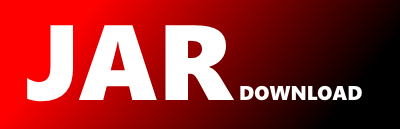
com.thematchbox.river.actions.IndexFeedback Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of AbstractRiver Show documentation
Show all versions of AbstractRiver Show documentation
This project contains an abstract implementation of an ElasticSearch River and is used as a basis for custom river implementations.
package com.thematchbox.river.actions;
/* Copyright 2015 theMatchBox
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
public class IndexFeedback {
private IndexJob indexJob;
private int successCount;
private int failCount;
private int total;
private long startTime;
private long currentTime;
private String errorMessage;
private boolean ignore = false;
public static enum State {
SCHEDULED,
FINISHED,
INDEXING,
FAILED
}
public synchronized IndexFeedback copy() {
return new IndexFeedback(indexJob, successCount, failCount, total, startTime, currentTime, errorMessage);
}
public synchronized void init(int total) {
successCount = 0;
failCount = 0;
this.total = total;
startTime = System.currentTimeMillis();
currentTime = startTime;
}
public IndexFeedback(IndexJob indexJob) {
this.indexJob = indexJob;
startTime = System.currentTimeMillis();
currentTime = startTime;
}
public synchronized void incrementSuccessCount(int value) {
successCount += value;
currentTime = System.currentTimeMillis();
}
public synchronized void incrementFailCount(int value) {
failCount += value;
currentTime = System.currentTimeMillis();
}
public synchronized void setErrorMessage(String errorMessage) {
this.errorMessage = errorMessage;
}
public IndexJob getIndexJob() {
return indexJob;
}
private IndexFeedback(IndexJob indexJob, int successCount, int failCount, int total, long startTime, long currentTime, String errorMessage) {
this.indexJob = indexJob;
this.successCount = successCount;
this.failCount = failCount;
this.total = total;
this.startTime = startTime;
this.currentTime = currentTime;
this.errorMessage = errorMessage;
}
public synchronized boolean isIgnore() {
return ignore;
}
public synchronized void setIgnore(boolean ignore) {
this.ignore = ignore;
}
public String getErrorMessage() {
return errorMessage;
}
public State getState() {
return errorMessage != null ? State.FAILED : total == 0 ? State.SCHEDULED : successCount + failCount >= total ? State.FINISHED : State.INDEXING;
}
public int getSuccessCount() {
return successCount;
}
public int getFailCount() {
return failCount;
}
public int getTotal() {
return total;
}
public long getStartTime() {
return startTime;
}
public long getCurrentTime() {
return currentTime;
}
public long getETA() {
if (getState() == State.FINISHED) return currentTime;
else {
double pace = getThroughputPerMillisecond();
int todo = total - failCount - successCount;
if (pace > 0 && todo > 0) {
return currentTime + (long) (todo / pace);
} else {
return 0;
}
}
}
public double getThroughputPerSecond() {
return getThroughputPerMillisecond() * 1000;
}
public double getThroughputPerMillisecond() {
long timePassed = currentTime - startTime;
double pace = 0;
if (timePassed > 0 && successCount > 0) {
pace = successCount / (double) timePassed;
}
return pace;
}
public double getProgress() {
return total > 0 ? (successCount + failCount) / (double) total : 0;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy