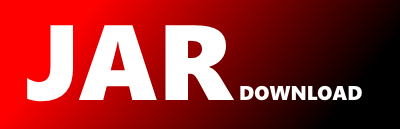
br.com.anteros.nosql.persistence.mongodb.query.UpdateResults Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of Anteros-NoSql-Persistence-MongoDB Show documentation
Show all versions of Anteros-NoSql-Persistence-MongoDB Show documentation
Anteros NoSQL Persistence MongoDB.
package br.com.anteros.nosql.persistence.mongodb.query;
import static java.lang.String.format;
import com.mongodb.WriteResult;
/**
* This class holds various metrics about the results of an update operation.
*/
public class UpdateResults {
private final WriteResult wr;
/**
* Creates an UpdateResults
*
* @param wr the WriteResult from the driver.
*/
public UpdateResults(final WriteResult wr) {
this.wr = wr;
}
/**
* @return number inserted; this should be either 0/1.
*/
public int getInsertedCount() {
return !getUpdatedExisting() ? getN() : 0;
}
/**
* @return the new _id field if an insert/upsert was performed
*/
public Object getNewId() {
return wr.getUpsertedId();
}
/**
* @return number updated
*/
public int getUpdatedCount() {
return getUpdatedExisting() ? getN() : 0;
}
/**
* @return true if updated, false if inserted or none effected
*/
public boolean getUpdatedExisting() {
return wr.isUpdateOfExisting();
}
/**
* @return the underlying data
*/
public WriteResult getWriteResult() {
return wr;
}
/**
* @return number of affected documents
*/
protected int getN() {
return wr.getN();
}
@Override
public String toString() {
return format("UpdateResults{wr=%s}", wr);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy