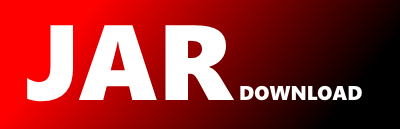
br.com.esec.icpm.libs.signature.response.polling.SimplePollingService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of certillion-client-library Show documentation
Show all versions of certillion-client-library Show documentation
This library is used to make integration with Certillion server, so our Clients can easily ask for signatures or generate certificates.
package br.com.esec.icpm.libs.signature.response.polling;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.google.common.util.concurrent.ListenableFuture;
import com.google.common.util.concurrent.SettableFuture;
import br.com.esec.icpm.libs.Server;
import br.com.esec.icpm.libs.signature.helper.RequestBatchSignatureHelper;
import br.com.esec.icpm.libs.signature.helper.RequestStatusHelper;
import br.com.esec.icpm.libs.signature.response.Futures;
import br.com.esec.icpm.mss.ws.SignatureStatusRespType;
import br.com.esec.icpm.server.factory.Status;
import br.com.esec.icpm.server.ws.ICPMException;
public class SimplePollingService extends BasePollingService {
private static Logger log = LoggerFactory.getLogger(RequestBatchSignatureHelper.class);
private static SimplePollingService instance;
public static SimplePollingService getInstance() {
if (instance == null)
instance = new SimplePollingService();
return instance;
}
public ListenableFuture status(Server server, long transactionId) {
if (Futures.containsKey(server, transactionId)) {
return Futures.get(server, transactionId);
}
SettableFuture future = SettableFuture.create();
Futures.put(server, transactionId, future);
final Checker checker = new Checker(server, transactionId);
schedule(checker);
return future;
}
private class Checker extends BaseChecker {
public Checker(Server server, long transactionId) {
super(server, transactionId);
}
@Override
protected void check(SettableFuture future) {
try {
SignatureStatusRespType signatureStatusResp = RequestStatusHelper.requestStatus(server, transactionId);
log.info("[TRANSACTION ID " + transactionId + "] Checking simple transaction ...");
if (signatureStatusResp.getStatus().getStatusCode() == Status.TRANSACTION_IN_PROGRESS.getCode()) {
schedule(this, counter); // schedule to run again in 2 seconds
log.info("[TRANSACTION ID " + transactionId + "] In progess yet. A next check was scheduled.");
} else {
future.set(signatureStatusResp);
}
} catch (ICPMException e) {
future.setException(e);
}
}
}
private SimplePollingService() {
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy