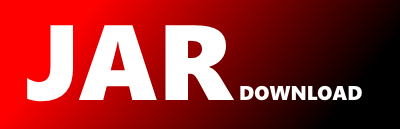
br.com.esec.icpm.libs.signature.BatchSignatureRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of certillion-client-library Show documentation
Show all versions of certillion-client-library Show documentation
This library is used to make integration with Certillion server, so our Clients can easily ask for signatures or generate certificates.
package br.com.esec.icpm.libs.signature;
import java.io.IOException;
import java.io.InputStream;
import java.util.ArrayList;
import java.util.List;
import java.util.concurrent.TimeoutException;
import org.apache.commons.io.FilenameUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import br.com.esec.icpm.libs.signature.helper.MimeTypeConstants;
import br.com.esec.icpm.libs.signature.helper.RequestBatchSignatureHelper;
import br.com.esec.icpm.libs.signature.response.handler.batch.SignatureBatchAsynchHandler;
import br.com.esec.icpm.libs.signature.response.handler.batch.SignatureBatchAsynchWithNotifyHandler;
import br.com.esec.icpm.libs.signature.response.handler.batch.SignatureBatchSynchHandler;
import br.com.esec.icpm.mss.ws.BatchSignatureComplexDocumentRespType;
import br.com.esec.icpm.mss.ws.SignatureStandardType;
import br.com.esec.icpm.server.ws.ICPMException;
public class BatchSignatureRequest {
private static Logger log = LoggerFactory.getLogger(BatchSignatureRequest.class);
private SignatureRequest request;
private SignatureStandardType standard = SignatureStandardType.CADES;
private String message;
private List documents = new ArrayList();
public BatchSignatureRequest(SignatureRequest request) {
this.request = request;
}
public BatchSignatureRequest cades() {
standard = SignatureStandardType.CADES;
return this;
}
public BatchSignatureRequest xades() {
standard = SignatureStandardType.XADES;
return this;
}
public BatchSignatureRequest adobePdf() {
standard = SignatureStandardType.ADOBEPDF;
return this;
}
public BatchSignatureRequest message(String message) {
this.message = message;
return this;
}
public BatchSignatureRequest document(String name, InputStream in) {
this.documents.add(new Document(name, in));
log.info("Document '" + name + "' added.");
return this;
}
public BatchSignatureRequest document(String name, InputStream in, String mymeType) {
this.documents.add(new Document(name, in, mymeType));
log.info("Document '" + name + "' added.");
return this;
}
public SignatureBatchSynchHandler sign() throws ICPMException, IOException {
log.info("Requesting asynch batch signature to '" + request.identifier + "' with standard '" + standard + "' and policy type '" + request.policyType + "'...");
BatchSignatureComplexDocumentRespType response = RequestBatchSignatureHelper.requestSynchBatchSignature(request.server, request.identifier, message, standard,
request.policyType, request.testMode, request.options.certificateFilters, documents, request.apId);
return new SignatureBatchSynchHandler(request.server, standard, response);
}
public SignatureBatchAsynchHandler asynchSign() throws ICPMException, IOException {
log.info("Requesting asynch batch signature to '" + request.identifier + "' with standard '" + standard + "' and policy type '" + request.policyType + "'...");
BatchSignatureComplexDocumentRespType response = RequestBatchSignatureHelper.requestAsynchBatchSignature(request.server, request.identifier, message, standard,
request.policyType, request.testMode, request.options.certificateFilters, documents, request.apId);
return new SignatureBatchAsynchHandler(request.server, standard, response);
}
public SignatureBatchAsynchWithNotifyHandler asynchSignWithNotify() throws ICPMException, IOException {
log.info("Requesting asynch batch signature to '" + request.identifier + "' with standard '" + standard + "' and policy type '" + request.policyType + "'...");
BatchSignatureComplexDocumentRespType response = RequestBatchSignatureHelper.requestAsynchWithNotifyBatchSignature(request.server, request.identifier, message, standard,
request.policyType, request.testMode, request.options.certificateFilters, documents, request.apId);
return new SignatureBatchAsynchWithNotifyHandler(request.server, standard, response);
}
public SignatureBatchAsynchHandler waitTo(long transactionId) throws ICPMException, InterruptedException, TimeoutException {
return new SignatureBatchAsynchHandler(request.server, standard, transactionId).waitTo();
}
public class Document {
private final String name;
private final InputStream in;
private final String mimeType;
public Document(String name, InputStream in) {
this.name = name;
this.in = in;
this.mimeType = MimeTypeConstants.getMimeType(FilenameUtils.getExtension(name));
log.info("Document without mimetype. Considering mimetype '" + this.mimeType + "'.");
}
public Document(String name, InputStream in, String mimeType) {
this.name = name;
this.in = in;
this.mimeType = mimeType;
}
public String getName() {
return name;
}
public InputStream getIn() {
return in;
}
public String getMimeType() {
return mimeType;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy