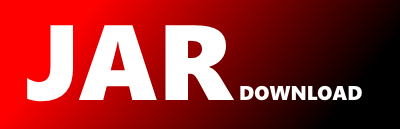
com.lambdaworks.redis.resource.Futures Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lettuce Show documentation
Show all versions of lettuce Show documentation
Advanced and thread-safe Java Redis client for synchronous, asynchronous, and
reactive usage. Supports Cluster, Sentinel, Pipelining, Auto-Reconnect, Codecs
and much more.
The newest version!
/*
* Copyright 2011-2016 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.lambdaworks.redis.resource;
import java.util.LinkedHashSet;
import java.util.Set;
import java.util.concurrent.atomic.AtomicInteger;
import com.lambdaworks.redis.internal.LettuceAssert;
import io.netty.util.concurrent.*;
/**
* Utility class to support netty's future handling.
*
* @author Mark Paluch
* @since 3.4
*/
class Futures {
/**
* Create a promise that emits a {@code Boolean} value on completion of the {@code future}
*
* @param future the future.
* @return Promise emitting a {@code Boolean} value. {@literal true} if the {@code future} completed successfully, otherwise
* the cause wil be transported.
*/
static Promise toBooleanPromise(Future> future) {
final DefaultPromise result = new DefaultPromise(GlobalEventExecutor.INSTANCE);
future.addListener(new GenericFutureListener>() {
@Override
public void operationComplete(Future
© 2015 - 2025 Weber Informatics LLC | Privacy Policy