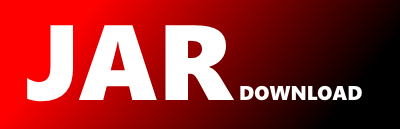
br.com.esec.icpm.signer.ws.rest.PemUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of security-helper Show documentation
Show all versions of security-helper Show documentation
This library is used to help with HTTP with SSL auth client and WS-Secutiry.
The newest version!
package br.com.esec.icpm.signer.ws.rest;
import java.io.BufferedReader;
import java.io.ByteArrayInputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import org.apache.commons.codec.binary.Base64;
import org.apache.commons.io.IOUtils;
public class PemUtils {
public static boolean isPem(File file) throws IOException {
FileInputStream in = null;
BufferedReader br = null;
try {
in = new FileInputStream(file);
br = new BufferedReader(new InputStreamReader(in));
String line = br.readLine();
if (line.startsWith("--")) {
return true;
}
return false;
} finally {
IOUtils.closeQuietly(in);
IOUtils.closeQuietly(br);
}
}
/**
* Reads and decodes a PEM certificate into a DER certificate.
*
* @param certificateFile file to convert to DER
* @return An InputStream containing the decoded certificate.
* @throws IOException
* if the input stream is closed or some other I/O error occurs.
*/
public static InputStream convertPemToDer(File certificateFile) throws IOException {
BufferedReader br = null;
InputStream certificateStream = null;
try {
certificateStream = new FileInputStream(certificateFile);
StringBuilder buf = new StringBuilder();
br = new BufferedReader(new InputStreamReader(certificateStream));
String line = br.readLine();
while (line != null) {
if (!line.startsWith("--")) {
buf.append(line);
}
line = br.readLine();
}
String pem = buf.toString();
return new ByteArrayInputStream(Base64.decodeBase64(pem));
} finally {
IOUtils.closeQuietly(br);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy