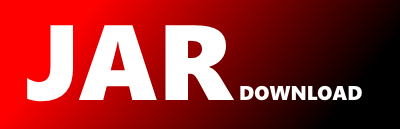
br.com.esec.icpm.util.FileUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of security-helper Show documentation
Show all versions of security-helper Show documentation
This library is used to help with HTTP with SSL auth client and WS-Secutiry.
The newest version!
package br.com.esec.icpm.util;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.net.URL;
import org.apache.commons.io.IOUtils;
@Deprecated
public class FileUtils {
public static byte[] loadFile(String path) {
return loadFile(path, null);
}
public static byte[] loadFile(String path, Class> clazz) {
InputStream in = null;
try {
in = loadFileStream(path, clazz);
if (in != null)
return IOUtils.toByteArray(in);
} catch (IOException e) {
// do nothing
} finally {
IOUtils.closeQuietly(in);
}
throw new IllegalStateException("File " + path + " not found");
}
public static InputStream loadFileStream(String path) {
return loadFileStream(path, null);
}
public static InputStream loadFileStream(String path, Class> clazz) {
final File file = getFile(path, clazz);
if (file == null)
throw new IllegalStateException("File " + path + " not found");
try {
return new FileInputStream(file);
} catch (FileNotFoundException e) {
throw new IllegalStateException("File " + path + " not found", e);
}
}
public static File getFile(String path) {
return getFile(path, null);
}
public static File getFile(String path, Class> clazz) {
try {
File file = new File(path);
if (file.exists()) {
return file;
}
} catch (Exception e) {
// do nothing
}
try {
File file = new File(new URL(path).toURI());
if (file.exists()) {
return file;
}
} catch (Exception e) {
// do nothing
}
try {
// attempt to load from the context classpath
ClassLoader loader = Thread.currentThread().getContextClassLoader();
URL url = loader.getResource("/" + path);
if (url != null) {
final File file = new File(url.toURI());
if (file.exists()) {
return file;
}
}
} catch (Exception e) {
// do nothing
}
try {
// attempt to load from the context classpath
ClassLoader loader = Thread.currentThread().getContextClassLoader();
URL url = loader.getResource(path);
if (url != null) {
final File file = new File(url.toURI());
if (file.exists()) {
return file;
}
}
} catch (Exception e) {
// do nothing
}
try {
// attempt to load from the context classpath
ClassLoader loader = Thread.currentThread().getContextClassLoader();
InputStream in = loader.getResourceAsStream("/" + path);
if (in != null) {
File tmp = File.createTempFile("security-helper-", ".data");
tmp.deleteOnExit();
IOUtils.copy(in, new FileOutputStream(tmp));
return tmp;
}
} catch (Exception e) {
// do nothing
}
try {
// attempt to load from the context classpath
ClassLoader loader = Thread.currentThread().getContextClassLoader();
InputStream in = loader.getResourceAsStream(path);
if (in != null) {
File tmp = File.createTempFile("security-helper-", ".data");
tmp.deleteOnExit();
IOUtils.copy(in, new FileOutputStream(tmp));
return tmp;
}
} catch (Exception e) {
// do nothing
}
try {
URL url = FileUtils.class.getResource("/" + path);
if (url != null) {
final File file = new File(url.toURI());
if (file.exists()) {
return file;
}
}
url = FileUtils.class.getResource(path);
if (url != null) {
final File file = new File(url.toURI());
if (file.exists()) {
return file;
}
}
} catch (Exception e) {
// do nothing
}
try {
if (clazz != null) {
URL url = clazz.getResource("/" + path);
if (url != null) {
final File file = new File(url.toURI());
if (file.exists()) {
return file;
}
}
url = clazz.getResource(path);
if (url != null) {
final File file = new File(url.toURI());
if (file.exists()) {
return file;
}
}
}
} catch (Exception e) {
// do nothing
}
throw new IllegalStateException("File " + path + " not found");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy