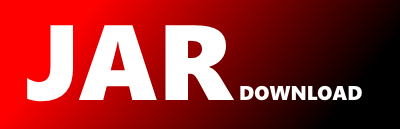
be.ordina.msdashboard.graph.GraphMapper Maven / Gradle / Ivy
/*
* Copyright 2012-2016 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package be.ordina.msdashboard.graph;
import be.ordina.msdashboard.nodes.model.Node;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import rx.functions.Func1;
import java.util.*;
import static be.ordina.msdashboard.nodes.model.NodeTypes.*;
import static be.ordina.msdashboard.graph.GraphRetriever.LINKS;
import static be.ordina.msdashboard.graph.GraphRetriever.NODES;
import static be.ordina.msdashboard.nodes.model.Node.DETAILS;
import static be.ordina.msdashboard.nodes.model.Node.ID;
import static be.ordina.msdashboard.nodes.model.Node.LANE;
/**
* @author Tim Ysewyn
*/
public class GraphMapper {
private static final Logger logger = LoggerFactory.getLogger(GraphMapper.class);
public static Func1, Map> toGraph() {
return (nodes) -> {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy