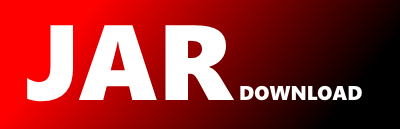
com.thematchbox.river.rest.RestResetIndexerStatusAction Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of AbstractRiver Show documentation
Show all versions of AbstractRiver Show documentation
This project contains an abstract implementation of an ElasticSearch River and is used as a basis for custom river implementations.
package com.thematchbox.river.rest;
import com.thematchbox.river.actions.IndexKey;
import com.thematchbox.river.indexers.MatchBoxIndexerManager;
import org.elasticsearch.client.Client;
import org.elasticsearch.common.inject.Inject;
import org.elasticsearch.common.settings.Settings;
import org.elasticsearch.common.xcontent.XContentBuilder;
import org.elasticsearch.common.xcontent.json.JsonXContent;
import org.elasticsearch.rest.*;
import java.io.IOException;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class RestResetIndexerStatusAction extends BaseRestHandler {
public static final String INDEX_NAME = "indexName";
public static final String INDEX_TYPE = "indexType";
private MatchBoxIndexerManager riverIndexingManager;
@Inject
public RestResetIndexerStatusAction(Settings settings, RestController controller, Client client, MatchBoxIndexerManager riverIndexingManager) {
super(settings, controller, client);
this.riverIndexingManager = riverIndexingManager;
controller.registerHandler(RestRequest.Method.DELETE, "/_matchboxClearIndexerStatus", this);
controller.registerHandler(RestRequest.Method.DELETE, "/_matchboxClearIndexerStatus/{" + INDEX_NAME + "}", this);
controller.registerHandler(RestRequest.Method.DELETE, "/_matchboxClearIndexerStatus/{" + INDEX_NAME + "}/{"+ INDEX_TYPE + "}", this);
}
@Override
protected void handleRequest(RestRequest request, RestChannel channel, Client client) throws Exception {
final String indexName = request.hasParam(INDEX_NAME) ? request.param(INDEX_NAME) : null;
final String indexType = request.hasParam(INDEX_TYPE) ? request.param(INDEX_TYPE) : null;
List keys = riverIndexingManager.getMatchingKeys(indexName, indexType);
Map responseMap = new HashMap<>();
for (IndexKey key : keys) {
responseMap.put(key, riverIndexingManager.clearStatus(key));
}
try {
XContentBuilder builder = JsonXContent.contentBuilder();
RestHelper.appendResponseMap(builder, responseMap);
channel.sendResponse(new BytesRestResponse(RestStatus.OK, builder));
} catch (IOException e) {
failed(channel, e);
}
}
private void failed(RestChannel channel, Throwable e) {
try {
channel.sendResponse(new BytesRestResponse(channel, e));
} catch (IOException e1) {
logger.error("Failed to send failure response", e1);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy