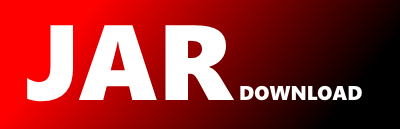
commonMain.aws.sdk.kotlin.services.wafv2.model.VisibilityConfig.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of wafv2-jvm Show documentation
Show all versions of wafv2-jvm Show documentation
The AWS Kotlin client for WAFV2
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.wafv2.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Defines and enables Amazon CloudWatch metrics and web request sample collection.
*/
public class VisibilityConfig private constructor(builder: Builder) {
/**
* Indicates whether the associated resource sends metrics to Amazon CloudWatch. For the list of available metrics, see [WAF Metrics](https://docs.aws.amazon.com/waf/latest/developerguide/monitoring-cloudwatch.html#waf-metrics) in the *WAF Developer Guide*.
*
* For web ACLs, the metrics are for web requests that have the web ACL default action applied. WAF applies the default action to web requests that pass the inspection of all rules in the web ACL without being either allowed or blocked. For more information, see [The web ACL default action](https://docs.aws.amazon.com/waf/latest/developerguide/web-acl-default-action.html) in the *WAF Developer Guide*.
*/
public val cloudWatchMetricsEnabled: kotlin.Boolean = builder.cloudWatchMetricsEnabled
/**
* A name of the Amazon CloudWatch metric dimension. The name can contain only the characters: A-Z, a-z, 0-9, - (hyphen), and _ (underscore). The name can be from one to 128 characters long. It can't contain whitespace or metric names that are reserved for WAF, for example `All` and `Default_Action`.
*/
public val metricName: kotlin.String = requireNotNull(builder.metricName) { "A non-null value must be provided for metricName" }
/**
* Indicates whether WAF should store a sampling of the web requests that match the rules. You can view the sampled requests through the WAF console.
*
* Request sampling doesn't provide a field redaction option, and any field redaction that you specify in your logging configuration doesn't affect sampling. The only way to exclude fields from request sampling is by disabling sampling in the web ACL visibility configuration.
*/
public val sampledRequestsEnabled: kotlin.Boolean = builder.sampledRequestsEnabled
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.wafv2.model.VisibilityConfig = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("VisibilityConfig(")
append("cloudWatchMetricsEnabled=$cloudWatchMetricsEnabled,")
append("metricName=$metricName,")
append("sampledRequestsEnabled=$sampledRequestsEnabled")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = cloudWatchMetricsEnabled.hashCode()
result = 31 * result + (metricName.hashCode())
result = 31 * result + (sampledRequestsEnabled.hashCode())
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as VisibilityConfig
if (cloudWatchMetricsEnabled != other.cloudWatchMetricsEnabled) return false
if (metricName != other.metricName) return false
if (sampledRequestsEnabled != other.sampledRequestsEnabled) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.wafv2.model.VisibilityConfig = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* Indicates whether the associated resource sends metrics to Amazon CloudWatch. For the list of available metrics, see [WAF Metrics](https://docs.aws.amazon.com/waf/latest/developerguide/monitoring-cloudwatch.html#waf-metrics) in the *WAF Developer Guide*.
*
* For web ACLs, the metrics are for web requests that have the web ACL default action applied. WAF applies the default action to web requests that pass the inspection of all rules in the web ACL without being either allowed or blocked. For more information, see [The web ACL default action](https://docs.aws.amazon.com/waf/latest/developerguide/web-acl-default-action.html) in the *WAF Developer Guide*.
*/
public var cloudWatchMetricsEnabled: kotlin.Boolean = false
/**
* A name of the Amazon CloudWatch metric dimension. The name can contain only the characters: A-Z, a-z, 0-9, - (hyphen), and _ (underscore). The name can be from one to 128 characters long. It can't contain whitespace or metric names that are reserved for WAF, for example `All` and `Default_Action`.
*/
public var metricName: kotlin.String? = null
/**
* Indicates whether WAF should store a sampling of the web requests that match the rules. You can view the sampled requests through the WAF console.
*
* Request sampling doesn't provide a field redaction option, and any field redaction that you specify in your logging configuration doesn't affect sampling. The only way to exclude fields from request sampling is by disabling sampling in the web ACL visibility configuration.
*/
public var sampledRequestsEnabled: kotlin.Boolean = false
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.wafv2.model.VisibilityConfig) : this() {
this.cloudWatchMetricsEnabled = x.cloudWatchMetricsEnabled
this.metricName = x.metricName
this.sampledRequestsEnabled = x.sampledRequestsEnabled
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.wafv2.model.VisibilityConfig = VisibilityConfig(this)
internal fun correctErrors(): Builder {
if (metricName == null) metricName = ""
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy