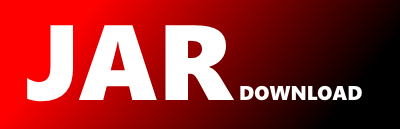
com.lambdaworks.redis.cluster.api.sync.NodeSelectionKeyCommands Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lettuce Show documentation
Show all versions of lettuce Show documentation
Advanced and thread-safe Java Redis client for synchronous, asynchronous, and
reactive usage. Supports Cluster, Sentinel, Pipelining, Auto-Reconnect, Codecs
and much more.
The newest version!
/*
* Copyright 2011-2016 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.lambdaworks.redis.cluster.api.sync;
import java.util.Date;
import java.util.List;
import com.lambdaworks.redis.Value;
import com.lambdaworks.redis.KeyScanCursor;
import com.lambdaworks.redis.MigrateArgs;
import com.lambdaworks.redis.ScanArgs;
import com.lambdaworks.redis.ScanCursor;
import com.lambdaworks.redis.SortArgs;
import com.lambdaworks.redis.StreamScanCursor;
import com.lambdaworks.redis.output.KeyStreamingChannel;
import com.lambdaworks.redis.output.ValueStreamingChannel;
/**
* Synchronous executed commands on a node selection for Keys (Key manipulation/querying).
*
* @param Key type.
* @param Value type.
* @author Mark Paluch
* @since 4.0
* @generated by com.lambdaworks.apigenerator.CreateSyncNodeSelectionClusterApi
*/
public interface NodeSelectionKeyCommands {
/**
* Delete one or more keys.
*
* @param keys the keys
* @return Long integer-reply The number of keys that were removed.
*/
Executions del(K... keys);
/**
* Unlink one or more keys (non blocking DEL).
*
* @param keys the keys
* @return Long integer-reply The number of keys that were removed.
*/
Executions unlink(K... keys);
/**
* Return a serialized version of the value stored at the specified key.
*
* @param key the key
* @return byte[] bulk-string-reply the serialized value.
*/
Executions dump(K key);
/**
* Determine how many keys exist.
*
* @param keys the keys
* @return Long integer-reply specifically: Number of existing keys
*/
Executions exists(K... keys);
/**
* Set a key's time to live in seconds.
*
* @param key the key
* @param seconds the seconds type: long
* @return Boolean integer-reply specifically:
*
* {@literal true} if the timeout was set. {@literal false} if {@code key} does not exist or the timeout could not
* be set.
*/
Executions expire(K key, long seconds);
/**
* Set the expiration for a key as a UNIX timestamp.
*
* @param key the key
* @param timestamp the timestamp type: posix time
* @return Boolean integer-reply specifically:
*
* {@literal true} if the timeout was set. {@literal false} if {@code key} does not exist or the timeout could not
* be set (see: {@code EXPIRE}).
*/
Executions expireat(K key, Date timestamp);
/**
* Set the expiration for a key as a UNIX timestamp.
*
* @param key the key
* @param timestamp the timestamp type: posix time
* @return Boolean integer-reply specifically:
*
* {@literal true} if the timeout was set. {@literal false} if {@code key} does not exist or the timeout could not
* be set (see: {@code EXPIRE}).
*/
Executions expireat(K key, long timestamp);
/**
* Find all keys matching the given pattern.
*
* @param pattern the pattern type: patternkey (pattern)
* @return List<K> array-reply list of keys matching {@code pattern}.
*/
Executions> keys(K pattern);
/**
* Find all keys matching the given pattern.
*
* @param channel the channel
* @param pattern the pattern
* @return Long array-reply list of keys matching {@code pattern}.
*/
Executions keys(KeyStreamingChannel channel, K pattern);
/**
* Atomically transfer a key from a Redis instance to another one.
*
* @param host the host
* @param port the port
* @param key the key
* @param db the database
* @param timeout the timeout in milliseconds
* @return String simple-string-reply The command returns OK on success.
*/
Executions migrate(String host, int port, K key, int db, long timeout);
/**
* Atomically transfer one or more keys from a Redis instance to another one.
*
* @param host the host
* @param port the port
* @param db the database
* @param timeout the timeout in milliseconds
* @param migrateArgs migrate args that allow to configure further options
* @return String simple-string-reply The command returns OK on success.
*/
Executions migrate(String host, int port, int db, long timeout, MigrateArgs migrateArgs);
/**
* Move a key to another database.
*
* @param key the key
* @param db the db type: long
* @return Boolean integer-reply specifically:
*/
Executions move(K key, int db);
/**
* returns the kind of internal representation used in order to store the value associated with a key.
*
* @param key the key
* @return String
*/
Executions objectEncoding(K key);
/**
* returns the number of seconds since the object stored at the specified key is idle (not requested by read or write
* operations).
*
* @param key the key
* @return number of seconds since the object stored at the specified key is idle.
*/
Executions objectIdletime(K key);
/**
* returns the number of references of the value associated with the specified key.
*
* @param key the key
* @return Long
*/
Executions objectRefcount(K key);
/**
* Remove the expiration from a key.
*
* @param key the key
* @return Boolean integer-reply specifically:
*
* {@literal true} if the timeout was removed. {@literal false} if {@code key} does not exist or does not have an
* associated timeout.
*/
Executions persist(K key);
/**
* Set a key's time to live in milliseconds.
*
* @param key the key
* @param milliseconds the milliseconds type: long
* @return integer-reply, specifically:
*
* {@literal true} if the timeout was set. {@literal false} if {@code key} does not exist or the timeout could not
* be set.
*/
Executions pexpire(K key, long milliseconds);
/**
* Set the expiration for a key as a UNIX timestamp specified in milliseconds.
*
* @param key the key
* @param timestamp the milliseconds-timestamp type: posix time
* @return Boolean integer-reply specifically:
*
* {@literal true} if the timeout was set. {@literal false} if {@code key} does not exist or the timeout could not
* be set (see: {@code EXPIRE}).
*/
Executions pexpireat(K key, Date timestamp);
/**
* Set the expiration for a key as a UNIX timestamp specified in milliseconds.
*
* @param key the key
* @param timestamp the milliseconds-timestamp type: posix time
* @return Boolean integer-reply specifically:
*
* {@literal true} if the timeout was set. {@literal false} if {@code key} does not exist or the timeout could not
* be set (see: {@code EXPIRE}).
*/
Executions pexpireat(K key, long timestamp);
/**
* Get the time to live for a key in milliseconds.
*
* @param key the key
* @return Long integer-reply TTL in milliseconds, or a negative value in order to signal an error (see the description
* above).
*/
Executions pttl(K key);
/**
* Return a random key from the keyspace.
*
* @return V bulk-string-reply the random key, or {@literal null} when the database is empty.
*/
Executions randomkey();
/**
* Rename a key.
*
* @param key the key
* @param newKey the newkey type: key
* @return String simple-string-reply
*/
Executions rename(K key, K newKey);
/**
* Rename a key, only if the new key does not exist.
*
* @param key the key
* @param newKey the newkey type: key
* @return Boolean integer-reply specifically:
*
* {@literal true} if {@code key} was renamed to {@code newkey}. {@literal false} if {@code newkey} already exists.
*/
Executions renamenx(K key, K newKey);
/**
* Create a key using the provided serialized value, previously obtained using DUMP.
*
* @param key the key
* @param ttl the ttl type: long
* @param value the serialized-value type: string
* @return String simple-string-reply The command returns OK on success.
*/
Executions restore(K key, long ttl, byte[] value);
/**
* Sort the elements in a list, set or sorted set.
*
* @param key the key
* @return List<V> array-reply list of sorted elements.
*/
Executions> sort(K key);
/**
* Sort the elements in a list, set or sorted set.
*
* @param channel streaming channel that receives a call for every value
* @param key the key
* @return Long number of values.
*/
Executions sort(ValueStreamingChannel channel, K key);
/**
* Sort the elements in a list, set or sorted set.
*
* @param key the key
* @param sortArgs sort arguments
* @return List<V> array-reply list of sorted elements.
*/
Executions> sort(K key, SortArgs sortArgs);
/**
* Sort the elements in a list, set or sorted set.
*
* @param channel streaming channel that receives a call for every value
* @param key the key
* @param sortArgs sort arguments
* @return Long number of values.
*/
Executions sort(ValueStreamingChannel channel, K key, SortArgs sortArgs);
/**
* Sort the elements in a list, set or sorted set.
*
* @param key the key
* @param sortArgs sort arguments
* @param destination the destination key to store sort results
* @return Long number of values.
*/
Executions sortStore(K key, SortArgs sortArgs, K destination);
/**
* Touch one or more keys. Touch sets the last accessed time for a key. Non-exsitent keys wont get created.
*
* @param keys the keys
* @return Long integer-reply the number of found keys.
*/
Executions touch(K... keys);
/**
* Get the time to live for a key.
*
* @param key the key
* @return Long integer-reply TTL in seconds, or a negative value in order to signal an error (see the description above).
*/
Executions ttl(K key);
/**
* Determine the type stored at key.
*
* @param key the key
* @return String simple-string-reply type of {@code key}, or {@code none} when {@code key} does not exist.
*/
Executions type(K key);
/**
* Incrementally iterate the keys space.
*
* @return KeyScanCursor<K> scan cursor.
*/
Executions> scan();
/**
* Incrementally iterate the keys space.
*
* @param scanArgs scan arguments
* @return KeyScanCursor<K> scan cursor.
*/
Executions> scan(ScanArgs scanArgs);
/**
* Incrementally iterate the keys space.
*
* @param scanCursor cursor to resume from a previous scan, must not be {@literal null}
* @param scanArgs scan arguments
* @return KeyScanCursor<K> scan cursor.
*/
Executions> scan(ScanCursor scanCursor, ScanArgs scanArgs);
/**
* Incrementally iterate the keys space.
*
* @param scanCursor cursor to resume from a previous scan, must not be {@literal null}
* @return KeyScanCursor<K> scan cursor.
*/
Executions> scan(ScanCursor scanCursor);
/**
* Incrementally iterate the keys space.
*
* @param channel streaming channel that receives a call for every key
* @return StreamScanCursor scan cursor.
*/
Executions scan(KeyStreamingChannel channel);
/**
* Incrementally iterate the keys space.
*
* @param channel streaming channel that receives a call for every key
* @param scanArgs scan arguments
* @return StreamScanCursor scan cursor.
*/
Executions scan(KeyStreamingChannel channel, ScanArgs scanArgs);
/**
* Incrementally iterate the keys space.
*
* @param channel streaming channel that receives a call for every key
* @param scanCursor cursor to resume from a previous scan, must not be {@literal null}
* @param scanArgs scan arguments
* @return StreamScanCursor scan cursor.
*/
Executions scan(KeyStreamingChannel channel, ScanCursor scanCursor, ScanArgs scanArgs);
/**
* Incrementally iterate the keys space.
*
* @param channel streaming channel that receives a call for every key
* @param scanCursor cursor to resume from a previous scan, must not be {@literal null}
* @return StreamScanCursor scan cursor.
*/
Executions scan(KeyStreamingChannel channel, ScanCursor scanCursor);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy