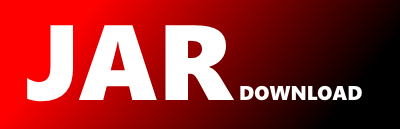
be.personify.iam.frontend.wicket.panel.common.ResourcePanel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of personify-frontend Show documentation
Show all versions of personify-frontend Show documentation
frontend library for different usages
package be.personify.iam.frontend.wicket.panel.common;
import java.util.List;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import org.apache.wicket.MarkupContainer;
import org.apache.wicket.injection.Injector;
import org.apache.wicket.markup.html.panel.EmptyPanel;
import org.apache.wicket.markup.html.panel.Panel;
import org.apache.wicket.model.IModel;
import org.apache.wicket.spring.injection.annot.SpringBean;
import org.springframework.core.env.Environment;
import org.springframework.hateoas.Link;
import org.springframework.hateoas.EntityModel;
import be.personify.iam.api.util.LinkUtil;
import be.personify.iam.frontend.wicket.model.common.ResourceModel;
/**
* A abstract resource panel
* @author vanderw
*
* @param the generic type
*/
public abstract class ResourcePanel extends Panel {
private static final Logger logger = LogManager.getLogger(ResourcePanel.class);
private static final long serialVersionUID = -7268964531051616124L;
public static final String SALT_KEY = "h";
protected static final String PANEL_BODY_NAME = "panel-body";
protected static final String EXPAND_LINK = "expandLink";
@SpringBean
private Environment environment;
private boolean showRelations;
private String parentName;
ClassInstanciator ci = new ClassInstanciator();
public ResourcePanel(String id, ResourceModel> model) {
super(id, model);
logger.debug("new resourcepanel {} with model {}", id, model);
Injector.get().inject(this);
}
public ResourcePanel(String id) {
super(id);
logger.debug("new resourcepanel {} with model {}", id);
Injector.get().inject(this);
}
@Override
protected void onInitialize() {
super.onInitialize();
}
public void setCustomModel(IModel model) {
logger.debug("setCustomModel {}", model);
setDefaultModel(model);
}
public void setCustomModel(ResourceModel> model) {
logger.debug("setCustomModel (ResourceModel) {}", model);
setDefaultModel(model);
}
public ResourceModel> getModel() {
return (ResourceModel>) getDefaultModel();
}
public boolean isShowRelations() {
logger.debug("isShowRelations {}", showRelations);
return showRelations;
}
public void setShowRelations(boolean showRelations) {
this.showRelations = showRelations;
}
public String getParentName() {
return parentName;
}
public void setParentName(String parentName) {
this.parentName = parentName;
}
public void addRelation(String parentName, String relationName, ResourceModel contestModel, boolean withRelations, MarkupContainer component) {
logger.debug("---------------- from parent {} add relation {} withrelations {}", parentName, relationName, withRelations);
//avoid loop
if ( getParentName() != null && getParentName().equals(relationName)) {
//why to lower case?
component.add(new EmptyPanel(relationName.toLowerCase()));
}
else {
Link link = findLink(relationName, contestModel.getLinks());
logger.debug("found link {} for relation {}", link, relationName );
if (link != null ) {
Panel panel = ci.getPanel(parentName, link, withRelations);
if ( panel != null ) {
component.add(panel);
}
else {
component.add(new EmptyPanel(relationName));
}
}
else {
component.add(new EmptyPanel(relationName));
}
}
}
public void addRelation(String parentName, String relationName, ResourceModel contestModel, boolean withRelations) {
addRelation(parentName, relationName, contestModel, withRelations, this);
}
public void addRelations(String parentName, ResourceModel> model, boolean withRelations) {
logger.debug("addRelations withrelations {}", withRelations);
List filteredLinks = LinkUtil.filterSelf(model.getLinks());
if ( filteredLinks != null ) {
for (Link link : filteredLinks) {
Panel panel = ci.getPanel(parentName, link, withRelations);
logger.debug("addRelations panel {} ", panel);
if ( panel == null ) {
add(new EmptyPanel(link.getRel().value()));
}
else if (panel instanceof ResourcesPanel) {
logger.debug("it's a resourcepanel");
ResourcesPanel> resourcesPanel = (ResourcesPanel) ci.getPanel(parentName, link, false);
if (resourcesPanel != null) {
if (resourcesPanel.getCustomModel().getCount() > 0 && withRelations) {
add(resourcesPanel);
} else {
logger.debug("panel is null for link " + link.getHref() + " " + link.getRel());
add(new EmptyPanel(link.getRel().value()));
}
}
}
}
}
}
public Link findLink(String type, List links) {
for (Link link : links) {
if (link.getRel().equals(type)) {
return link;
}
}
return null;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy