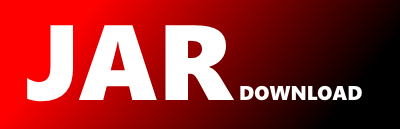
be.selckin.ws.util.java2php.ServicePHPBuilder Maven / Gradle / Ivy
/**
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package be.selckin.ws.util.java2php;
import be.selckin.ws.util.java2php.php.Argument;
import be.selckin.ws.util.java2php.php.Operation;
import be.selckin.ws.util.java2php.php.PhpService;
import be.selckin.ws.util.java2php.php.TypeHint;
import com.google.common.collect.Lists;
import org.apache.cxf.binding.soap.SoapBindingConstants;
import org.apache.cxf.binding.soap.SoapBindingFactory;
import org.apache.cxf.binding.soap.model.SoapBindingInfo;
import org.apache.cxf.common.WSDLConstants;
import org.apache.cxf.common.xmlschema.SchemaCollection;
import org.apache.cxf.common.xmlschema.XmlSchemaUtils;
import org.apache.cxf.service.ServiceModelVisitor;
import org.apache.cxf.service.model.BindingInfo;
import org.apache.cxf.service.model.EndpointInfo;
import org.apache.cxf.service.model.FaultInfo;
import org.apache.cxf.service.model.InterfaceInfo;
import org.apache.cxf.service.model.MessageInfo;
import org.apache.cxf.service.model.MessagePartInfo;
import org.apache.cxf.service.model.OperationInfo;
import org.apache.cxf.service.model.ServiceInfo;
import org.apache.ws.commons.schema.XmlSchema;
import org.apache.ws.commons.schema.XmlSchemaComplexType;
import org.apache.ws.commons.schema.XmlSchemaElement;
import org.apache.ws.commons.schema.XmlSchemaObject;
import org.apache.ws.commons.schema.XmlSchemaSequence;
import org.apache.ws.commons.schema.XmlSchemaSequenceMember;
import org.apache.ws.commons.schema.XmlSchemaType;
import javax.xml.namespace.QName;
import java.util.ArrayList;
import java.util.List;
public class ServicePHPBuilder extends ServiceModelVisitor {
private SoapBindingInfo soapBindingInfo;
private NameManager nameManager;
private OperationInfo currentOperation;
private SchemaCollection xmlSchemaCollection;
private XmlSchemaElement inputWrapperElement;
private XmlSchemaComplexType inputWrapperComplexType;
private NamespacePrefixAccumulator prefixAccumulator;
private List services = new ArrayList<>();
private PhpService current;
/**
* Construct builder object.
*
* @param serviceInfo CXF service model description of the service.
* @param prefixAccumulator object that keeps track of prefixes through an entire WSDL.
* @param nameManager object that generates names for JavaScript objects.
*/
public ServicePHPBuilder(ServiceInfo serviceInfo, NamespacePrefixAccumulator prefixAccumulator, NameManager nameManager) {
super(serviceInfo);
this.nameManager = nameManager;
xmlSchemaCollection = serviceInfo.getXmlSchemaCollection();
this.prefixAccumulator = prefixAccumulator;
}
public List getServices() {
return services;
}
@Override
public void begin(FaultInfo fault) {
}
@Override
public void begin(InterfaceInfo intf) {
current = new PhpService(intf.getName(), nameManager.getPhpNamespace(intf.getName()), intf.getName().getLocalPart());
services.add(current);
}
@Override
public void end(InterfaceInfo intf) {
current = null;
}
@Override
public void end(OperationInfo op) {
// we only process the wrapped operation, not the unwrapped alternative.
if (op.isUnwrapped()) {
return;
}
if (!op.isUnwrappedCapable())
throw new RuntimeException("only wrapped is supported");
collectWrapperElementInfo();
QName contextQName = inputWrapperComplexType.getQName();
if (contextQName == null) {
contextQName = inputWrapperElement.getQName();
}
XmlSchemaSequence sequence = XmlSchemaUtils.getSequence(inputWrapperComplexType);
XmlSchema wrapperSchema = xmlSchemaCollection.getSchemaByTargetNamespace(contextQName.getNamespaceURI());
List arguments = Lists.newArrayList();
for (int i = 0; i < sequence.getItems().size(); i++) {
XmlSchemaSequenceMember sequenceItem = sequence.getItems().get(i);
ParticleInfo itemInfo = ParticleInfo.forLocalItem((XmlSchemaObject) sequenceItem, wrapperSchema, xmlSchemaCollection, prefixAccumulator, contextQName);
TypeHint typeHint;
XmlSchemaType type = itemInfo.getType(); // null for an any.
if (type instanceof XmlSchemaComplexType) {
QName baseName;
if (type.getQName() != null) {
baseName = type.getQName();
} else {
baseName = ((XmlSchemaElement) sequenceItem).getQName();
}
typeHint = new TypeHint(itemInfo.isArray(), nameManager.getAbsolutePhpClassName(baseName));
} else {
typeHint = new TypeHint(itemInfo.isArray());
}
arguments.add(new Argument(typeHint, itemInfo.getXmlName()));
}
TypeHint returnType = findReturnType(currentOperation);
current.addOperation(new Operation(nameManager.getPhpName(currentOperation.getName()), returnType, arguments));
}
private TypeHint findReturnType(OperationInfo operationInfo) {
MessagePartInfo outputMessagePart = operationInfo.getOutput().getMessagePart(0);
XmlSchemaElement outputWrapperElement = (XmlSchemaElement) outputMessagePart.getXmlSchema();
if (outputWrapperElement == null) {
outputWrapperElement = XmlSchemaUtils.findElementByRefName(xmlSchemaCollection, outputMessagePart.getElementQName(), serviceInfo.getTargetNamespace());
}
return new TypeHint(outputWrapperElement.getMaxOccurs() > 1, nameManager.getAbsolutePhpClassName(outputWrapperElement.getQName()));
}
// This function finds all the information for the wrapper.
private void collectWrapperElementInfo() {
if (currentOperation.getInput() != null) {
MessagePartInfo inputWrapperPartInfo = currentOperation.getInput().getMessagePart(0);
assert inputWrapperPartInfo.isElement();
inputWrapperElement = (XmlSchemaElement) inputWrapperPartInfo.getXmlSchema();
if (inputWrapperElement == null) {
inputWrapperElement = XmlSchemaUtils.findElementByRefName(xmlSchemaCollection, inputWrapperPartInfo.getElementQName(), serviceInfo.getTargetNamespace());
}
inputWrapperComplexType = (XmlSchemaComplexType) inputWrapperElement.getSchemaType();
// the null name is probably something awful in RFSB.
if (inputWrapperComplexType == null) {
inputWrapperComplexType = (XmlSchemaComplexType) XmlSchemaUtils.getElementType(xmlSchemaCollection, serviceInfo.getTargetNamespace(), inputWrapperElement, null);
}
if (inputWrapperComplexType == null) {
throw new UnsupportedConstruct("MISSING_WRAPPER_TYPE");
}
if (inputWrapperComplexType.getQName() == null && !inputWrapperPartInfo.isElement()) {
throw new UnsupportedConstruct("NON_ELEMENT_ANON_TYPE_PART");
}
}
}
@Override
public void begin(ServiceInfo service) {
BindingInfo xml = null;
for (BindingInfo bindingInfo : service.getBindings()) {
if (SoapBindingConstants.SOAP11_BINDING_ID.equals(bindingInfo.getBindingId())
|| SoapBindingConstants.SOAP12_BINDING_ID.equals(bindingInfo.getBindingId())
|| SoapBindingFactory.SOAP_11_BINDING.equals(bindingInfo.getBindingId())
|| SoapBindingFactory.SOAP_12_BINDING.equals(bindingInfo.getBindingId())
) {
SoapBindingInfo sbi = (SoapBindingInfo) bindingInfo;
if (WSDLConstants.NS_SOAP11_HTTP_TRANSPORT.equals(sbi.getTransportURI())
|| WSDLConstants.NS_SOAP12_HTTP_BINDING.equals(sbi.getTransportURI())
|| "http://cxf.apache.org/transports/local".equals(sbi.getTransportURI())) {
soapBindingInfo = sbi;
break;
}
} else if (WSDLConstants.NS_BINDING_XML.equals(bindingInfo.getBindingId())) {
xml = bindingInfo;
}
}
// For now, we use soap if its available, and XML if it isn't.\
if (soapBindingInfo == null && xml == null) {
throw new UnsupportedConstruct("NO_USABLE_BINDING");
}
}
@Override
public void end(FaultInfo fault) {
}
@Override
public void end(MessageInfo msg) {
}
@Override
public void end(MessagePartInfo part) {
}
@Override
public void end(ServiceInfo service) {
}
@Override
public void begin(OperationInfo op) {
if (op.isUnwrapped()) {
return;
}
currentOperation = op;
}
@Override
public void begin(MessageInfo msg) {
}
@Override
public void begin(EndpointInfo endpointInfo) {
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy