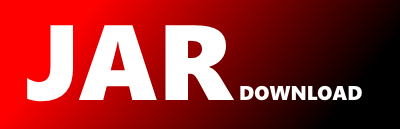
br.com.esec.icpm.libs.signature.helper.attach.XadesAttachSignatureHelper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of certillion-client-library Show documentation
Show all versions of certillion-client-library Show documentation
This library is used to make integration with Certillion server, so our Clients can easily ask for signatures or generate certificates.
package br.com.esec.icpm.libs.signature.helper.attach;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import javax.xml.parsers.ParserConfigurationException;
import javax.xml.transform.OutputKeys;
import javax.xml.transform.Transformer;
import javax.xml.transform.TransformerConfigurationException;
import javax.xml.transform.TransformerException;
import javax.xml.transform.TransformerFactory;
import javax.xml.transform.dom.DOMSource;
import javax.xml.transform.stream.StreamResult;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.w3c.dom.Document;
import org.w3c.dom.Node;
import org.xml.sax.SAXException;
public class XadesAttachSignatureHelper {
private static Logger log = LoggerFactory.getLogger(XadesAttachSignatureHelper.class);
private static DocumentBuilder docBuilder;
private static Transformer transformer;
static {
DocumentBuilderFactory docFactory = DocumentBuilderFactory.newInstance();
try {
docBuilder = docFactory.newDocumentBuilder();
} catch (ParserConfigurationException e) {
throw new IllegalStateException("Can not mount docBuilder.", e);
}
TransformerFactory transformerFactory = TransformerFactory.newInstance();
try {
transformer = transformerFactory.newTransformer();
transformer.setOutputProperty(OutputKeys.OMIT_XML_DECLARATION, "yes");
} catch (TransformerConfigurationException e) {
throw new IllegalStateException("Can not mount transformer.", e);
}
}
public static void attach(long transactionId, InputStream originalXml, InputStream signature, OutputStream signedXml) {
try {
log.info("[TRANSACTION " + transactionId + "] " + "Attaching signature on XML... ");
Document originalDoc = docBuilder.parse(originalXml);
Document signatureTagDoc = docBuilder.parse(signature);
// faz o attach de verdade
Node child = originalDoc.getFirstChild();
Node newTag = signatureTagDoc.getFirstChild().cloneNode(true);
originalDoc.adoptNode(newTag);
child.appendChild(newTag);
encodeXml(originalDoc, signedXml);
log.info("[TRANSACTION " + transactionId + "] " + "XML attached.");
} catch (IOException e) {
throw new IllegalStateException(e);
} catch (SAXException e) {
throw new IllegalStateException(e);
} catch (TransformerException e) {
throw new IllegalStateException(e);
}
}
private static void encodeXml(Node rootTag, OutputStream out) throws TransformerException {
DOMSource source = new DOMSource(rootTag);
StreamResult result = new StreamResult(out);
transformer.transform(source, result);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy