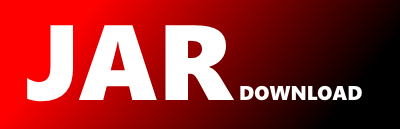
aQute.bnd.stream.MapStream Maven / Gradle / Ivy
The newest version!
package aQute.bnd.stream;
import java.util.AbstractMap.SimpleImmutableEntry;
import java.util.Arrays;
import java.util.Collection;
import java.util.Comparator;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.BiFunction;
import java.util.function.BiPredicate;
import java.util.function.BinaryOperator;
import java.util.function.Consumer;
import java.util.function.DoubleConsumer;
import java.util.function.Function;
import java.util.function.IntConsumer;
import java.util.function.LongConsumer;
import java.util.function.Predicate;
import java.util.function.Supplier;
import java.util.function.ToDoubleBiFunction;
import java.util.function.ToIntBiFunction;
import java.util.function.ToLongBiFunction;
import java.util.stream.BaseStream;
import java.util.stream.Collector;
import java.util.stream.Collectors;
import java.util.stream.DoubleStream;
import java.util.stream.IntStream;
import java.util.stream.LongStream;
import java.util.stream.Stream;
public interface MapStream extends BaseStream, MapStream> {
static MapStream of(Map extends K, ? extends V> map) {
return of(map.entrySet());
}
static MapStream ofNullable(Map extends K, ? extends V> map) {
return (map != null) ? of(map) : empty();
}
static MapStream of(Collection extends Entry extends K, ? extends V>> collection) {
return of(collection.stream());
}
static MapStream ofNullable(Collection extends Entry extends K, ? extends V>> collection) {
return (collection != null) ? of(collection) : empty();
}
static MapStream of(Stream extends Entry extends K, ? extends V>> stream) {
return new EntryPipeline<>(stream);
}
static MapStream ofNullable(Stream extends Entry extends K, ? extends V>> stream) {
return (stream != null) ? of(stream) : empty();
}
static MapStream concat(MapStream extends K, ? extends V> a, MapStream extends K, ? extends V> b) {
return of(Stream.concat(a.entries(), b.entries()));
}
static MapStream empty() {
return of(Stream.empty());
}
static MapStream of(K k1, V v1) {
return of(Stream.of(entry(k1, v1)));
}
static MapStream of(K k1, V v1, K k2, V v2) {
return ofEntries(entry(k1, v1), entry(k2, v2));
}
static MapStream of(K k1, V v1, K k2, V v2, K k3, V v3) {
return ofEntries(entry(k1, v1), entry(k2, v2), entry(k3, v3));
}
static MapStream of(K k1, V v1, K k2, V v2, K k3, V v3, K k4, V v4) {
return ofEntries(entry(k1, v1), entry(k2, v2), entry(k3, v3), entry(k4, v4));
}
@SafeVarargs
static MapStream ofEntries(Entry extends K, ? extends V>... entries) {
return of(Arrays.stream(entries));
}
static MapStream ofEntries(Stream extends O> stream,
Function super O, ? extends Entry extends K, ? extends V>> entryMapper) {
return of(stream.map(entryMapper));
}
static Entry entry(K key, V value) {
return new SimpleImmutableEntry<>(key, value);
}
Stream> entries();
Stream keys();
Stream values();
MapStream distinct();
MapStream filter(BiPredicate super K, ? super V> filter);
MapStream filterKey(Predicate super K> filter);
MapStream filterValue(Predicate super V> filter);
MapStream map(BiFunction super K, ? super V, ? extends Entry extends R, ? extends S>> mapper);
MapStream mapKey(Function super K, ? extends R> mapper);
MapStream mapValue(Function super V, ? extends S> mapper);
Stream mapToObj(BiFunction super K, ? super V, ? extends O> mapper);
IntStream mapToInt(ToIntBiFunction super K, ? super V> mapper);
LongStream mapToLong(ToLongBiFunction super K, ? super V> mapper);
DoubleStream mapToDouble(ToDoubleBiFunction super K, ? super V> mapper);
MapStream flatMap(
BiFunction super K, ? super V, ? extends MapStream extends R, ? extends S>> mapper);
MapStream flatMapKey(Function super K, ? extends Stream extends R>> mapper);
MapStream flatMapValue(Function super V, ? extends Stream extends S>> mapper);
Stream flatMapToObj(BiFunction super K, ? super V, ? extends Stream extends O>> mapper);
IntStream flatMapToInt(BiFunction super K, ? super V, ? extends IntStream> mapper);
LongStream flatMapToLong(BiFunction super K, ? super V, ? extends LongStream> mapper);
DoubleStream flatMapToDouble(BiFunction super K, ? super V, ? extends DoubleStream> mapper);
MapStream peek(BiConsumer super K, ? super V> peek);
MapStream peekKey(Consumer super K> peek);
MapStream peekValue(Consumer super V> peek);
MapStream sorted();
MapStream sorted(Comparator super Entry> comparator);
MapStream sortedByKey();
MapStream sortedByKey(Comparator super K> comparator);
MapStream sortedByValue();
MapStream sortedByValue(Comparator super V> comparator);
MapStream limit(long maxSize);
MapStream skip(long n);
long count();
void forEach(BiConsumer super K, ? super V> consumer);
void forEachOrdered(BiConsumer super K, ? super V> consumer);
boolean anyMatch(BiPredicate super K, ? super V> predicate);
boolean allMatch(BiPredicate super K, ? super V> predicate);
boolean noneMatch(BiPredicate super K, ? super V> predicate);
R collect(Supplier supplier, BiConsumer> accumulator,
BiConsumer combiner);
R collect(Collector super Entry extends K, ? extends V>, A, R> collector);
static Collector super Entry extends K, ? extends V>, ?, Map> toMap() {
return Collectors.toMap(Entry::getKey, Entry::getValue);
}
static Collector super Entry extends K, ? extends V>, ?, Map> toMap(
BinaryOperator mergeFunction) {
return Collectors.toMap(Entry::getKey, Entry::getValue, mergeFunction);
}
static > Collector super Entry extends K, ? extends V>, ?, M> toMap(
BinaryOperator mergeFunction, Supplier mapSupplier) {
return Collectors.toMap(Entry::getKey, Entry::getValue, mergeFunction, mapSupplier);
}
Optional> max(Comparator super Entry> comparator);
Optional> maxByKey(Comparator super K> comparator);
Optional> maxByValue(Comparator super V> comparator);
Optional> min(Comparator super Entry> comparator);
Optional> minByKey(Comparator super K> comparator);
Optional> minByValue(Comparator super V> comparator);
Optional> findAny();
Optional> findFirst();
Entry[] toArray();
MapStream takeWhile(BiPredicate super K, ? super V> predicate);
MapStream takeWhileKey(Predicate super K> predicate);
MapStream takeWhileValue(Predicate super V> predicate);
MapStream dropWhile(BiPredicate super K, ? super V> predicate);
MapStream dropWhileKey(Predicate super K> predicate);
MapStream dropWhileValue(Predicate super V> predicate);
MapStream mapMulti(TriConsumer super K, ? super V, ? super BiConsumer> mapper);
MapStream mapMultiKey(BiConsumer super K, ? super Consumer> mapper);
MapStream mapMultiValue(BiConsumer super V, ? super Consumer> mapper);
Stream mapMultiToObj(TriConsumer super K, ? super V, ? super Consumer> mapper);
IntStream mapMultiToInt(TriConsumer super K, ? super V, ? super IntConsumer> mapper);
LongStream mapMultiToLong(TriConsumer super K, ? super V, ? super LongConsumer> mapper);
DoubleStream mapMultiToDouble(TriConsumer super K, ? super V, ? super DoubleConsumer> mapper);
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy