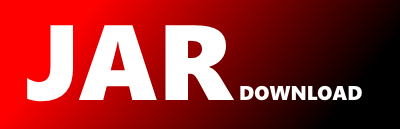
br.com.esec.icpm.libs.signature.ApplicationProviderClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of certillion-client-library Show documentation
Show all versions of certillion-client-library Show documentation
This library is used to make integration with Certillion server, so our Clients can easily ask for signatures or generate certificates.
package br.com.esec.icpm.libs.signature;
import java.io.InputStream;
import javax.ws.rs.Path;
import javax.ws.rs.client.Entity;
import javax.ws.rs.client.WebTarget;
import javax.ws.rs.core.MediaType;
import javax.ws.rs.core.Response;
import javax.ws.rs.core.Response.Status.Family;
import br.com.esec.icpm.mss.rest.ApplicationProviderResource;
public class ApplicationProviderClient implements ApplicationProviderResource {
private WebTarget target;
public ApplicationProviderClient(WebTarget target) {
this.target = target;
}
@Override
public void downloadSignedDocument(long transactionId) {
throw new UnsupportedOperationException("Nao implementado nesse proxy client.");
}
public InputStream downloadSignedDocumentCustomized(long transactionId) {
try {
String basePath = ApplicationProviderResource.class.getAnnotation(Path.class).value();
String extraPath = ApplicationProviderResource.class.getDeclaredMethod("downloadSignedDocument", Long.TYPE).getAnnotation(Path.class).value();
String uri = basePath + extraPath;
Response response = target.path(uri).resolveTemplate("transactionId", transactionId).request().get();
if (response.getStatusInfo().getFamily() == Family.SUCCESSFUL) {
return response.readEntity(InputStream.class);
} else {
throw new IllegalStateException("Cannot upload document. Certillion server anwser with status '" + response.getStatusInfo().getReasonPhrase() + " (" + response.getStatusInfo().getStatusCode() + ")'");
}
} catch (NoSuchMethodException e) {
throw new IllegalStateException("Method downloadSignedDocument cannot be found.", e);
} catch (SecurityException e) {
throw new IllegalStateException("Method downloadSignedDocument cannot be access.", e);
}
}
@Override
public String oldUpload(InputStream document) {
throw new UnsupportedOperationException("Nao implementado nesse proxy client.");
}
@Override
public String uploadDocument(InputStream document) {
try {
String basePath = ApplicationProviderResource.class.getAnnotation(Path.class).value();
String extraPath = ApplicationProviderResource.class.getDeclaredMethod("uploadDocument", InputStream.class).getAnnotation(Path.class).value();
String uri = basePath + extraPath;
Response response = target
.path(uri)
.request(MediaType.TEXT_PLAIN)
.post(Entity.entity(document, MediaType.APPLICATION_OCTET_STREAM));
if (response.getStatusInfo().getFamily() == Family.SUCCESSFUL) {
return response.readEntity(String.class);
} else {
throw new IllegalStateException("Cannot upload document. Certillion server anwser with status '" + response.getStatusInfo().getReasonPhrase() + " (" + response.getStatusInfo().getStatusCode() + ")'");
}
} catch (NoSuchMethodException e) {
throw new IllegalStateException("Method uploadDocument cannot be found.", e);
} catch (SecurityException e) {
throw new IllegalStateException("Method uploadDocument cannot be access.", e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy