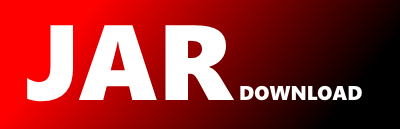
commonMain.aws.sdk.kotlin.services.xray.model.GetTimeSeriesServiceStatisticsRequest.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.xray.model
import aws.smithy.kotlin.runtime.SdkDsl
import aws.smithy.kotlin.runtime.time.Instant
public class GetTimeSeriesServiceStatisticsRequest private constructor(builder: Builder) {
/**
* The end of the time frame for which to aggregate statistics.
*/
public val endTime: aws.smithy.kotlin.runtime.time.Instant? = builder.endTime
/**
* A filter expression defining entities that will be aggregated for statistics. Supports ID, service, and edge functions. If no selector expression is specified, edge statistics are returned.
*/
public val entitySelectorExpression: kotlin.String? = builder.entitySelectorExpression
/**
* The forecasted high and low fault count values. Forecast enabled requests require the EntitySelectorExpression ID be provided.
*/
public val forecastStatistics: kotlin.Boolean? = builder.forecastStatistics
/**
* The Amazon Resource Name (ARN) of the group for which to pull statistics from.
*/
public val groupArn: kotlin.String? = builder.groupArn
/**
* The case-sensitive name of the group for which to pull statistics from.
*/
public val groupName: kotlin.String? = builder.groupName
/**
* Pagination token.
*/
public val nextToken: kotlin.String? = builder.nextToken
/**
* Aggregation period in seconds.
*/
public val period: kotlin.Int? = builder.period
/**
* The start of the time frame for which to aggregate statistics.
*/
public val startTime: aws.smithy.kotlin.runtime.time.Instant? = builder.startTime
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.xray.model.GetTimeSeriesServiceStatisticsRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("GetTimeSeriesServiceStatisticsRequest(")
append("endTime=$endTime,")
append("entitySelectorExpression=$entitySelectorExpression,")
append("forecastStatistics=$forecastStatistics,")
append("groupArn=$groupArn,")
append("groupName=$groupName,")
append("nextToken=$nextToken,")
append("period=$period,")
append("startTime=$startTime")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = endTime?.hashCode() ?: 0
result = 31 * result + (entitySelectorExpression?.hashCode() ?: 0)
result = 31 * result + (forecastStatistics?.hashCode() ?: 0)
result = 31 * result + (groupArn?.hashCode() ?: 0)
result = 31 * result + (groupName?.hashCode() ?: 0)
result = 31 * result + (nextToken?.hashCode() ?: 0)
result = 31 * result + (period ?: 0)
result = 31 * result + (startTime?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as GetTimeSeriesServiceStatisticsRequest
if (endTime != other.endTime) return false
if (entitySelectorExpression != other.entitySelectorExpression) return false
if (forecastStatistics != other.forecastStatistics) return false
if (groupArn != other.groupArn) return false
if (groupName != other.groupName) return false
if (nextToken != other.nextToken) return false
if (period != other.period) return false
if (startTime != other.startTime) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.xray.model.GetTimeSeriesServiceStatisticsRequest = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The end of the time frame for which to aggregate statistics.
*/
public var endTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* A filter expression defining entities that will be aggregated for statistics. Supports ID, service, and edge functions. If no selector expression is specified, edge statistics are returned.
*/
public var entitySelectorExpression: kotlin.String? = null
/**
* The forecasted high and low fault count values. Forecast enabled requests require the EntitySelectorExpression ID be provided.
*/
public var forecastStatistics: kotlin.Boolean? = null
/**
* The Amazon Resource Name (ARN) of the group for which to pull statistics from.
*/
public var groupArn: kotlin.String? = null
/**
* The case-sensitive name of the group for which to pull statistics from.
*/
public var groupName: kotlin.String? = null
/**
* Pagination token.
*/
public var nextToken: kotlin.String? = null
/**
* Aggregation period in seconds.
*/
public var period: kotlin.Int? = null
/**
* The start of the time frame for which to aggregate statistics.
*/
public var startTime: aws.smithy.kotlin.runtime.time.Instant? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.xray.model.GetTimeSeriesServiceStatisticsRequest) : this() {
this.endTime = x.endTime
this.entitySelectorExpression = x.entitySelectorExpression
this.forecastStatistics = x.forecastStatistics
this.groupArn = x.groupArn
this.groupName = x.groupName
this.nextToken = x.nextToken
this.period = x.period
this.startTime = x.startTime
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.xray.model.GetTimeSeriesServiceStatisticsRequest = GetTimeSeriesServiceStatisticsRequest(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy