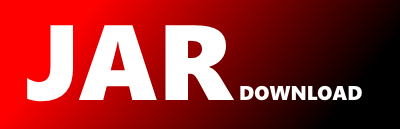
br.com.anteros.jsondoc.springmvc.scanner.builder.SpringHeaderBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of Anteros-JSONDoc-SpringMVC Show documentation
Show all versions of Anteros-JSONDoc-SpringMVC Show documentation
The support for Spring MVC of the jsondoc project.
The newest version!
package br.com.anteros.jsondoc.springmvc.scanner.builder;
import java.lang.annotation.Annotation;
import java.lang.reflect.Method;
import java.util.Arrays;
import java.util.LinkedHashSet;
import java.util.List;
import java.util.Set;
import org.springframework.web.bind.annotation.RequestHeader;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.ValueConstants;
import br.com.anteros.jsondoc.core.pojo.ApiHeaderDoc;
public class SpringHeaderBuilder {
/**
* From Spring's documentation: Supported at the type level as well as at
* the method level! When used at the type level, all method-level mappings
* inherit this header restriction. Finally it looks for @ApiHeader
* annotations on parameters and adds the result to the final Set
*
* @param method
* @param controller
* @return
*/
public static Set buildHeaders(Method method) {
Set headers = new LinkedHashSet();
Class> controller = method.getDeclaringClass();
RequestMapping typeAnnotation = controller.getAnnotation(RequestMapping.class);
RequestMapping methodAnnotation = method.getAnnotation(RequestMapping.class);
if (typeAnnotation != null) {
List headersStringList = Arrays.asList(typeAnnotation.headers());
addToHeaders(headers, headersStringList);
}
if (methodAnnotation != null) {
List headersStringList = Arrays.asList(methodAnnotation.headers());
addToHeaders(headers, headersStringList);
}
Annotation[][] parametersAnnotations = method.getParameterAnnotations();
for (int i = 0; i < parametersAnnotations.length; i++) {
for (int j = 0; j < parametersAnnotations[i].length; j++) {
if (parametersAnnotations[i][j] instanceof RequestHeader) {
RequestHeader requestHeader = (RequestHeader) parametersAnnotations[i][j];
headers.add(new ApiHeaderDoc(requestHeader.value(), "", requestHeader.defaultValue().equals(ValueConstants.DEFAULT_NONE) ? new String[] {} : new String[] { requestHeader.defaultValue() }));
}
}
}
return headers;
}
private static void addToHeaders(Set headers, List headersStringList) {
for (String header : headersStringList) {
String[] splitHeader = header.split("=");
if (splitHeader.length > 1) {
headers.add(new ApiHeaderDoc(splitHeader[0], null, new String[] { splitHeader[1] }));
} else {
headers.add(new ApiHeaderDoc(splitHeader[0], null, new String[] {}));
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy