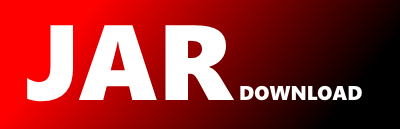
br.com.behaviortest.api.common.LogExecution Maven / Gradle / Ivy
package br.com.behaviortest.api.common;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import br.com.behaviortest.api.engine.holder.ExecutionHolder;
import br.com.behaviortest.model.design.Script;
import br.com.behaviortest.model.dto.Browser;
import br.com.behaviortest.model.type.Evaluation;
import br.com.behaviortest.util.LogUtil;
import br.com.behaviortest.util.ObjectUtil;
import br.com.behaviortest.util.StringUtil;
/**
* @author Felipe Rudolfe
* @since 9 de jan de 2020
*/
public class LogExecution {
private LogExecution() {}
public static void start() {
banner();
startExecution();
}
public static void showScriptHeader(String id, String name, String suiteId, String suiteName) {
nextline(1);
ponto();
dataSuite(suiteId, suiteName);
dataScript(id, name);
pontoWithStart();
}
public static void dataScript(String id, String name) {
LogUtil.log(Const.SCRIPT_DATA, id, name);
}
public static void dataSuite(String id, String name) {
if (ObjectUtil.isNotNullOrEmpty(id)) {
LogUtil.log(Const.SUITE_DATA, id, name);
}
}
public static void colletedSteps() {
LogUtil.log(Const.COLLECTED_STEPS);
}
public static void executedSteps() {
nextlineWithStart(1);
LogUtil.log(Const.EXECUTED_STEPS);
}
public static void preconditions() {
LogUtil.log(Const.PRECONDITIONS);
}
public static void procedures() {
LogUtil.log(Const.PROCEDURES);
}
public static void postconditions() {
LogUtil.log(Const.POSTCONDITIONS);
}
public static void showSteps(List steps) {
if (steps.isEmpty()) {
LogUtil.log("| - // -");
} else {
for (int i = 0; i < steps.size(); i++) {
LogUtil.log(Const.STEP, Const.ITEM_CHAR, steps.get(i));
}
}
}
public static void showStep(String step) {
if (ExecutionHolder.isCollectionMode()) {
LogUtil.log(Const.STEP, Const.ITEM_CHAR, step);
} else if (ExecutionHolder.getThrowable() != null) {
LogUtil.log(Const.STEP, Const.FAILURE_CHAR, step);
} else {
LogUtil.log(Const.STEP, Const.SUCCESS_CHAR, step);
}
}
public static void showEvaluation(Evaluation evaluation, Throwable e) {
LogUtil.log(Const.EVALUATION, evaluation);
if (ObjectUtil.isNotNull(e)) {
String msg = (e.getMessage() != null) ? e.getMessage() : e.getCause().getMessage();
msg = msg.replace("\n", "\n| ");
LogUtil.log(Const.MOTIVE, msg);
}
pontoWithStart();
}
public static void nextline(int qtd) {
int i = 0;
while (i < qtd) {
LogUtil.log(Const.NEXTLINE);
i++;
}
}
public static void nextlineWithStart(int qtd) {
int i = 0;
while (i < qtd) {
LogUtil.log("|" + Const.NEXTLINE);
i++;
}
}
public static void banner() {
LogUtil.log(Const.BANNER);
}
public static void startExecution() {
barra();
LogUtil.log(Const.START_EXECUTION);
barra();
}
public static void testClassFound() {
nextline(1);
LogUtil.log(Const.LOAD_TEST_EXECUTION);
traco();
}
public static void params() {
nextline(1);
LogUtil.log(Const.PARAMS);
traco();
}
public static void browsersValidate() {
nextline(1);
LogUtil.log(Const.VALIDATE_BROWSERS);
traco();
}
public static void testValidate() {
nextline(1);
LogUtil.log(Const.VALIDATE_TEST_IMPLEMENT);
traco();
}
public static void testValidated() {
LogUtil.log(Const.VALIDATED_TEST);
}
public static void runScripts() {
nextline(1);
LogUtil.log(Const.RUN_SCRIPTS);
traco();
}
public static void barra() {
LogUtil.log(Const.BARRA);
}
public static void ponto() {
LogUtil.log(Const.PONTO);
}
public static void pontoWithStart() {
LogUtil.log(Const.PONTO.replaceFirst(".", "|"));
}
public static void traco() {
LogUtil.log(Const.TRACO);
}
public static void loadclasss(Map> mapTest) {
testClassFound();
for (Entry> entry : mapTest.entrySet()) {
Class clazz = entry.getValue();
LogUtil.log(clazz.getName());
}
}
public static void params(String [] params) {
params();
for (String param : params) {
LogUtil.log(param);
}
}
public static void browser(Browser browser) {
LogUtil.log(" " + StringUtil.toJson(browser));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy