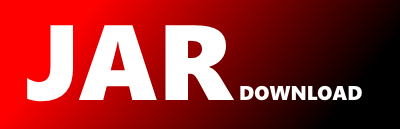
com.esec.signature.MakeExternalSignature Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of esec-itext Show documentation
Show all versions of esec-itext Show documentation
This library is used to sign PDFs.
The newest version!
package com.esec.signature;
import java.io.ByteArrayInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.security.GeneralSecurityException;
import java.security.NoSuchAlgorithmException;
import java.security.cert.Certificate;
import java.util.Calendar;
import java.util.HashMap;
import com.esec.text.DocumentException;
import com.esec.text.pdf.PdfDate;
import com.esec.text.pdf.PdfDictionary;
import com.esec.text.pdf.PdfName;
import com.esec.text.pdf.PdfPKCS7;
import com.esec.text.pdf.PdfSignature;
import com.esec.text.pdf.PdfSignatureAppearance;
import com.esec.text.pdf.PdfString;
/**
* Class that signs your PDF.
*
*/
public class MakeExternalSignature {
private static final int ESTIMATED_SIZE = 8192;
public static InputStream getData(PdfSignatureAppearance sap, Certificate signerCert) throws IOException, DocumentException, GeneralSecurityException {
sap.setCertificate(signerCert);
PdfSignature dic = new PdfSignature(PdfName.ADOBE_PPKLITE, PdfName.ADBE_PKCS7_DETACHED);
dic.setReason(sap.getReason());
dic.setLocation(sap.getLocation());
dic.setContact(sap.getContact());
dic.setDate(new PdfDate(sap.getSignDate())); // time-stamp will over-rule this
sap.setCryptoDictionary(dic);
HashMap exc = new HashMap();
exc.put(PdfName.CONTENTS, new Integer(ESTIMATED_SIZE * 2 + 2));
sap.preCloseWithExternalSignature(exc);
return sap.getRangeStream();
}
public static void attachSignature(PdfSignatureAppearance sap, Certificate signerCert, byte[] encodedSig) throws IOException, DocumentException, GeneralSecurityException {
sap.setCertificate(signerCert);
PdfSignature dic = new PdfSignature(PdfName.ADOBE_PPKLITE, PdfName.ADBE_PKCS7_DETACHED);
dic.setReason(sap.getReason());
dic.setLocation(sap.getLocation());
dic.setContact(sap.getContact());
dic.setDate(new PdfDate(sap.getSignDate())); // time-stamp will over-rule this
sap.setCryptoDictionary(dic);
HashMap exc = new HashMap();
exc.put(PdfName.CONTENTS, new Integer(ESTIMATED_SIZE * 2 + 2));
sap.preCloseWithExternalSignature(exc);
// InputStream data = sap.getRangeStream();
if (ESTIMATED_SIZE + 2 < encodedSig.length)
throw new IOException("Not enough space");
byte[] paddedSig = new byte[ESTIMATED_SIZE];
System.arraycopy(encodedSig, 0, paddedSig, 0, encodedSig.length);
PdfDictionary dic2 = new PdfDictionary();
dic2.put(PdfName.CONTENTS, new PdfString(paddedSig).setHexWriting(true));
sap.close(dic2);
}
/**
* Signs the document using the detached mode, CMS or CAdES equivalent.
*
* @param sap the PdfSignatureAppearance
* @param externalDigest the interface providing the actual signing
* @param externalSignature
* @param chain the certificate chain
* @throws IOException
* @throws DocumentException
* @throws GeneralSecurityException
*/
public static void signDetached(PdfSignatureAppearance sap, ExternalDigest externalDigest, ExternalSignature externalSignature, Certificate[] chain) throws IOException, DocumentException, GeneralSecurityException {
sap.setCertificate(chain[0]);
PdfSignature dic = new PdfSignature(PdfName.ADOBE_PPKLITE, PdfName.ADBE_PKCS7_DETACHED);
dic.setReason(sap.getReason());
dic.setLocation(sap.getLocation());
dic.setContact(sap.getContact());
dic.setDate(new PdfDate(sap.getSignDate())); // time-stamp will over-rule this
sap.setCryptoDictionary(dic);
HashMap exc = new HashMap();
exc.put(PdfName.CONTENTS, new Integer(ESTIMATED_SIZE * 2 + 2));
sap.preCloseWithExternalSignature(exc);
String hashAlgorithm = externalSignature.getHashAlgorithm();
PdfPKCS7 sgn = new PdfPKCS7(null, chain, null, hashAlgorithm, null, false);
InputStream data = sap.getRangeStream();
byte hash[] = DigestAlgorithms.digest(data, externalDigest.getMessageDigest(hashAlgorithm));
Calendar cal = Calendar.getInstance();
byte[] sh = sgn.getAuthenticatedAttributeBytes(hash, cal, null);
byte[] extSignature = externalSignature.sign(new ByteArrayInputStream(sh));
sgn.setExternalDigest(extSignature, null, externalSignature.getEncryptionAlgorithm());
byte[] encodedSig = sgn.getEncodedPKCS7(hash, cal);
if (ESTIMATED_SIZE + 2 < encodedSig.length)
throw new IOException("Not enough space");
byte[] paddedSig = new byte[ESTIMATED_SIZE];
System.arraycopy(encodedSig, 0, paddedSig, 0, encodedSig.length);
PdfDictionary dic2 = new PdfDictionary();
dic2.put(PdfName.CONTENTS, new PdfString(paddedSig).setHexWriting(true));
sap.close(dic2);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy