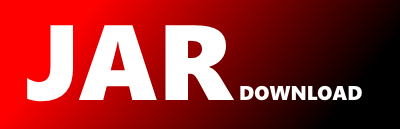
be.unamur.inference.web.UserSessionBuilder Maven / Gradle / Ivy
package be.unamur.inference.web;
import java.io.InputStream;
import java.util.Iterator;
import java.util.Vector;
import be.unamur.inference.web.exception.SessionBuildException;
/**
* Represents an abstract {@link UserSessionBuilder}. This object encapsulates
* methods to manage the building, filtering and dispatching the
* {@link UserSession}s get from an {@link InputStream}. The filtering is done
* using include and exclude {@link UserRequestFilter}s and the processing is
* delegated to {@link UserSessionProcessor}s registered as listeners of this
* builder object.
*
* @author Xavier Devroey - [email protected]
*
* @param The type of {@link UserSession} to consider.
* @param The type of {@link UserRequest} to consider.
*/
public abstract class UserSessionBuilder, U extends UserRequest> {
/**
* Listener notified when a session is built.
*/
private Vector> listeners;
/**
* Only requests satisfying all the include filters are considered for
* sessions.
*/
private Vector> includes;
/**
* Only requests that does not satisfy all the exclude filters are
* considered for sessions.
*/
private Vector> excludes;
/**
* Creates a new user session builder.
*/
public UserSessionBuilder() {
this.listeners = new Vector>();
this.includes = new Vector>();
this.excludes = new Vector>();
}
/**
* Add a listener notified when a session is built (during buildSession
* call).
*
* @param listener The listener to add.
* @return This object.
*/
public UserSessionBuilder addListener(UserSessionProcessor listener) {
this.listeners.add(listener);
return this;
}
/**
* Notifies the listeners that the given session has been built.
*
* @param session The session given to the listeners.
*/
protected void sessionCompleted(T session) {
for (UserSessionProcessor p : this.listeners) {
p.process(session);
}
}
/**
* Add an include filter to this builder. Only requests satisfying all the
* include filters are added to sessions.
*
* @param filter The filter to add
* @return This object.
*/
public UserSessionBuilder include(UserRequestFilter filter) {
this.includes.add(filter);
return this;
}
/**
* Add an include filter to this builder. Only requests that does not
* satisfy all the exclude filters are added to sessions.
*
* @param filter The filter to add
* @return This object.
*/
public UserSessionBuilder exclude(UserRequestFilter filter) {
this.excludes.add(filter);
return this;
}
/**
* Check if the request is accepted according to the include and exclude
* filters.
*
* @param req The request to process.
* @return True if the request satisfy all the include filters and does not
* satisfy all the exclude filters.
*/
protected boolean isAcceptedRequest(U req) {
boolean ok = true;
Iterator> it = this.includes.iterator();
UserRequestFilter f;
// Check includes
while (ok && it.hasNext()) {
f = it.next();
ok = ok && f.filter(req);
}
// Check excludes
it = this.excludes.iterator();
while (ok && it.hasNext()) {
f = it.next();
ok = ok && !f.filter(req);
}
return ok;
}
/**
* Build the session from the different request of the {@link InputStream}.
* Each time a session is build, the sessionCompleted(Session) method is
* called to notify the listeners of the builder. All the requests in the
* builded sessions have to satisfy isAcceptedRequest(Request).
*
* @param input The {@link InputStream} to read.
* @throws SessionBuildException If an exception occurs during the building
* of the sessions.
*/
public abstract void buildSessions(InputStream input) throws SessionBuildException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy