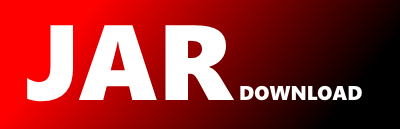
be.selckin.ws.util.java2php.Php Maven / Gradle / Ivy
/**
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package be.selckin.ws.util.java2php;
import be.selckin.ws.util.java2php.php.Argument;
import be.selckin.ws.util.java2php.php.PhpProperty;
import be.selckin.ws.util.java2php.php.TypeHint;
import com.google.common.base.Function;
import com.google.common.base.Joiner;
import com.google.common.base.Strings;
import com.google.common.collect.ImmutableSet;
import com.google.common.collect.Iterables;
import org.apache.ws.commons.schema.XmlSchemaComplexType;
import org.apache.ws.commons.schema.XmlSchemaSimpleContent;
import org.apache.ws.commons.schema.XmlSchemaSimpleType;
import org.apache.ws.commons.schema.XmlSchemaType;
import java.io.BufferedWriter;
import java.io.IOException;
import java.util.List;
import java.util.Stack;
public class Php {
private static final String NL = "\n";
private static final ImmutableSet PHP_KEYWORDS = ImmutableSet.of(
"abstract", "and", "array", "as", "break", "callable", "case", "catch", "class", "clone", "const", "continue",
"declare", "default", "die", "do", "echo", "else", "elseif", "empty", "enddeclare", "endfor", "endforeach",
"endif", "endswitch", "endwhile", "eval", "exit", "extends", "final", "for", "foreach", "function", "global",
"goto", "if", "implements", "include", "include_once", "instanceof", "insteadof", "interface", "isset", "list",
"namespace", "new", "or", "print", "private", "protected", "public", "require", "require_once", "return", "static",
"switch", "throw", "trait", "try", "unset", "use", "var", "while", "xor"
);
private BufferedWriter code;
private Stack prefixStack;
public Php(BufferedWriter writer) {
this.code = writer;
prefixStack = new Stack();
prefixStack.push("");
}
private String prefix() {
return prefixStack.peek();
}
public void appendField(String name, String defaultValue) throws IOException {
code.append(prefix());
code.append("private ").append("$").append(name).append(" = ").append(defaultValue).append(";");
code.append(NL);
}
public void appendConst(String name, String value) throws IOException {
code.append(prefix());
code.append("const ").append(mangleKeyword(name)).append(" = ").append("\"").append(value).append("\";");
code.append(NL);
}
public void appendLine(String line) throws IOException {
code.append(prefix());
code.append(line);
code.append(NL);
}
public void startBlock() throws IOException {
code.append(prefix());
code.append("{" + NL);
prefixStack.push(prefix() + " ");
}
public void endBlock() throws IOException {
prefixStack.pop();
code.append(prefix());
code.append("}" + NL);
}
public void indentMore() throws IOException {
prefixStack.push(prefix() + " ");
}
public void indentLess() throws IOException {
prefixStack.pop();
}
public void startNamespace(String name) throws IOException {
appendLine("namespace " + name);
startBlock();
}
public void endNamespace() throws IOException {
endBlock();
}
public void startClass(String name) throws IOException {
appendLine("class " + name);
startBlock();
}
public void endClass() throws IOException {
endBlock();
}
public void startInterface(String name) throws IOException {
appendLine("interface " + name);
startBlock();
}
public void endInterface() throws IOException {
endBlock();
}
public void startFunction(String name, List arguments) throws IOException {
code.append(prefix());
code.append("function ");
code.append(name);
code.append("(");
code.append(Joiner.on(", ").join(Iterables.transform(arguments, new Function() {
@Override
public String apply(Argument input) {
TypeHint typeHint = input.getTypeHint();
String hint = "";
if (typeHint.isArray()) {
hint = "array ";
} else if (!typeHint.isPrimitive()) {
hint = typeHint.getName() + ' ';
}
return hint + '$' + input.getName();
}
})));
code.append(")");
code.append(NL);
startBlock();
}
public void endFunction() throws IOException {
endBlock();
}
public void appendPhpDocVar(PhpProperty phpProperty) throws IOException {
String part = toPhpDocVar(phpProperty.getName(), phpProperty.getTypeHint());
if (!Strings.isNullOrEmpty(part))
appendLine("/* " + part + " */");
}
public void appendPhpDocVars(List properties) throws IOException {
appendLine("/**");
for (PhpProperty property : properties) {
String docPart = toPhpDocVar(property.getName(), property.getTypeHint());
if (!Strings.isNullOrEmpty(docPart)) {
appendLine(" * " + docPart);
}
}
appendLine("*/");
}
private String toPhpDocVar(String varName, TypeHint typeHint) throws IOException {
if (!typeHint.isPrimitive()) {
String hint = typeHint.getName() + (typeHint.isArray() ? "[]" : "");
return "@var $" + varName + ' ' + hint;
}
return "";
}
public void appendPhpDocParam(String name, PhpProperty phpProperty) throws IOException {
TypeHint typeHint = phpProperty.getTypeHint();
if (!typeHint.isPrimitive()) {
String hint = typeHint.getName() + (typeHint.isArray() ? "[]" : "");
appendLine("/* @param " + hint + " $" + name + " */");
}
}
public void appendPhpDocReturn(TypeHint typeHint) throws IOException {
if (!typeHint.isPrimitive()) {
String suffix = typeHint.isArray() ? "[]" : "";
appendLine("/* @return " + typeHint.getName() + suffix + " */");
}
}
/**
* We don't want to generate Javascript overhead for complex types with simple content models,
* at least until or unless we decide to cope with attributes in a general way.
*
* @param type
* @return
*/
public static boolean notVeryComplexType(XmlSchemaType type) {
return type instanceof XmlSchemaSimpleType
|| (type instanceof XmlSchemaComplexType
&& ((XmlSchemaComplexType) type).getContentModel() instanceof XmlSchemaSimpleContent);
}
private void assertNotKeyword(String name) {
if (isPHPKeyword(name))
throw new RuntimeException(name + " is a php keyword");
}
private boolean isPHPKeyword(String name) {
return PHP_KEYWORDS.contains(name.toLowerCase());
}
private String mangleKeyword(String name) {
return isPHPKeyword(name) ? name + "_" : name;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy