/**
* Configures inspection of the response header for success and failure indicators.
*/
public var header: aws.sdk.kotlin.services.wafv2.model.ResponseInspectionHeader? = null
/**
* Configures inspection of the response JSON for success and failure indicators. WAF can inspect the first 65,536 bytes (64 KB) of the response JSON.
*/
public var json: aws.sdk.kotlin.services.wafv2.model.ResponseInspectionJson? = null
/**
* Configures inspection of the response status code for success and failure indicators.
*/
public var statusCode: aws.sdk.kotlin.services.wafv2.model.ResponseInspectionStatusCode? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.wafv2.model.ResponseInspection) : this() {
this.bodyContains = x.bodyContains
this.header = x.header
this.json = x.json
this.statusCode = x.statusCode
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.wafv2.model.ResponseInspection = ResponseInspection(this)
/**
* construct an [aws.sdk.kotlin.services.wafv2.model.ResponseInspectionBodyContains] inside the given [block]
*/
public fun bodyContains(block: aws.sdk.kotlin.services.wafv2.model.ResponseInspectionBodyContains.Builder.() -> kotlin.Unit) {
this.bodyContains = aws.sdk.kotlin.services.wafv2.model.ResponseInspectionBodyContains.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.wafv2.model.ResponseInspectionHeader] inside the given [block]
*/
public fun header(block: aws.sdk.kotlin.services.wafv2.model.ResponseInspectionHeader.Builder.() -> kotlin.Unit) {
this.header = aws.sdk.kotlin.services.wafv2.model.ResponseInspectionHeader.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.wafv2.model.ResponseInspectionJson] inside the given [block]
*/
public fun json(block: aws.sdk.kotlin.services.wafv2.model.ResponseInspectionJson.Builder.() -> kotlin.Unit) {
this.json = aws.sdk.kotlin.services.wafv2.model.ResponseInspectionJson.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.wafv2.model.ResponseInspectionStatusCode] inside the given [block]
*/
public fun statusCode(block: aws.sdk.kotlin.services.wafv2.model.ResponseInspectionStatusCode.Builder.() -> kotlin.Unit) {
this.statusCode = aws.sdk.kotlin.services.wafv2.model.ResponseInspectionStatusCode.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy