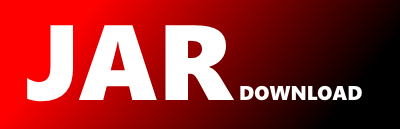
com.lambdaworks.redis.protocol.Command Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lettuce Show documentation
Show all versions of lettuce Show documentation
Advanced and thread-safe Java Redis client for synchronous, asynchronous, and
reactive usage. Supports Cluster, Sentinel, Pipelining, Auto-Reconnect, Codecs
and much more.
The newest version!
/*
* Copyright 2011-2016 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.lambdaworks.redis.protocol;
import com.lambdaworks.redis.internal.LettuceAssert;
import com.lambdaworks.redis.output.CommandOutput;
import io.netty.buffer.ByteBuf;
/**
* A Redis command with a {@link ProtocolKeyword command type}, {@link CommandArgs arguments} and an optional {@link CommandOutput
* output}. All successfully executed commands will eventually return a {@link CommandOutput} object.
*
* @param Key type.
* @param Value type.
* @param Command output type.
*
* @author Will Glozer
* @author Mark Paluch
*/
public class Command implements RedisCommand, WithLatency {
private final ProtocolKeyword type;
protected CommandArgs args;
protected CommandOutput output;
protected Throwable exception;
protected boolean cancelled = false;
protected boolean completed = false;
protected long sentNs = -1;
protected long firstResponseNs = -1;
protected long completedNs = -1;
/**
* Create a new command with the supplied type.
*
* @param type Command type, must not be {@literal null}.
* @param output Command output, can be {@literal null}.
*/
public Command(ProtocolKeyword type, CommandOutput output) {
this(type, output, null);
}
/**
* Create a new command with the supplied type and args.
*
* @param type Command type, must not be {@literal null}.
* @param output Command output, can be {@literal null}.
* @param args Command args, can be {@literal null}
*/
public Command(ProtocolKeyword type, CommandOutput output, CommandArgs args) {
LettuceAssert.notNull(type, "Command type must not be null");
this.type = type;
this.output = output;
this.args = args;
}
/**
* Get the object that holds this command's output.
*
* @return The command output object.
*/
@Override
public CommandOutput getOutput() {
return output;
}
@Override
public boolean completeExceptionally(Throwable throwable) {
if (output != null) {
output.setError(throwable.getMessage());
}
exception = throwable;
return true;
}
/**
* Mark this command complete and notify all waiting threads.
*/
@Override
public void complete() {
completed = true;
}
@Override
public void cancel() {
cancelled = true;
}
/**
* Encode and write this command to the supplied buffer using the new Unified
* Request Protocol.
*
* @param buf Buffer to write to.
*/
public void encode(ByteBuf buf) {
buf.writeByte('*');
CommandArgs.IntegerArgument.writeInteger(buf, 1 + (args != null ? args.count() : 0));
buf.writeBytes(CommandArgs.CRLF);
CommandArgs.BytesArgument.writeBytes(buf, type.getBytes());
if (args != null) {
args.encode(buf);
}
}
public String getError() {
return output.getError();
}
@Override
public CommandArgs getArgs() {
return args;
}
/**
*
* @return the resut from the output.
*/
public T get() {
if (output != null) {
return output.get();
}
return null;
}
@Override
public String toString() {
final StringBuilder sb = new StringBuilder();
sb.append(getClass().getSimpleName());
sb.append(" [type=").append(type);
sb.append(", output=").append(output);
sb.append(']');
return sb.toString();
}
public void setOutput(CommandOutput output) {
if (isCancelled() || completed) {
throw new IllegalStateException("Command is completed/cancelled. Cannot set a new output");
}
this.output = output;
}
@Override
public ProtocolKeyword getType() {
return type;
}
@Override
public boolean isCancelled() {
return cancelled;
}
@Override
public boolean isDone() {
return completed;
}
@Override
public void sent(long timeNs) {
sentNs = timeNs;
firstResponseNs = -1;
completedNs = -1;
}
@Override
public void firstResponse(long timeNs) {
firstResponseNs = timeNs;
}
@Override
public void completed(long timeNs) {
completedNs = timeNs;
}
@Override
public long getSent() {
return sentNs;
}
@Override
public long getFirstResponse() {
return firstResponseNs;
}
@Override
public long getCompleted() {
return completedNs;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy