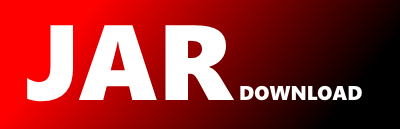
biz.paluch.spinach.api.async.DisqueQueueAsyncCommands Maven / Gradle / Ivy
package biz.paluch.spinach.api.async;
import java.util.List;
import biz.paluch.spinach.api.Job;
import biz.paluch.spinach.api.QScanArgs;
import com.lambdaworks.redis.KeyScanCursor;
import com.lambdaworks.redis.RedisFuture;
import com.lambdaworks.redis.ScanCursor;
/**
* Asynchronous executed commands related with Disque Queues.
*
* @param Key type.
* @param Value type.
* @author Mark Paluch
*/
public interface DisqueQueueAsyncCommands {
/**
* Queue jobs if not already queued.
*
* @param jobIds the job Id's
* @return the number of jobs actually move from active to queued state
*/
RedisFuture enqueue(String... jobIds);
/**
* Queue jobs if not already queued and increment the nack counter.
*
* @param jobIds the job Id's
* @return the number of jobs actually move from active to queued state
*/
RedisFuture nack(String... jobIds);
/**
* Remove the job from the queue.
*
* @param jobIds the job Id's
* @return the number of jobs actually moved from queue to active state
*/
RedisFuture dequeue(String... jobIds);
/**
* If the job is queued, remove it from queue and change state to active. Postpone the job requeue time in the future so
* that we'll wait the retry time before enqueueing again.
*
* * Return how much time the worker likely have before the next requeue event or an error:
*
* - -ACKED: The job is already acknowledged, so was processed already.
* - -NOJOB We don't know about this job. The job was either already acknowledged and purged, or this node never received
* a copy.
* - -TOOLATE 50% of the job TTL already elapsed, is no longer possible to delay it.
*
*
* @param jobId the job Id
* @return retry count.
*/
RedisFuture working(String jobId);
/**
* Return the number of jobs queued.
*
* @param queue the queue
* @return the number of jobs queued
*/
RedisFuture qlen(K queue);
/**
* Return an array of at most "count" jobs available inside the queue "queue" without removing the jobs from the queue. This
* is basically an introspection and debugging command.
*
* @param queue the queue
* @param count number of jobs to return
* @return List of jobs.
*/
RedisFuture>> qpeek(K queue, long count);
/**
* Incrementally iterate the keys space.
*
* @return KeyScanCursor<K> scan cursor.
*/
RedisFuture> qscan();
/**
* Incrementally iterate the keys space.
*
* @param scanArgs scan arguments
* @return KeyScanCursor<K> scan cursor.
*/
RedisFuture> qscan(QScanArgs scanArgs);
/**
* Incrementally iterate the keys space.
*
* @param scanCursor cursor to resume from a previous scan
* @param scanArgs scan arguments
* @return KeyScanCursor<K> scan cursor.
*/
RedisFuture> qscan(ScanCursor scanCursor, QScanArgs scanArgs);
/**
* Incrementally iterate the keys space.
*
* @param scanCursor cursor to resume from a previous scan
* @return KeyScanCursor<K> scan cursor.
*/
RedisFuture> qscan(ScanCursor scanCursor);
}