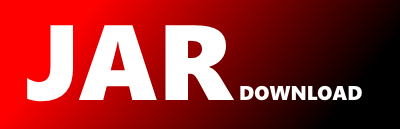
br.com.esec.icpm.signer.ws.rest.SslUtils Maven / Gradle / Ivy
package br.com.esec.icpm.signer.ws.rest;
import java.io.IOException;
import java.net.MalformedURLException;
import java.net.URL;
import java.nio.charset.Charset;
import java.security.KeyManagementException;
import java.security.KeyStore;
import java.security.KeyStoreException;
import java.security.NoSuchAlgorithmException;
import java.security.UnrecoverableKeyException;
import java.security.cert.CertificateException;
import java.security.cert.X509Certificate;
import javax.net.ssl.SSLContext;
import javax.net.ssl.TrustManager;
import javax.net.ssl.TrustManagerFactory;
import javax.net.ssl.X509TrustManager;
import javax.ws.rs.client.ClientBuilder;
import org.apache.commons.io.IOUtils;
import org.apache.http.conn.ssl.SSLConnectionSocketFactory;
import org.apache.http.conn.ssl.SSLContextBuilder;
import org.apache.http.conn.ssl.X509HostnameVerifier;
import br.com.esec.icpm.signer.security.SecurityConfig;
public class SslUtils {
public static void config(final ClientBuilder builder) {
SSLContext sslContext = buildSslContext();
builder.sslContext(sslContext);
builder.hostnameVerifier(getHostNameVerifier());
}
public static SSLContext buildSslContext() {
try {
// SSL Context
SSLContextBuilder sslContextBuilder = new SSLContextBuilder();
if (SecurityConfig.getKeystore() != null)
sslContextBuilder.loadKeyMaterial(SecurityConfig.getKeystore(), SecurityConfig.getKeystorePassword().toCharArray()); // load the key material
sslContextBuilder.loadTrustMaterial(null); // Load java truststore
if (SecurityConfig.getTruststore() != null)
sslContextBuilder.loadTrustMaterial(SecurityConfig.getTruststore()); // Load any other material
return sslContextBuilder.build();
} catch (UnrecoverableKeyException e) {
throw new IllegalStateException(e);
} catch (NoSuchAlgorithmException e) {
throw new IllegalStateException(e);
} catch (KeyStoreException e) {
throw new IllegalStateException(e);
} catch (CertificateException e) {
throw new IllegalStateException(e);
} catch (IOException e) {
throw new IllegalStateException(e);
} catch (KeyManagementException e) {
throw new IllegalStateException(e);
}
}
public static X509HostnameVerifier getHostNameVerifier() {
return SecurityConfig.isAllowAllHosts() ? SSLConnectionSocketFactory.ALLOW_ALL_HOSTNAME_VERIFIER : SSLConnectionSocketFactory.BROWSER_COMPATIBLE_HOSTNAME_VERIFIER;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy