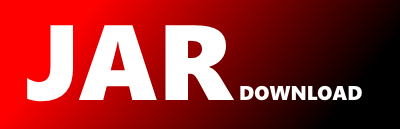
be.selckin.ws.util.java2php.ParticleInfo Maven / Gradle / Ivy
/**
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package be.selckin.ws.util.java2php;
import org.apache.cxf.common.xmlschema.SchemaCollection;
import org.apache.cxf.common.xmlschema.XmlSchemaConstants;
import org.apache.cxf.common.xmlschema.XmlSchemaUtils;
import org.apache.ws.commons.schema.XmlSchema;
import org.apache.ws.commons.schema.XmlSchemaElement;
import org.apache.ws.commons.schema.XmlSchemaObject;
import org.apache.ws.commons.schema.XmlSchemaParticle;
import org.apache.ws.commons.schema.XmlSchemaType;
import javax.xml.namespace.QName;
/**
* All the information needed to create the JavaScript for an Xml Schema element
* or xs:any.
*/
public final class ParticleInfo {
private String xmlName;
private XmlSchemaType type;
private boolean empty;
private long minOccurs;
private long maxOccurs;
private boolean nillable;
private boolean anyType;
public ParticleInfo() {
}
public static ParticleInfo forLocalItem(XmlSchemaObject sequenceObject, XmlSchema currentSchema, SchemaCollection schemaCollection, NamespacePrefixAccumulator prefixAccumulator, QName contextName) {
XmlSchemaParticle sequenceParticle = XmlSchemaUtils.getObjectParticle(sequenceObject, contextName);
ParticleInfo elementInfo = new ParticleInfo();
XmlSchemaParticle realParticle = sequenceParticle;
if (sequenceParticle instanceof XmlSchemaElement) {
XmlSchemaElement sequenceElement = (XmlSchemaElement) sequenceParticle;
if (sequenceElement.getRef().getTargetQName() != null) {
XmlSchemaElement refElement = sequenceElement.getRef().getTarget();
if (refElement == null) {
throw new UnsupportedConstruct("ELEMENT_DANGLING_REFERENCE " + sequenceElement.getQName() + sequenceElement.getRef().getTargetQName());
}
realParticle = refElement;
//elementInfo.global = true;
}
elementInfo.nillable = ((XmlSchemaElement) realParticle).isNillable();
}
elementInfo.minOccurs = sequenceParticle.getMinOccurs();
elementInfo.maxOccurs = sequenceParticle.getMaxOccurs();
if (!(realParticle instanceof XmlSchemaElement)) // any
throw new RuntimeException("any type not supported");
XmlSchemaElement element = (XmlSchemaElement) realParticle;
QName elementQName = XmlSchemaUtils.getElementQualifiedName(element, currentSchema);
String elementNamespaceURI = elementQName.getNamespaceURI();
boolean elementNoNamespace = "".equals(elementNamespaceURI);
XmlSchema elementSchema = null;
if (!elementNoNamespace) {
elementSchema = schemaCollection.getSchemaByTargetNamespace(elementNamespaceURI);
if (elementSchema == null) {
throw new RuntimeException("Missing schema " + elementNamespaceURI);
}
}
boolean qualified = !elementNoNamespace && XmlSchemaUtils.isElementQualified(element, true, currentSchema, elementSchema);
elementInfo.xmlName = prefixAccumulator.xmlElementString(elementQName, qualified);
elementInfo.type = element.getSchemaType();
if (elementInfo.type == null) {
if (element.getSchemaTypeName() == null || element.getSchemaTypeName().equals(XmlSchemaConstants.ANY_TYPE_QNAME)) {
elementInfo.anyType = true;
} else {
elementInfo.type = schemaCollection.getTypeByQName(element.getSchemaTypeName());
if (elementInfo.type == null
&& !element.getSchemaTypeName()
.getNamespaceURI().equals(XmlSchemaConstants.XSD_NAMESPACE_URI)) {
XmlSchemaUtils.unsupportedConstruct("MISSING_TYPE", element.getSchemaTypeName()
.toString(), element.getQName(), element);
}
}
} else if (elementInfo.type.getQName() != null && XmlSchemaConstants.ANY_TYPE_QNAME.equals(elementInfo.type.getQName())) {
elementInfo.anyType = true;
}
return elementInfo;
}
public String getXmlName() {
return xmlName;
}
public XmlSchemaType getType() {
return type;
}
public boolean isEmpty() {
return empty;
}
public long getMinOccurs() {
return minOccurs;
}
public long getMaxOccurs() {
return maxOccurs;
}
public boolean isArray() {
return maxOccurs > 1;
}
public boolean isOptional() {
return minOccurs == 0;
}
public boolean isNillable() {
return nillable;
}
public boolean isAnyType() {
return anyType;
}
@Override
public String toString() {
return "ItemInfo: " + xmlName;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy