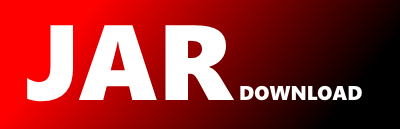
be.ugent.rml.records.JSONRecord Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rmlmapper Show documentation
Show all versions of rmlmapper Show documentation
The RMLMapper executes RML rules to generate high quality Linked Data from multiple originally (semi-)structured data sources.
package be.ugent.rml.records;
import com.jayway.jsonpath.JsonPath;
import com.jayway.jsonpath.PathNotFoundException;
import net.minidev.json.JSONArray;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.util.ArrayList;
import java.util.List;
/**
* This class is a specific implementation of a record for JSON.
* Every record corresponds with a JSON object in a data source.
*/
public class JSONRecord extends Record {
protected Logger logger = LoggerFactory.getLogger(this.getClass());
private String path;
private Object document;
public JSONRecord(Object document, String path) {
this.path = path;
this.document = document;
}
/**
* This method returns the objects for a reference (JSONPath) in the record.
* @param value the reference for which objects need to be returned.
* @return a list of objects for the reference.
*/
@Override
public List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy