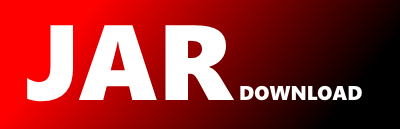
com.lambdaworks.redis.RedisCommandBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lettuce Show documentation
Show all versions of lettuce Show documentation
Advanced and thread-safe Java Redis client for synchronous, asynchronous, and
reactive usage. Supports Cluster, Sentinel, Pipelining, Auto-Reconnect, Codecs
and much more.
The newest version!
/*
* Copyright 2011-2016 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.lambdaworks.redis;
import static com.lambdaworks.redis.LettuceStrings.string;
import static com.lambdaworks.redis.protocol.CommandKeyword.*;
import static com.lambdaworks.redis.protocol.CommandType.*;
import java.util.*;
import java.nio.ByteBuffer;
import java.util.Date;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.lambdaworks.redis.codec.RedisCodec;
import com.lambdaworks.redis.internal.LettuceAssert;
import com.lambdaworks.redis.internal.LettuceLists;
import com.lambdaworks.redis.output.*;
import com.lambdaworks.redis.protocol.BaseRedisCommandBuilder;
import com.lambdaworks.redis.protocol.Command;
import com.lambdaworks.redis.protocol.CommandArgs;
import com.lambdaworks.redis.protocol.RedisCommand;
/**
* @param
* @param
* @author Mark Paluch
*/
@SuppressWarnings({ "unchecked", "Convert2Diamond", "WeakerAccess", "varargs" })
class RedisCommandBuilder extends BaseRedisCommandBuilder {
static final String MUST_NOT_CONTAIN_NULL_ELEMENTS = "must not contain null elements";
static final String MUST_NOT_BE_EMPTY = "must not be empty";
static final String MUST_NOT_BE_NULL = "must not be null";
private static final byte[] MINUS_BYTES = { '-' };
private static final byte[] PLUS_BYTES = { '+' };
public RedisCommandBuilder(RedisCodec codec) {
super(codec);
}
public Command append(K key, V value) {
notNullKey(key);
return createCommand(APPEND, new IntegerOutput(codec), key, value);
}
public Command auth(String password) {
LettuceAssert.notNull(password, "Password " + MUST_NOT_BE_NULL);
LettuceAssert.notEmpty(password, "Password " + MUST_NOT_BE_EMPTY);
CommandArgs args = new CommandArgs(codec).add(password);
return createCommand(AUTH, new StatusOutput(codec), args);
}
public Command bgrewriteaof() {
return createCommand(BGREWRITEAOF, new StatusOutput(codec));
}
public Command bgsave() {
return createCommand(BGSAVE, new StatusOutput(codec));
}
public Command bitcount(K key) {
notNullKey(key);
CommandArgs args = new CommandArgs(codec).addKey(key);
return createCommand(BITCOUNT, new IntegerOutput(codec), args);
}
public Command bitcount(K key, long start, long end) {
notNullKey(key);
CommandArgs args = new CommandArgs(codec);
args.addKey(key).add(start).add(end);
return createCommand(BITCOUNT, new IntegerOutput(codec), args);
}
public Command> bitfield(K key, BitFieldArgs bitFieldArgs) {
notNullKey(key);
LettuceAssert.notNull(bitFieldArgs, "BitFieldArgs must not be null");
CommandArgs args = new CommandArgs(codec);
args.addKey(key);
bitFieldArgs.build(args);
return createCommand(BITFIELD, (CommandOutput) new ArrayOutput(codec), args);
}
public Command>> bitfieldValue(K key, BitFieldArgs bitFieldArgs) {
notNullKey(key);
LettuceAssert.notNull(bitFieldArgs, "BitFieldArgs must not be null");
CommandArgs args = new CommandArgs(codec);
args.addKey(key);
bitFieldArgs.build(args);
return createCommand(BITFIELD, (CommandOutput) new ValueValueListOutput(codec), args);
}
public Command bitpos(K key, boolean state) {
notNullKey(key);
CommandArgs args = new CommandArgs(codec);
args.addKey(key).add(state ? 1 : 0);
return createCommand(BITPOS, new IntegerOutput(codec), args);
}
public Command bitpos(K key, boolean state, long start, long end) {
notNullKey(key);
CommandArgs args = new CommandArgs(codec);
args.addKey(key).add(state ? 1 : 0).add(start).add(end);
return createCommand(BITPOS, new IntegerOutput(codec), args);
}
public Command bitopAnd(K destination, K... keys) {
LettuceAssert.notNull(destination, "Destination " + MUST_NOT_BE_NULL);
notEmpty(keys);
CommandArgs args = new CommandArgs(codec);
args.add(AND).addKey(destination).addKeys(keys);
return createCommand(BITOP, new IntegerOutput(codec), args);
}
public Command bitopNot(K destination, K source) {
LettuceAssert.notNull(destination, "Destination " + MUST_NOT_BE_NULL);
LettuceAssert.notNull(source, "Source " + MUST_NOT_BE_NULL);
CommandArgs args = new CommandArgs(codec);
args.add(NOT).addKey(destination).addKey(source);
return createCommand(BITOP, new IntegerOutput(codec), args);
}
public Command bitopOr(K destination, K... keys) {
LettuceAssert.notNull(destination, "Destination " + MUST_NOT_BE_NULL);
notEmpty(keys);
CommandArgs args = new CommandArgs(codec);
args.add(OR).addKey(destination).addKeys(keys);
return createCommand(BITOP, new IntegerOutput(codec), args);
}
public Command bitopXor(K destination, K... keys) {
LettuceAssert.notNull(destination, "Destination " + MUST_NOT_BE_NULL);
notEmpty(keys);
CommandArgs args = new CommandArgs(codec);
args.add(XOR).addKey(destination).addKeys(keys);
return createCommand(BITOP, new IntegerOutput(codec), args);
}
public Command> blpop(long timeout, K... keys) {
notEmpty(keys);
CommandArgs args = new CommandArgs(codec).addKeys(keys).add(timeout);
return createCommand(BLPOP, new KeyValueOutput(codec), args);
}
public Command> brpop(long timeout, K... keys) {
notEmpty(keys);
CommandArgs args = new CommandArgs(codec).addKeys(keys).add(timeout);
return createCommand(BRPOP, new KeyValueOutput(codec), args);
}
public Command brpoplpush(long timeout, K source, K destination) {
LettuceAssert.notNull(source, "Source " + MUST_NOT_BE_NULL);
LettuceAssert.notNull(destination, "Destination " + MUST_NOT_BE_NULL);
CommandArgs args = new CommandArgs(codec);
args.addKey(source).addKey(destination).add(timeout);
return createCommand(BRPOPLPUSH, new ValueOutput(codec), args);
}
public Command clientGetname() {
CommandArgs args = new CommandArgs(codec).add(GETNAME);
return createCommand(CLIENT, new KeyOutput(codec), args);
}
public Command clientSetname(K name) {
LettuceAssert.notNull(name, "Name " + MUST_NOT_BE_NULL);
CommandArgs args = new CommandArgs(codec).add(SETNAME).addKey(name);
return createCommand(CLIENT, new StatusOutput(codec), args);
}
public Command clientKill(String addr) {
LettuceAssert.notNull(addr, "Addr " + MUST_NOT_BE_NULL);
LettuceAssert.notEmpty(addr, "Addr " + MUST_NOT_BE_EMPTY);
CommandArgs args = new CommandArgs(codec).add(KILL).add(addr);
return createCommand(CLIENT, new StatusOutput(codec), args);
}
public Command clientKill(KillArgs killArgs) {
LettuceAssert.notNull(killArgs, "KillArgs " + MUST_NOT_BE_NULL);
CommandArgs args = new CommandArgs(codec).add(KILL);
killArgs.build(args);
return createCommand(CLIENT, new IntegerOutput(codec), args);
}
public Command clientPause(long timeout) {
CommandArgs args = new CommandArgs(codec).add(PAUSE).add(timeout);
return createCommand(CLIENT, new StatusOutput(codec), args);
}
public Command clientList() {
CommandArgs args = new CommandArgs(codec).add(LIST);
return createCommand(CLIENT, new StatusOutput(codec), args);
}
public Command> command() {
CommandArgs args = new CommandArgs(codec);
return createCommand(COMMAND, new ArrayOutput(codec), args);
}
public Command> commandInfo(String... commands) {
LettuceAssert.notNull(commands, "Commands " + MUST_NOT_BE_NULL);
LettuceAssert.notEmpty(commands, "Commands " + MUST_NOT_BE_EMPTY);
LettuceAssert.noNullElements(commands, "Commands " + MUST_NOT_CONTAIN_NULL_ELEMENTS);
CommandArgs args = new CommandArgs(codec);
args.add(INFO);
for (String command : commands) {
args.add(command);
}
return createCommand(COMMAND, new ArrayOutput(codec), args);
}
public Command commandCount() {
CommandArgs args = new CommandArgs(codec).add(COUNT);
return createCommand(COMMAND, new IntegerOutput(codec), args);
}
public Command configRewrite() {
CommandArgs args = new CommandArgs(codec).add(REWRITE);
return createCommand(CONFIG, new StatusOutput(codec), args);
}
public Command> configGet(String parameter) {
LettuceAssert.notNull(parameter, "Parameter " + MUST_NOT_BE_NULL);
LettuceAssert.notEmpty(parameter, "Parameter " + MUST_NOT_BE_EMPTY);
CommandArgs args = new CommandArgs(codec).add(GET).add(parameter);
return createCommand(CONFIG, new StringListOutput(codec), args);
}
public Command configResetstat() {
CommandArgs args = new CommandArgs(codec).add(RESETSTAT);
return createCommand(CONFIG, new StatusOutput(codec), args);
}
public Command configSet(String parameter, String value) {
LettuceAssert.notNull(parameter, "Parameter " + MUST_NOT_BE_NULL);
LettuceAssert.notEmpty(parameter, "Parameter " + MUST_NOT_BE_EMPTY);
LettuceAssert.notNull(value, "Value " + MUST_NOT_BE_NULL);
CommandArgs args = new CommandArgs(codec).add(SET).add(parameter).add(value);
return createCommand(CONFIG, new StatusOutput(codec), args);
}
public Command dbsize() {
return createCommand(DBSIZE, new IntegerOutput(codec));
}
public Command debugCrashAndRecover(Long delay) {
CommandArgs args = new CommandArgs(codec).add("CRASH-AND-RECOVER");
if (delay != null) {
args.add(delay);
}
return createCommand(DEBUG, new StatusOutput(codec), args);
}
public Command debugObject(K key) {
notNullKey(key);
CommandArgs args = new CommandArgs(codec).add(OBJECT).addKey(key);
return createCommand(DEBUG, new StatusOutput(codec), args);
}
public Command debugOom() {
return createCommand(DEBUG, null, new CommandArgs(codec).add("OOM"));
}
public Command debugHtstats(int db) {
CommandArgs args = new CommandArgs(codec).add(HTSTATS).add(db);
return createCommand(DEBUG, new StatusOutput(codec), args);
}
public Command debugReload() {
return createCommand(DEBUG, new StatusOutput(codec), new CommandArgs(codec).add(RELOAD));
}
public Command debugRestart(Long delay) {
CommandArgs args = new CommandArgs(codec).add(RESTART);
if (delay != null) {
args.add(delay);
}
return createCommand(DEBUG, new StatusOutput(codec), args);
}
public Command debugSdslen(K key) {
notNullKey(key);
return createCommand(DEBUG, new StatusOutput(codec), new CommandArgs(codec).add("SDSLEN").addKey(key));
}
public Command debugSegfault() {
CommandArgs args = new CommandArgs(codec).add(SEGFAULT);
return createCommand(DEBUG, null, args);
}
public Command decr(K key) {
notNullKey(key);
return createCommand(DECR, new IntegerOutput(codec), key);
}
public Command decrby(K key, long amount) {
notNullKey(key);
CommandArgs args = new CommandArgs(codec).addKey(key).add(amount);
return createCommand(DECRBY, new IntegerOutput(codec), args);
}
public Command del(K... keys) {
notEmpty(keys);
CommandArgs args = new CommandArgs(codec).addKeys(keys);
return createCommand(DEL, new IntegerOutput(codec), args);
}
public Command del(Iterable keys) {
LettuceAssert.notNull(keys, "Keys " + MUST_NOT_BE_NULL);
CommandArgs args = new CommandArgs(codec).addKeys(keys);
return createCommand(DEL, new IntegerOutput(codec), args);
}
public Command unlink(K... keys) {
notEmpty(keys);
CommandArgs args = new CommandArgs(codec).addKeys(keys);
return createCommand(UNLINK, new IntegerOutput(codec), args);
}
public Command unlink(Iterable keys) {
LettuceAssert.notNull(keys, "Keys " + MUST_NOT_BE_NULL);
CommandArgs args = new CommandArgs(codec).addKeys(keys);
return createCommand(UNLINK, new IntegerOutput(codec), args);
}
public Command discard() {
return createCommand(DISCARD, new StatusOutput(codec));
}
public Command dump(K key) {
notNullKey(key);
CommandArgs args = new CommandArgs(codec).addKey(key);
return createCommand(DUMP, new ByteArrayOutput(codec), args);
}
public Command echo(V msg) {
LettuceAssert.notNull(msg, "message " + MUST_NOT_BE_NULL);
CommandArgs args = new CommandArgs(codec).addValue(msg);
return createCommand(ECHO, new ValueOutput(codec), args);
}
public Command eval(String script, ScriptOutputType type, K... keys) {
LettuceAssert.notNull(script, "Script " + MUST_NOT_BE_NULL);
LettuceAssert.notEmpty(script, "Script " + MUST_NOT_BE_EMPTY);
LettuceAssert.notNull(type, "ScriptOutputType " + MUST_NOT_BE_NULL);
LettuceAssert.notNull(keys, "Keys " + MUST_NOT_BE_NULL);
CommandArgs args = new CommandArgs(codec);
args.add(script).add(keys.length).addKeys(keys);
CommandOutput output = newScriptOutput(codec, type);
return createCommand(EVAL, output, args);
}
public Command eval(String script, ScriptOutputType type, K[] keys, V... values) {
LettuceAssert.notNull(script, "Script " + MUST_NOT_BE_NULL);
LettuceAssert.notEmpty(script, "Script " + MUST_NOT_BE_EMPTY);
LettuceAssert.notNull(type, "ScriptOutputType " + MUST_NOT_BE_NULL);
LettuceAssert.notNull(keys, "Keys " + MUST_NOT_BE_NULL);
LettuceAssert.notNull(values, "Values " + MUST_NOT_BE_NULL);
CommandArgs args = new CommandArgs(codec);
args.add(script).add(keys.length).addKeys(keys).addValues(values);
CommandOutput output = newScriptOutput(codec, type);
return createCommand(EVAL, output, args);
}
public Command evalsha(String digest, ScriptOutputType type, K... keys) {
LettuceAssert.notNull(digest, "Digest " + MUST_NOT_BE_NULL);
LettuceAssert.notEmpty(digest, "Digest " + MUST_NOT_BE_EMPTY);
LettuceAssert.notNull(type, "ScriptOutputType " + MUST_NOT_BE_NULL);
LettuceAssert.notNull(keys, "Keys " + MUST_NOT_BE_NULL);
CommandArgs args = new CommandArgs(codec);
args.add(digest).add(keys.length).addKeys(keys);
CommandOutput output = newScriptOutput(codec, type);
return createCommand(EVALSHA, output, args);
}
public Command evalsha(String digest, ScriptOutputType type, K[] keys, V... values) {
LettuceAssert.notNull(digest, "Digest " + MUST_NOT_BE_NULL);
LettuceAssert.notEmpty(digest, "Digest " + MUST_NOT_BE_EMPTY);
LettuceAssert.notNull(type, "ScriptOutputType " + MUST_NOT_BE_NULL);
LettuceAssert.notNull(keys, "Keys " + MUST_NOT_BE_NULL);
LettuceAssert.notNull(values, "Values " + MUST_NOT_BE_NULL);
CommandArgs args = new CommandArgs(codec);
args.add(digest).add(keys.length).addKeys(keys).addValues(values);
CommandOutput output = newScriptOutput(codec, type);
return createCommand(EVALSHA, output, args);
}
public Command exists(K key) {
notNullKey(key);
return createCommand(EXISTS, new BooleanOutput(codec), key);
}
public Command exists(K... keys) {
notEmpty(keys);
return createCommand(EXISTS, new IntegerOutput(codec), new CommandArgs(codec).addKeys(keys));
}
public Command exists(Iterable keys) {
LettuceAssert.notNull(keys, "Keys " + MUST_NOT_BE_NULL);
return createCommand(EXISTS, new IntegerOutput(codec), new CommandArgs(codec).addKeys(keys));
}
public Command expire(K key, long seconds) {
notNullKey(key);
CommandArgs args = new CommandArgs(codec).addKey(key).add(seconds);
return createCommand(EXPIRE, new BooleanOutput(codec), args);
}
public Command expireat(K key, long timestamp) {
notNullKey(key);
CommandArgs args = new CommandArgs(codec).addKey(key).add(timestamp);
return createCommand(EXPIREAT, new BooleanOutput(codec), args);
}
public Command flushall() {
return createCommand(FLUSHALL, new StatusOutput(codec));
}
public Command flushallAsync() {
return createCommand(FLUSHALL, new StatusOutput(codec), new CommandArgs(codec).add(ASYNC));
}
public Command flushdb() {
return createCommand(FLUSHDB, new StatusOutput(codec));
}
public Command flushdbAsync() {
return createCommand(FLUSHDB, new StatusOutput(codec), new CommandArgs(codec).add(ASYNC));
}
public Command get(K key) {
notNullKey(key);
return createCommand(GET, new ValueOutput(codec), key);
}
public Command getbit(K key, long offset) {
notNullKey(key);
CommandArgs args = new CommandArgs(codec).addKey(key).add(offset);
return createCommand(GETBIT, new IntegerOutput(codec), args);
}
public Command getrange(K key, long start, long end) {
notNullKey(key);
CommandArgs args = new CommandArgs(codec).addKey(key).add(start).add(end);
return createCommand(GETRANGE, new ValueOutput(codec), args);
}
public Command getset(K key, V value) {
notNullKey(key);
return createCommand(GETSET, new ValueOutput(codec), key, value);
}
public Command hdel(K key, K... fields) {
notNullKey(key);
LettuceAssert.notNull(fields, "Fields " + MUST_NOT_BE_NULL);
LettuceAssert.notEmpty(fields, "Fields " + MUST_NOT_BE_EMPTY);
CommandArgs args = new CommandArgs(codec).addKey(key).addKeys(fields);
return createCommand(HDEL, new IntegerOutput(codec), args);
}
public Command hexists(K key, K field) {
notNullKey(key);
LettuceAssert.notNull(field, "Field " + MUST_NOT_BE_NULL);
CommandArgs args = new CommandArgs(codec).addKey(key).addKey(field);
return createCommand(HEXISTS, new BooleanOutput(codec), args);
}
public Command hget(K key, K field) {
notNullKey(key);
LettuceAssert.notNull(field, "Field " + MUST_NOT_BE_NULL);
CommandArgs