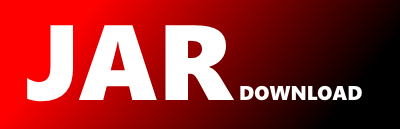
be.yildizgames.common.logging.logback.LogbackConfigFileGenerator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of common-logging-logback Show documentation
Show all versions of common-logging-logback Show documentation
Logback implementation for Common Logging library.
/*
* This file is part of the Yildiz-Engine project, licenced under the MIT License (MIT)
*
* Copyright (c) 2019 Grégory Van den Borre
*
* More infos available: https://engine.yildiz-games.be
*
* Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated
* documentation files (the "Software"), to deal in the Software without restriction, including without
* limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies
* of the Software, and to permit persons to whom the Software is furnished to do so,
* subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all copies or substantial
* portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE
* WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS
* OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM,
* DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
*
*/
package be.yildizgames.common.logging.logback;
import be.yildizgames.common.logging.LoggerConfiguration;
import java.util.ArrayList;
import java.util.List;
/**
* Generate a logback configuration file.
*
* @author Grégory Van den Borre
*/
class LogbackConfigFileGenerator {
/**
* Simple indentation spaces.
*/
private static final String INDENT = " ";
/**
* Double indentation spaces.
*/
private static final String INDENT2 = INDENT + INDENT;
/**
* TCP output.
*/
private static final String TCP = "TCP";
/**
* File output.
*/
private static final String FILE = "FILE";
/**
* Console output.
*/
private static final String CONSOLE = "CONSOLE";
/**
* Appender tag.
*/
private static final String APPENDER_NAME = INDENT + "";
/**
* Encoder tag.
*/
private static final String ENCODER = INDENT2 + "\n";
/**
* Closed pattern tag.
*/
private static final String PATTERN_CLOSE = "\n";
/**
* Closed appender tag.
*/
private static final String APPENDER_CLOSE = INDENT + " \n";
/**
* Closed encoder tag.
*/
private static final String ENCODER_CLOSE = INDENT2 + "\n";
/**
* Generate the content from the configuration.
*
* @param configuration Configuration to use.
* @return The generated content.
*/
final String generate(final LoggerConfiguration configuration) {
LogbackLoggerLevelMapper mapper = new LogbackLoggerLevelMapper();
StringBuilder builder = new StringBuilder();
builder.append("\n");
List appender = new ArrayList<>();
for(LoggerConfiguration.SupportedOutput out : configuration.getLoggerOutputs()) {
switch (out) {
case TCP:
this.generateTcp(builder, configuration);
appender.add(TCP);
break;
case FILE:
this.generateFile(builder, configuration);
appender.add(FILE);
break;
default:
this.generateConsole(builder, configuration);
appender.add(CONSOLE);
break;
}
}
builder
.append(" \n");
appender.forEach(a -> builder
.append(" \n"));
builder.append(" \n");
for (String disabled : configuration.getLoggerToDisable()) {
if (!disabled.isEmpty() && !disabled.isBlank()) {
builder.append(" \n");
}
}
builder.append(" \n");
return builder.toString();
}
/**
* Generate content for the console output.
*
* @param builder stringBuilder.
* @param configuration configuration to use.
*/
private void generateConsole(StringBuilder builder, LoggerConfiguration configuration) {
builder
.append(APPENDER_NAME)
.append(CONSOLE)
.append("\" class=\"ch.qos.logback.core.ConsoleAppender\">\n")
.append(ENCODER)
.append(PATTERN)
.append(configuration.getLoggerPattern())
.append(PATTERN_CLOSE)
.append(ENCODER_CLOSE)
.append(APPENDER_CLOSE);
}
/**
* Generate content for the file output.
*
* @param builder stringBuilder.
* @param configuration configuration to use.
*/
private void generateFile(StringBuilder builder, LoggerConfiguration configuration) {
builder
.append(" \n")
.append(APPENDER_NAME)
.append(FILE)
.append("\" class=\"ch.qos.logback.core.FileAppender\">\n")
.append(" ")
.append(configuration.getLoggerOutputFile())
.append("-${byDay}.txt \n")
.append(" true \n")
.append(ENCODER)
.append(PATTERN)
.append(configuration.getLoggerPattern())
.append(PATTERN_CLOSE)
.append(ENCODER_CLOSE)
.append(APPENDER_CLOSE);
}
/**
* Generate content for the tcp output.
*
* @param builder stringBuilder.
* @param configuration configuration to use.
*/
private void generateTcp(StringBuilder builder, LoggerConfiguration configuration) {
builder
.append(APPENDER_NAME)
.append(TCP)
.append("\" class=\"com.splunk.logging.TcpAppender\">\n")
.append(" ")
.append(configuration.getLoggerTcpHost())
.append(" \n")
.append(" ")
.append(configuration.getLoggerTcpPort())
.append(" \n")
.append(" \n")
.append(PATTERN)
.append(configuration.getLoggerPattern())
.append(PATTERN_CLOSE)
.append(" \n")
.append(APPENDER_CLOSE);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy