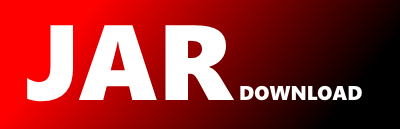
com.lambdaworks.redis.dynamic.codec.AnnotationRedisCodecResolver Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lettuce Show documentation
Show all versions of lettuce Show documentation
Advanced and thread-safe Java Redis client for synchronous, asynchronous, and
reactive usage. Supports Cluster, Sentinel, Pipelining, Auto-Reconnect, Codecs
and much more.
The newest version!
/*
* Copyright 2011-2016 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.lambdaworks.redis.dynamic.codec;
import java.util.Collections;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
import java.util.stream.Collectors;
import com.lambdaworks.redis.dynamic.annotation.Key;
import com.lambdaworks.redis.dynamic.annotation.Value;
import com.lambdaworks.redis.codec.RedisCodec;
import com.lambdaworks.redis.dynamic.CommandMethod;
import com.lambdaworks.redis.dynamic.parameter.Parameter;
import com.lambdaworks.redis.dynamic.support.ClassTypeInformation;
import com.lambdaworks.redis.dynamic.support.TypeInformation;
import com.lambdaworks.redis.internal.LettuceAssert;
import com.lambdaworks.redis.internal.LettuceLists;
/**
* Annotation-based {@link RedisCodecResolver}. Considers {@code @Key} and {@code @Value} annotations of method parameters to
* determine a {@link RedisCodec} that is able to handle all involved types.
*
* @author Mark Paluch
* @since 5.0
* @see Key
* @see Value
*/
public class AnnotationRedisCodecResolver implements RedisCodecResolver {
private final List> codecs;
/**
* Creates a new {@link AnnotationRedisCodecResolver} given a {@link List} of {@link RedisCodec}s.
*
* @param codecs must not be {@literal null}.
*/
public AnnotationRedisCodecResolver(List> codecs) {
LettuceAssert.notNull(codecs, "List of RedisCodecs must not be null");
this.codecs = LettuceLists.unmodifiableList(codecs);
}
@Override
public RedisCodec, ?> resolve(CommandMethod commandMethod) {
LettuceAssert.notNull(commandMethod, "CommandMethod must not be null");
Set> keyTypes = findTypes(commandMethod, Key.class);
Set> valueTypes = findTypes(commandMethod, Value.class);
if (keyTypes.isEmpty() && valueTypes.isEmpty()) {
Voted> voted = voteForTypeMajority(commandMethod);
if (voted != null) {
return voted.subject;
}
return codecs.get(0);
}
if ((keyTypes.size() == 1 && (valueTypes.isEmpty() || valueTypes.size() == 1))
|| (valueTypes.size() == 1 && (keyTypes.isEmpty() || keyTypes.size() == 1))) {
RedisCodec, ?> codec = resolveCodec(keyTypes, valueTypes);
if (codec != null) {
return codec;
}
}
throw new IllegalStateException(String.format("Cannot resolve Codec for method %s", commandMethod.getMethod()));
}
private Voted> voteForTypeMajority(CommandMethod commandMethod) {
List>> votes = codecs.stream().map(redisCodec -> new Voted>(redisCodec, 0))
.collect(Collectors.toList());
commandMethod.getParameters().getBindableParameters().forEach(parameter -> {
for (Voted> vote : votes) {
ClassTypeInformation extends RedisCodec> typeInformation = ClassTypeInformation.from(vote.subject.getClass());
TypeInformation> superTypeInformation = typeInformation.getSuperTypeInformation(RedisCodec.class);
List> typeArguments = superTypeInformation.getTypeArguments();
if (typeArguments.size() != 2) {
continue;
}
TypeInformation> keyType = typeArguments.get(0);
TypeInformation> valueType = typeArguments.get(1);
if (keyType.isAssignableFrom(parameter.getTypeInformation())) {
vote.votes++;
}
if (valueType.isAssignableFrom(parameter.getTypeInformation())) {
vote.votes++;
}
}
});
Collections.sort(votes);
if (votes.isEmpty()) {
return null;
}
Voted> voted = votes.get(votes.size() - 1);
if (voted.votes == 0) {
return null;
}
return voted;
}
private RedisCodec, ?> resolveCodec(Set> keyTypes, Set> valueTypes) {
Class> keyType = keyTypes.isEmpty() ? null : keyTypes.iterator().next();
Class> valueType = valueTypes.isEmpty() ? null : valueTypes.iterator().next();
for (RedisCodec, ?> codec : codecs) {
ClassTypeInformation> typeInformation = ClassTypeInformation.from(codec.getClass());
TypeInformation> keyTypeArgument = typeInformation.getTypeArgument(RedisCodec.class, 0);
TypeInformation> valueTypeArgument = typeInformation.getTypeArgument(RedisCodec.class, 1);
if (keyTypeArgument == null || valueTypeArgument == null) {
continue;
}
boolean keyMatch = false;
boolean valueMatch = false;
if (keyType != null) {
keyMatch = keyTypeArgument.isAssignableFrom(ClassTypeInformation.from(keyType));
}
if (valueType != null) {
valueMatch = valueTypeArgument.isAssignableFrom(ClassTypeInformation.from(valueType));
}
if (keyType != null && valueType != null && keyMatch && valueMatch) {
return codec;
}
if (keyType != null && valueType == null && keyMatch) {
return codec;
}
if (keyType == null && valueType != null && valueMatch) {
return codec;
}
}
return null;
}
private Set> findTypes(CommandMethod commandMethod, Class> annotation) {
Set> types = new HashSet<>();
for (Parameter parameter : commandMethod.getParameters().getBindableParameters()) {
types.addAll(parameter.getAnnotations().stream()
.filter(parameterAnnotation -> annotation.isAssignableFrom(parameterAnnotation.getClass()))
.map(parameterAnnotation -> {
if (parameter.getTypeInformation().isCollectionLike()
&& !parameter.getTypeInformation().getType().isArray()) {
return parameter.getTypeInformation().getComponentType().getType();
}
return parameter.getTypeInformation().getType();
}).collect(Collectors.toList()));
}
return types;
}
private static class Voted implements Comparable> {
private T subject;
private int votes;
public Voted(T subject, int votes) {
this.subject = subject;
this.votes = votes;
}
@Override
public int compareTo(Voted> o) {
return votes - o.votes;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy