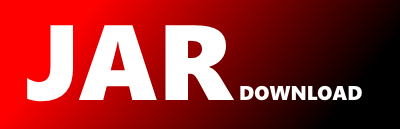
be.bagofwords.util.Utils Maven / Gradle / Ivy
package be.bagofwords.util;
import java.io.*;
import java.lang.reflect.Field;
import java.net.InetAddress;
import java.net.NetworkInterface;
import java.net.SocketException;
import java.util.*;
import java.util.Map.Entry;
import java.util.stream.Stream;
public class Utils {
public static ArrayList list() {
return new ArrayList<>();
}
public static int minFraq(int value, int divisor) {
int result = value / divisor;
if (value % divisor != 0)
return result + 1;
else
return result;
}
public static int minLog(int val) {
int exp = 0;
int currVal = 1;
while (currVal < val) {
exp++;
currVal *= 2;
}
return exp;
}
public static int readInt(byte[] data, int position, int numOfBytes) {
int result = 0;
for (int i = 0; i < numOfBytes; i++)
if (i > 0)
result = (result << 8) | ((int) data[position + i] & 0xFF);
else
result = (result << 8) | ((int) data[position + i]);
return result;
}
public static void writeInt(byte[] data, int position, int value, int numOfBytes) {
for (int i = numOfBytes - 1; i >= 0; i--) {
data[position + i] = (byte) value;
value = value >> 8;
}
}
private static final int[] starts;
static {
starts = new int[5];
for (int i = 1; i <= 4; i++)
starts[i] = -(1 << (i * 8 - 1));
}
public static String getIP() {
try {
Enumeration ifaces = NetworkInterface.getNetworkInterfaces();
for (NetworkInterface iface : Collections.list(ifaces)) {
if (!iface.isLoopback()) {
Enumeration raddrs = iface.getInetAddresses();
for (InetAddress raddr : Collections.list(raddrs)) {
String ip = raddr.toString().replaceFirst("/", "");
if (ip.matches("\\d+\\.\\d+\\.\\d+\\.\\d+"))
return ip;
}
}
}
} catch (SocketException e) {
e.printStackTrace();
}
return "127.0.0.1";
}
public static String getGitVersion() {
File file = new File(".git/logs/HEAD");
if (file.exists()) {
String lastLine = readLastLine(file);
String[] parts = lastLine.split(" ");
return parts[1];
} else {
throw new RuntimeException("Could not find .git directory!");
}
}
private static String readLastLine(File file) {
String lastLine = null;
try {
BufferedReader rdr = new BufferedReader(new FileReader(file));
String line;
while ((line = rdr.readLine()) != null) {
lastLine = line;
}
return lastLine;
} catch (IOException exp) {
throw new RuntimeException(exp);
}
}
public static ArrayList> reverseList(HashMap map) {
ArrayList> result = new ArrayList<>();
for (Entry entry : map.entrySet())
result.add(new Pair<>(entry.getValue(), entry.getKey()));
return result;
}
public static ArrayList> list(HashMap map) {
ArrayList> result = new ArrayList<>();
for (Entry entry : map.entrySet())
result.add(new Pair<>(entry.getKey(), entry.getValue()));
return result;
}
public static ArrayList list(List origList, T obj) {
ArrayList result = new ArrayList<>(origList);
result.add(obj);
return result;
}
public static ArrayList list(T... objs) {
ArrayList result = new ArrayList<>();
Collections.addAll(result, objs);
return result;
}
public static Pair pair(S obj1, T obj2) {
return new Pair<>(obj1, obj2);
}
public static HashMap map() {
return new HashMap<>();
}
public static HashMap map(ArrayList> values) {
HashMap res = map();
for (Pair val : values)
res.put(val.getFirst(), val.getSecond());
return res;
}
public static String getStackTrace(Throwable e) {
StringWriter writer = new StringWriter();
e.printStackTrace(new PrintWriter(writer));
return writer.getBuffer().toString();
}
public static void threadSleep(long ms) {
try {
Thread.sleep(ms);
} catch (InterruptedException e) {
//we don't like interrupted exceptions. Who came up with this idea?
}
}
public static boolean terminateWasRequestedForCurrentThread() {
Thread thread = Thread.currentThread();
if (thread instanceof SafeThread) {
return ((SafeThread) thread).isTerminateRequested();
} else {
return thread.isInterrupted();
}
}
public static List filter(List list, Filter filter) {
List result = new ArrayList<>();
for (T obj : list) {
if (filter.accept(obj)) {
result.add(obj);
}
}
return result;
}
public static void addLibraryPath(String pathToAdd) {
try {
final Field usrPathsField = ClassLoader.class.getDeclaredField("usr_paths");
usrPathsField.setAccessible(true);
//get array of paths
final String[] paths = (String[]) usrPathsField.get(null);
//check if the path to add is already present
for (String path : paths) {
if (path.equals(pathToAdd)) {
return;
}
}
//add the new path
final String[] newPaths = Arrays.copyOf(paths, paths.length + 1);
newPaths[newPaths.length - 1] = pathToAdd;
usrPathsField.set(null, newPaths);
} catch (Exception e) {
throw new RuntimeException(e);
}
}
public static String getStackTrace(StackTraceElement[] stackTrace) {
String result = "";
for (int i = stackTrace.length - 1; i >= 0; i--) {
StackTraceElement element = stackTrace[i];
result += element.getClassName() + "." + element.getMethodName() + "(" + element.getFileName() + ":" + element.getLineNumber() + ")";
if (i > 0) {
result += "\n";
}
}
return result;
}
public static Stream fasterParallelStream(Collection items) {
return splitListInSublists(items).parallelStream().flatMap(sublist -> sublist.stream());
}
private static List> splitListInSublists(Collection values) {
List> sublists = new ArrayList<>();
for (int i = 0; i < Runtime.getRuntime().availableProcessors() * 10; i++) {
sublists.add(new ArrayList<>());
}
int ind = 0;
for (T value : values) {
sublists.get(ind % sublists.size()).add(value);
ind++;
}
return sublists;
}
public static void noException(Action action) {
try {
action.run();
} catch (Exception ex) {
throw new RuntimeException("Unexpected exception", ex);
}
}
public static interface Action {
public void run() throws Exception;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy