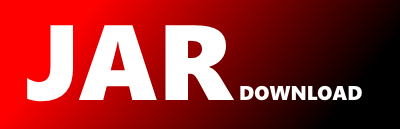
com.thematchbox.river.batch.BatchIndexKey Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of AbstractRiver Show documentation
Show all versions of AbstractRiver Show documentation
This project contains an abstract implementation of an ElasticSearch River and is used as a basis for custom river implementations.
package com.thematchbox.river.batch;
import com.thematchbox.river.actions.IndexKey;
import com.thematchbox.river.data.PersistentObject;
public abstract class BatchIndexKey, T extends PersistentObject> extends IndexKey {
public final ItemContainer payLoad;
public final String id;
protected BatchIndexKey(String indexName, String indexType, ItemContainer payLoad, String id) {
super(indexName, indexType);
this.payLoad = payLoad;
this.id = id;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
if (!super.equals(o)) return false;
BatchIndexKey that = (BatchIndexKey) o;
return id.equals(that.id);
}
@Override
public int hashCode() {
int result = super.hashCode();
result = 31 * result + id.hashCode();
return result;
}
@SuppressWarnings("UnusedDeclaration")
public ItemContainer getPayLoad() {
return payLoad;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy