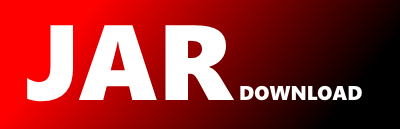
biz.gabrys.maven.plugin.util.timer.SystemTimer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of plugin-utils Show documentation
Show all versions of plugin-utils Show documentation
A Java library which contains utilities for Maven 2 plugins.
The newest version!
/*
* Maven Plugin Utils
* http://maven-plugin-utils.projects.gabrys.biz/
*
* Copyright (c) 2015 Adam Gabrys
*
* This file is licensed under the BSD 3-Clause (the "License").
* You may not use this file except in compliance with the License.
* You may obtain:
* - a copy of the License at project page
* - a template of the License at https://opensource.org/licenses/BSD-3-Clause
*/
package biz.gabrys.maven.plugin.util.timer;
/**
*
* Default implementation of the {@link Timer} which based on the {@link System#currentTimeMillis()} method.
*
*
* Example:
*
*
*
* public class ExampleMojo extends AbstractMojo {
*
* public void execute() {
* {@link Timer} timer = {@link SystemTimer#getStartedTimer() SystemTimer.getStartedTimer()};
*
* // logic
* ...
*
* getLog().info("Finished in " + timer.stop());
* }
* }
*
*
* @since 1.0
*/
public class SystemTimer implements Timer {
private final Object mutex = new Object();
private Long startTime;
private volatile TimeSpan time;
/**
* Constructs a new instance.
* @since 1.0
*/
public SystemTimer() {
// do nothing
}
@Override
public void start() {
synchronized (mutex) {
time = null;
startTime = System.currentTimeMillis();
}
}
@Override
public TimeSpan stop() {
if (time == null) {
synchronized (mutex) {
if (time == null) {
time = getTime();
}
}
}
return time;
}
/**
* {@inheritDoc} The current counted time span is equal to:
*
* - {@code null} when timer has not been started
* - {@code current time - start time} when timer has been started and has not been stopped
* - {@code counted time during stop} when timer has been stopped
*
* @since 2.0.0
*/
@Override
public TimeSpan getTime() {
if (startTime == null) {
return null;
}
if (time == null) {
return new TimeSpan(System.currentTimeMillis() - startTime);
}
return time;
}
/**
* Returns a new started timer.
* @return the new timer.
* @since 1.0
* @see #start()
*/
public static SystemTimer getStartedTimer() {
final SystemTimer timer = new SystemTimer();
timer.start();
return timer;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy