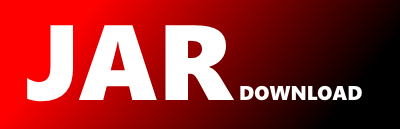
be.bagofwords.counts.Counter Maven / Gradle / Ivy
package be.bagofwords.counts;
import be.bagofwords.ui.UI;
import be.bagofwords.util.Pair;
import com.fasterxml.jackson.annotation.JsonIgnore;
import java.util.*;
import java.util.concurrent.Semaphore;
public class Counter {
private final Map counts;
private long cachedTotal;
private Semaphore lock;
public Counter() {
counts = new HashMap<>();
cachedTotal = -1;
lock = new Semaphore(1000);
}
public void inc(T s) {
inc(s, 1l);
}
public synchronized void inc(T s, long count) {
if (counts.containsKey(s)) {
lock.acquireUninterruptibly(1);
counts.put(s, counts.get(s) + count);
lock.release();
} else {
lock.acquireUninterruptibly(1000);
counts.put(s, count);
lock.release(1000);
}
cachedTotal = -1;
}
public void print() {
List> orderedCounts = new ArrayList<>(counts.entrySet());
Collections.sort(orderedCounts, new Comparator>() {
@Override
public int compare(Map.Entry o1, Map.Entry o2) {
return -o1.getValue().compareTo(o2.getValue());
}
});
for (Map.Entry s : orderedCounts) {
UI.write(s.getKey() + " (" + s.getValue() + ")");
}
}
public Set keySet() {
return counts.keySet();
}
public List sortedKeys() {
Set keys = counts.keySet();
List result = new ArrayList<>(keys);
Collections.sort(result, new Comparator() {
@Override
public int compare(T o1, T o2) {
return -Long.compare(get(o1), get(o2));
}
});
return result;
}
public long get(T s) {
Long count = counts.get(s);
if (count == null) {
return 0;
} else {
return count;
}
}
@JsonIgnore
public long getTotal() {
if (cachedTotal == -1) {
cachedTotal = 0;
for (Long val : counts.values()) {
cachedTotal += val;
}
}
return cachedTotal;
}
public Set> entrySet() {
return counts.entrySet();
}
public Counter clone() {
Counter clone = new Counter<>();
clone.getMap().putAll(getMap());
return clone;
}
@JsonIgnore
public Map getMap() {
return counts;
}
public int size() {
return counts.size();
}
public void set(T s, long value) {
counts.put(s, value);
}
public void addAll(Counter other) {
for (Map.Entry entry : other.entrySet()) {
inc(entry.getKey(), entry.getValue());
}
}
public void addAll(List> values) {
counts.clear();
for (Pair value : values) {
counts.put(value.getFirst(), value.getSecond());
}
}
public void trim(int maxSize) {
if (maxSize <= 0) {
throw new RuntimeException("Incorrect max size:" + maxSize);
}
if (size() > maxSize) {
List sortedKeys = sortedKeys();
for (int i = maxSize; i < sortedKeys.size(); i++) {
counts.remove(sortedKeys.get(i));
}
}
}
public void clear() {
counts.clear();
}
public List> getValuesAsList() {
List> result = new ArrayList<>();
for (Map.Entry entry : counts.entrySet()) {
result.add(new Pair<>(entry.getKey(), entry.getValue()));
}
return result;
}
public void setValuesAsList(List> values) {
counts.clear();
for (Pair value : values) {
counts.put(value.getFirst(), value.getSecond());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy