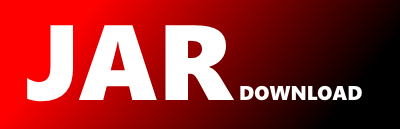
br.com.esec.icpm.libs.signature.helper.attach.CadesAttachSignatureHelper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of certillion-client-library Show documentation
Show all versions of certillion-client-library Show documentation
This library is used to make integration with Certillion server, so our Clients can easily ask for signatures or generate certificates.
package br.com.esec.icpm.libs.signature.helper.attach;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import org.apache.commons.io.IOUtils;
import org.bouncycastle.asn1.ASN1InputStream;
import org.bouncycastle.asn1.ASN1OutputStream;
import org.bouncycastle.asn1.BEROctetString;
import org.bouncycastle.asn1.cms.CMSObjectIdentifiers;
import org.bouncycastle.asn1.cms.ContentInfo;
import org.bouncycastle.asn1.cms.SignedData;
import org.bouncycastle.asn1.pkcs.PKCSObjectIdentifiers;
import org.bouncycastle.cms.CMSException;
import org.bouncycastle.cms.CMSProcessable;
import org.bouncycastle.util.io.Streams;
public class CadesAttachSignatureHelper {
public static void attach(long transactionId, InputStream originalDocument, InputStream signature, OutputStream signedDocument) {
ASN1InputStream signatureAsn1In = null;
try {
signatureAsn1In = new ASN1InputStream(signature);
ContentInfo contentInfo = ContentInfo.getInstance(signatureAsn1In.readObject());
SignedData sdDetached = SignedData.getInstance(contentInfo.getContent());
// TODO: Está carregando todo o documento para a memoria. Corrigir isto!!!
ContentInfo encapContentInfo = new ContentInfo(CMSObjectIdentifiers.data, new BEROctetString(IOUtils.toByteArray(originalDocument)));
SignedData sdAttached = new SignedData(
sdDetached.getDigestAlgorithms(),
encapContentInfo,
sdDetached.getCertificates(),
sdDetached.getCRLs(),
sdDetached.getSignerInfos());
ASN1OutputStream signedDocumentAsn1Out = new ASN1OutputStream(signedDocument);
ContentInfo contentInfoAttached = new ContentInfo(PKCSObjectIdentifiers.signedData, sdAttached);
signedDocumentAsn1Out.writeObject(contentInfoAttached);
signedDocumentAsn1Out.close();
} catch (Exception e) {
throw new IllegalStateException(e);
} finally {
IOUtils.closeQuietly(signatureAsn1In);
IOUtils.closeQuietly(originalDocument);
IOUtils.closeQuietly(signature);
IOUtils.closeQuietly(signedDocument);
}
}
static class CMSProcessableInputStream implements CMSProcessable {
private InputStream input;
private boolean used = false;
public CMSProcessableInputStream(InputStream input) {
this.input = input;
}
public InputStream getInputStream() {
checkSingleUsage();
return input;
}
public void write(OutputStream zOut) throws IOException, CMSException {
checkSingleUsage();
Streams.pipeAll(input, zOut);
input.close();
}
public Object getContent() {
return getInputStream();
}
private synchronized void checkSingleUsage() {
if (used) {
throw new IllegalStateException("CMSProcessableInputStream can only be used once");
}
used = true;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy