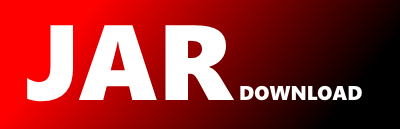
be.bagofwords.counts.DoubleCounter Maven / Gradle / Ivy
package be.bagofwords.counts;
import be.bagofwords.ui.UI;
import java.util.*;
public class DoubleCounter {
private final HashMap counts;
public DoubleCounter() {
counts = new HashMap<>();
}
public void inc(T s) {
inc(s, 1.0);
}
public void inc(T s, double value) {
if (counts.containsKey(s))
counts.put(s, counts.get(s) + value);
else
counts.put(s, value);
}
public void print() {
List> orderedCounts = new ArrayList<>(counts.entrySet());
Collections.sort(orderedCounts, new Comparator>() {
@Override
public int compare(Map.Entry o1, Map.Entry o2) {
return -o1.getValue().compareTo(o2.getValue());
}
});
for (Map.Entry s : orderedCounts) {
UI.write(s.getKey() + " (" + s.getValue() + ")");
}
}
public String[] keySet() {
Set var = counts.keySet();
return var.toArray(new String[var.size()]);
}
public List sortedKeys() {
Set keys = counts.keySet();
List result = new ArrayList<>(keys);
Collections.sort(result, new Comparator() {
@Override
public int compare(T o1, T o2) {
return -Double.compare(get(o1), get(o2));
}
});
return result;
}
public double get(T s) {
Double count = counts.get(s);
if (count == null) {
return 0;
} else {
return count;
}
}
public double getTotal() {
int total = 0;
for (Double val : counts.values()) {
total += val;
}
return total;
}
public double getMax() {
double max = 0;
for (Double val : counts.values()) {
max = Math.max(max, val);
}
return max;
}
public int size() {
return counts.size();
}
public void set(T s, double value) {
counts.put(s, value);
}
public void addAll(DoubleCounter other) {
for (Map.Entry entry : other.entrySet()) {
inc(entry.getKey(), entry.getValue());
}
}
private Set> entrySet() {
return counts.entrySet();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy